Coming from the relational DB world, we identify objects by their relationships. A one-to-one relationship could be a person with an address. Modeling it in a relational database would most probably require two tables: a person and an address table with a foreign key person_id in the address table.
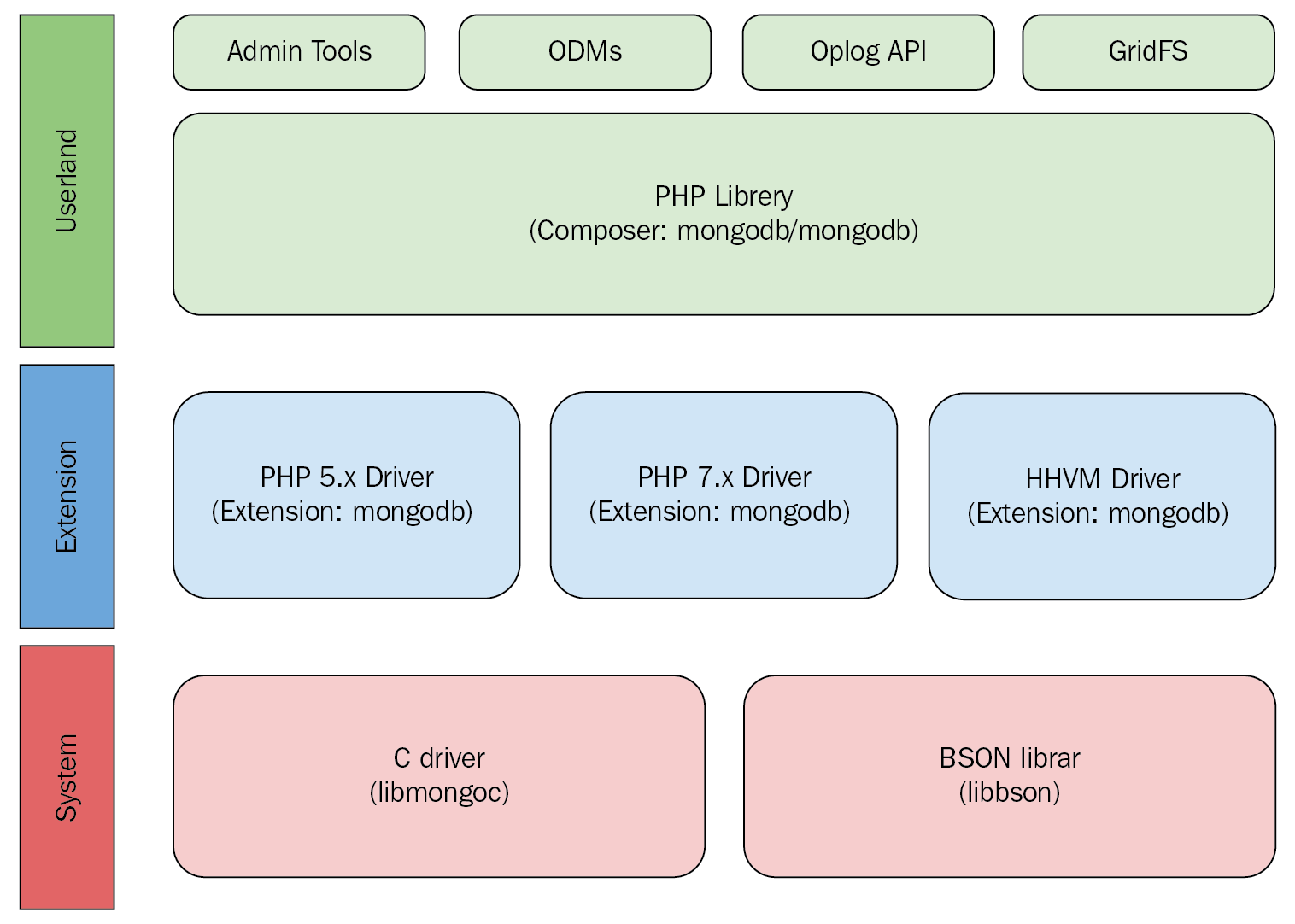
The perfect analogy in MongoDB would be two collections, person and address, looking like this:
> db.Person.findOne()
{
"_id" : ObjectId("590a530e3e37d79acac26a41"), "name" : "alex"
}
> db.Address.findOne()
{
"_id" : ObjectId("590a537f3e37d79acac26a42"),
"person_id" : ObjectId("590a530e3e37d79acac26a41"),
"address" : "N29DD"
}
Now we can use the same pattern as we do in a relational database to find a person from an address:
> db.Person.find({"_id": db.Address.findOne({"address":"N29DD"}).person_id})
{
"_id" : ObjectId("590a530e3e37d79acac26a41"), "name" : "alex"
}
This pattern is well known and works in the relational world.
In MongoDB, we don't have to follow this pattern and there are more suitable ways to model these kinds of relationship.
A way in which we would typically model a one-one or one-few relationship in MongoDB would be through embedding. The same example would then become if the person has two addresses:
{ "_id" : ObjectId("590a55863e37d79acac26a43"), "name" : "alex", "address" : [ "N29DD", "SW1E5ND" ] }
Using an embedded array we can have access to every address this user has. Embedding querying is rich and flexible so that we can store more information in each document:
{ "_id" : ObjectId("590a56743e37d79acac26a44"),
"name" : "alex",
"address" : [ { "description" : "home", "postcode" : "N29DD" },
{ "description" : "work", "postcode" : "SW1E5ND" } ] }
Advantages of this approach:
- No need for two queries across different collections
- Can exploit atomic updates to make sure that updates in the document will be all-or-nothing from the perspective of other readers of this document
- Can embed attributes in multiple nest levels creating complex structures
The most notable disadvantage is that the document maximum size is 16 MB so this approach cannot be used for an arbitrary, ever growing number of attributes. Storing hundreds of elements in embedded arrays will also degrade performance.