Controllers are responsible for the workflow of the application. They retrieve user requests, work with the model, and then choose the view to render.
Controllers in ASP.NET Core MVC are C# classes that reside under the Controllers directory and inherit from Microsoft.AspNetCore.Mvc.Controller. Controller classes must match one of the following rules as well:
- The class name ends with Controller, for example, UsersController
- The class inherits from a class whose name ends with Controller
- The class is decorated with the [Controller] or [ApiController] attribute
Public methods inside controller classes are also called actions. Each action handles a different request from end users and generates output. Usually, a controller class gathers multiple actions that relate to the same logical operation, such as login, or application entities such as users.
In SPAs, from the server's point of view, there is only a single page in the application. Therefore, for SPAs, we do not need more than a single controller with a single action that serves the main application page and lets the frontend code take charge.
To create the controller, follow these steps:
- Right-click on the application name in Solution Explorer and choose Add | New Folder:

- Set the folder name to Controllers.
- Right-click on the newly created Controllers folder and choose Add | Controller….
- If the Add Dependencies dialog pops up, choose Minimal Dependencies and click Add:

You will have to perform step 3 once more after dependencies have been installed successfully.
- In the list of available templates, choose MVC Controller – Empty:
- Set the name as HomeController and click Add.
The controller is now ready and should look similar to the following:
namespace GiveNTake.Controllers
{
public class HomeController : Controller
{
public IActionResult Index()
{
return View();
}
}
}
This controller has a single action method called Index. This is the naming convention for the default action of a controller. For example, if a controller is responsible for operations related to a User entity, then the Index action will generate an output with the list of users in the system.
The return View(); line tells ASP.NET Core MVC to generate a view using the default MVC naming convention. These will be discussed in the next section, Views.
For now, change the return View(); line to return Content(“Hello from MVC!”);. This will tell MVC to return a simple Hello from MVC! string to the end user. The Index method should look similar to the following after the change:
public IActionResult Index()
{
return Content("Hello from MVC!");
}
ASP.NET MVC is ready to run — follow these steps to build and run the application:
- Click on Build | Build Solution, or use the keyboard shortcut Ctrl + Shift + B:

- Once the build ends successfully, click on the Play button on the toolbar, or click F5:

- The default browser should open and look like the following screenshot:
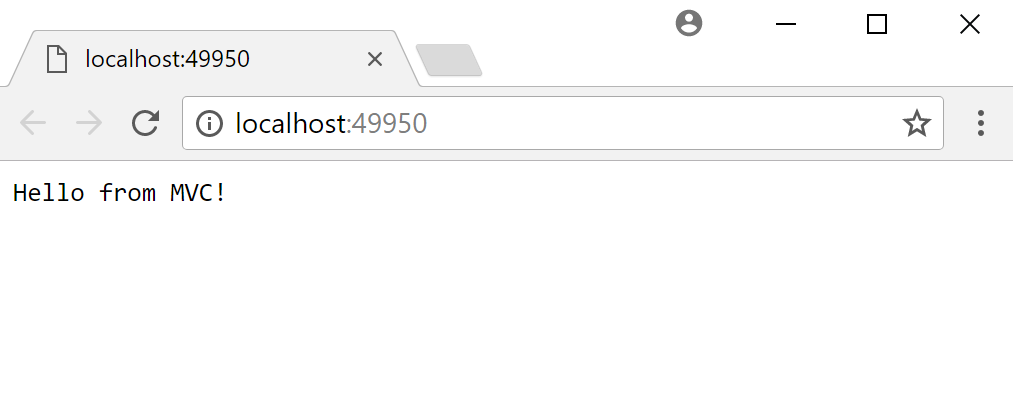
Now that we have an operating ASP.NET Core MVC application, we can continue and fit it to our needs.