The following code sample is a RADOS class which when executed reads the object, calculates the MD5 hash, and then writes it as an attribute to the object without any client involvement. Each time this class is called, it repeats this operation a 1000 times locally to OSD and only notifies the client at the end of this processing. We have the following steps to perform:
- Create the directory for our new RADOS class:
mkdir ~/ceph/src/cls/md5
- Now create the C++ source file:
~/ceph/src/cls/md5/cls_md5.cc
- Place the following code into it:
#include "objclass/objclass.h"
#include <openssl/md5.h>
CLS_VER(1,0)
CLS_NAME(md5)
cls_handle_t h_class;
cls_method_handle_t h_calc_md5;
static int calc_md5(cls_method_context_t hctx, bufferlist *in,
bufferlist *out)
{
char md5string[33];
for(int i = 0; i < 1000; ++i)
{
size_t size;
int ret = cls_cxx_stat(hctx, &size, NULL);
if (ret < 0)
return ret;
bufferlist data;
ret = cls_cxx_read(hctx, 0, size, &data);
if (ret < 0)
return ret;
unsigned char md5out[16];
MD5((unsigned char*)data.c_str(), data.length(), md5out);
for(int i = 0; i < 16; ++i)
sprintf(&md5string[i*2], "%02x", (unsigned int)md5out[i]);
CLS_LOG(0,"Loop:%d - %s",i,md5string);
bufferlist attrbl;
attrbl.append(md5string);
ret = cls_cxx_setxattr(hctx, "MD5", &attrbl);
if (ret < 0)
{
CLS_LOG(0, "Error setting attribute");
return ret;
}
}
out->append((const char*)md5string, sizeof(md5string));
return 0;
}
void __cls_init()
{
CLS_LOG(0, "loading cls_md5");
cls_register("md5", &h_class);
cls_register_cxx_method(h_class, "calc_md5", CLS_METHOD_RD |
CLS_METHOD_WR, calc_md5, &h_calc_md5)
}
- Change into the build directory created previously and create our new RADOS class using make:
cd ~/ceph/build
make cls_md5
The preceding commands will give the following output:

- We now need to copy our new class to OSDs in our cluster:
sudo scp vagrant@ansible:/home/vagrant/ceph/build/lib/libcls_md5.so*
/usr/lib/rados-classes/
The preceding command will give the following output:

Also, restart the OSD for it to load the class.
You will now see in the Ceph OSD log that it is loading our new class:
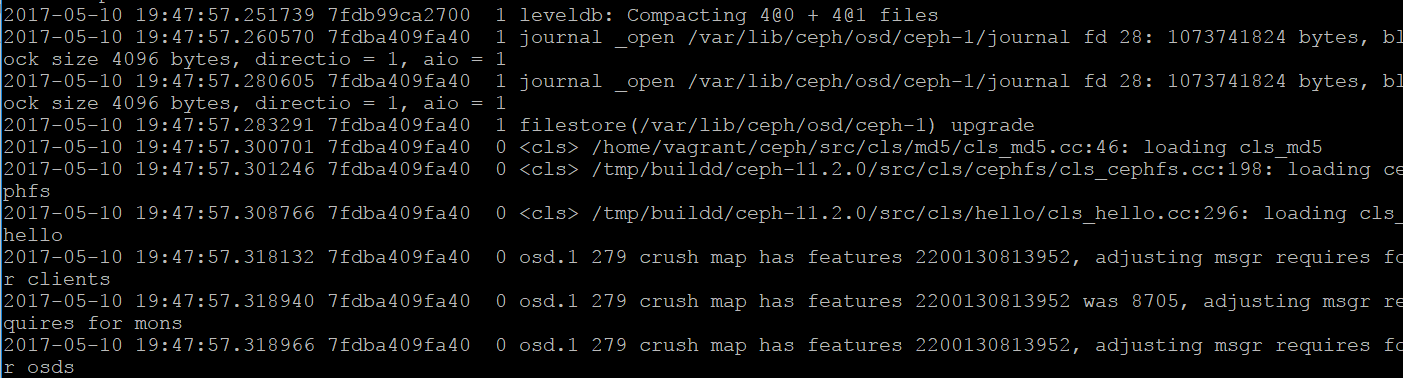
This needs to be repeated for all OSD nodes in the cluster.