In the previous example, we tested the method with only one value. Practically, when testing an enterprise application, we want to test it with different values to estimate the method's performance.
First of all, we can define a property for each parameter, set the Params attribute, and specify the value(s) for which we need that method to be tested. Then we can use that property in the code. BenchmarkRun automatically tests that method with all of the parameters and generates the report. Here is the complete code snippet of the TestBenchmark class:
public class TestBenchmark { [Params(10,20,30)] public int Len { get; set; } [Benchmark] public void Fibonacci() { int a = 0, b = 1, c = 0; Console.Write("{0} {1}", a, b); for (int i = 2; i < Len; i++) { c = a + b; Console.Write(" {0}", c); a = b; b = c; } } [Benchmark] public void FibonacciRecursive() { Fibonacci_Recursive(0, 1, 1, Len); } private void Fibonacci_Recursive(int a, int b, int counter, int len) { if (counter <= len) { Console.Write("{0} ", a); Fibonacci_Recursive(b, a + b, counter + 1, len); } } }
After running Benchmark, the following report is generated:
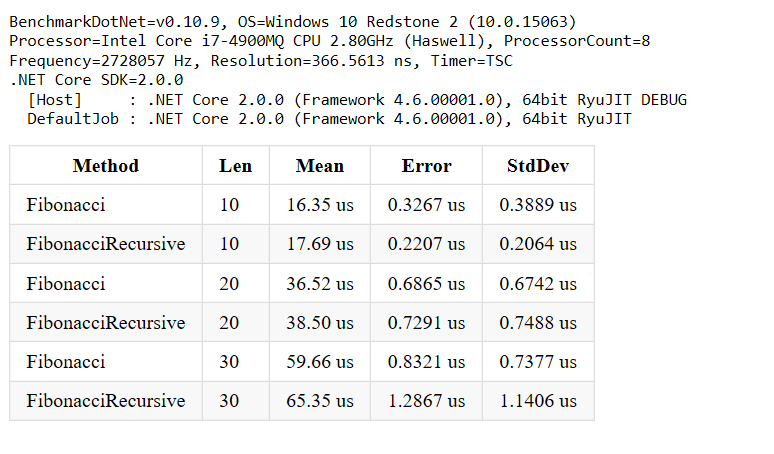