A Task is basically a piece of executable code that is dynamically created and managed by TEA. You can use dependency injection (DI) to obtain objects in the DI context of the Task (or any parent context—see Figure 3-4). You can think of it as a more powerful Runnable.
A Very Simple Task
Something worth noting here is that the sample Task does not implement an interface. The only requirement for a Task is that it has a single method annotated with @Execute. This method can use DI. Every Task has its own dependency injection context. This is important, for instance, when injecting a TaskProgressTracker , which we will cover in detail in Chapter 8.
Naming
Apart from the required @Execute method , you can influence how TEA handles and presents a Task (in logs, UI, etc.).
Providing a proper name for a Task is the most basic thing you need to do. By default, TEA will use the simple class name of a Task to show in logs and the Tasking Live View (If you revisit any of the samples from Chapter 4, you will see this because we have not named a Task until now. For instance, see Figure 4-7.)
Naming a Task with the @Named annotation
This Task will be shown as "Named Task" instead of TaskStaticNamed whenever TEA needs to display a Task name—in the log as well as in the Tasking Live View.
Naming a Task with the toString Method

@Named and toString in Action
Hint
Run the TEA-Book-Samples launch configuration and find the TEA ➤ Samples ➤ CH05-S01: Naming entry in the TEA menu.
Incidentally, the same naming logic is also used for TaskChain implementations.
Return Values
It is not normally possible to return any values from a Task . Since the framework is always the caller for each Task (as we will describe in Chapter 6, Tasks are passed to the framework, and the framework is responsible for executing them at the right point in time), it would not make that much sense in the first place.
Returning Information from a Task
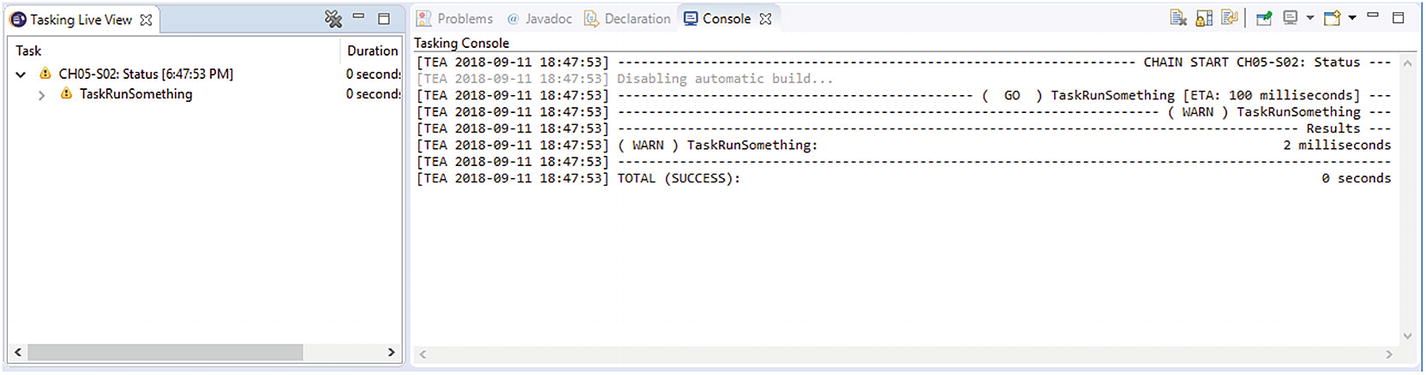
Returning an IStatus to the TEA framework
Output Capturing
Sometimes a Task needs to call code that is not aware of TEA at all; it could be any preexisting code. In this case, the code may do a plethora of different things to log things, including writing to System.out and System.err. This will work just fine in headless environments because TEA logs to the same place in these cases. However, when running a Task in the IDE, this is suboptimal. Output is partially in the Tasking Console, and the System.out/System.err part goes to the output streams of the IDE itself. That’s definitely what we don’t want.
Capturing Output of Third-party Code