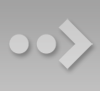
192 CHAPTER 8RECURSION
Similarly, the Collatz problem is fascinating (because it is so simple and yet so
little progress has been made in answering the question of whether it always ter-
minates with a 1), but this problem is really a problem in pure mathematics. The
Ackermann function similarly, although it has been useful in theoretical computer
science, is not something that has found its way into practical issues central to
computation in the real world.
In contrast, a computation that does come up in the real world, and that can
and should be defined recursively if possible, is the generation of the permutations
or combinations of a list of items. A rank-ordering of preferences of N items, for
example, can occur in N! ways because any of the possible rank orderings could
occur. It frequently happens in specifying requirements for a computation that one
must generate permutations and combinations of options if only in order to test
that one’s software works for all possible sets of input values.
One puzzle-level problem that when attacked naively is a permutation problem
is the Jumble puzzle from the newspaper, in which readers are given anagrams of
five- and six-letter words and asked to come up with the English words made up
of those letters. Given the letters “celos,” for example, one would be expected to
come up with the word “close.”
A naive method for solving the Jumble puzzle is to generate all the permutations
of the list of letters, that is, all the five-letter (or six-letter) rearrangements of
the given letters used without replacement. These putative words could then be
looked up (using binary search of course) in a dictionary of English words. The
naive solution to the Jumble puzzle is to treat the letters as a mathematical set
of N letters. We start with a “word” word initialized to the null string and then
call a permutation-generation method, passing in the set of letters and the current
value of word.
The method runs a loop over all N possible starting letters. In each iteration of
this loop we pick one letter from the set, assign that letter as the next letter in the
word by concatenating the letter onto the current value of word, remove the chosen
letter from the set, and then recursively call the method, passing in as parameters
the new set (one smaller than before) and the new partially constructed word,one
letter longer than before. The base case occurs when there are no more letters in
the set, at which point we return word as constructed.
The recursive process for generating the permutations is shown in Figure 8.2,
where we generate all possible permutations from the list of three letters {e, h, t}.In
the first step, we choose a first letter, e,addthee to the partial permutation we have
created, remove e from the list of unused letters, and call the method recursively.
The method chooses a letter (the second letter), choosing the first entry in the list,
h, adding it to the partial permutation, deleting the h from the list of unused letters,
and calling the method recursively. The third letter is now chosen, and we have the
three-letter word made up of the three letters in their original sequence order 123.