In the last chapter, we learned how to use the Raspberry Pi cluster we built for parallel programming with MPI4PY and Python 3. In this chapter, we will be introduced to the SciPy stack and install it on the Pi. We will also get started with symbolic programming with SymPy.
The Scientific Python Stack
SciPy (short for Scientific Python) is an open-source library for scientific and technical computing in Python.
SciPy has modules for numerical operations, ordinary differential equation solvers, fast Fourier transforms, optimization, linear algebra, integration, interpolation, signal processing, and image processing. SciPy is extensively used by scientific, mathematical, and engineering communities around the world. There are many other libraries which use core modules of SciPy and NumPy for operations. OpenCV and SciKit are the best examples of other major libraries using NumPy and/or SciPy.
The SciPy stack has the following components :
NumPy is a library for numerical computations. It provides all the basic data types required for numeric and scientific computing .
SciPy Library has many modules for scientific programming.
Matplotlib is used for data visualization.
SymPy is used for Symbolic Programming.
IPython is an advanced Python interpreter with added features.
Pandas is used for data analysis.
Nose is used for automating test cases.
The following figure (Figure 9-1) summarizes the role of the Python SciPy stack in the world of scientific computing.
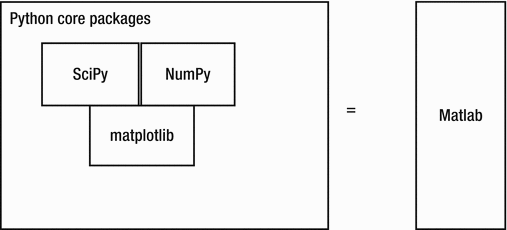
Figure 9-1. The components of the SciPy stack
In this book, we will study NymPy, SciPy Library, Matplotlib, and SymPy.
Installation of the SciPy Stack
The best way to install the SciPy stack on Raspberry Pi is to use apt-get and pip.
First, upgrade pip with the following command:
sudo python3 -m pip install --upgrade pip
Install SymPy with the following command:
sudo pip3 install sympy
The rest of the components of the SciPy stack can conveniently be installed with the apt-get utility as follows:
sudo apt-get install python3-matplotlib -y
sudo apt-get install python3-scipy -y
sudo apt-get install python3-numpy -y
This installs the required components of the SciPy stack.
SymPy
The SymPy website says:
SymPy is a Python library for symbolic mathematics. It aims to become a full-featured computer algebra system (CAS) while keeping the code as simple as possible in order to be comprehensible and easily extensible. SymPy is written entirely in Python.
SymPy is licensed under BSD and free. It has been written entirely in Python. It depends on mpmath and is lightweight in nature as there are no other dependencies.
Getting Started
Let’s get started with SymPy. Run the following commands to create and navigate to the directory for the chapter:
cd ∼
cd book
cd code
mkdir chapter09
cd chapter09
We will keep the code for this chapter in the directory chapter09 and will continue this practice for the rest of the book, i.e. creating directories by chapter for organizing the code.
I assume that you have some basic knowledge of mathematics and calculus, so I do not have to explain the basics when explaining the code. Let’s see a simple example (Listing 9-1) of the concept of symbolic computation.
Listing 9-1. prog01.py
import math
import sympy
print(math.sqrt(9))
print(math.sqrt(8))
print(sympy.sqrt(9))
print(sympy.sqrt(8))
Run the code above (Listing 9-1). The following is the output:
3.0
2.8284271247461903
3
2*sqrt(2)
In the output above, we can see that the math.sqrt() method directly produces the results in numeric format, whereas the sympy.sqrt() method produces the result in numeric format only in the case where it is an integer. Rather than producing a fractional value, it keeps sqrt(2) as it is. This way, we can symbolically compute a lot of mathematical equations. Equipped with this knowledge of the concept of symbolic mathematics, let’s go deeper into SymPy with Python 3.
Symbols
Let’s study the concept of symbols . Symbols are analogous to variables in mathematics. We can use them for evaluations in equations and expressions. They can also be used to solve equations. The sympy.symbols() method converts a string into symbolic variables as follows (Listing 9-2):
Listing 9-2. prog02.py
from sympy import *
x = symbols('x')
sigma, y = symbols('sigma y')
print(x, y, sigma)
The output is as follows:
x y sigma
The above code (Listing 9-2) demonstrates that the symbols() method can accept a string where the tokens are separated by whitespace as an argument.
Let’s see one more example (Listing 9-3) which demonstrates the evaluation of an expression with symbols.
Listing 9-3. prog03.py
from sympy import *
x = symbols('x')
expr = x + 1
print(expr.subs(x, 3))
This will substitute 3 in place of x in the expression and evaluate that. The code (Listing 9-3) produces 4 as output.
We can substitute for multiple symbols as follows (Listing 9-4):
Listing 9-4. prog04.py
from sympy import *
x, y = symbols('x y')
expr = x + y
print(expr.subs({x:3, y:2}))
Here, we are substituting for multiple symbols at once. Run the code and check the output.
Converting Strings to SymPy Expressions
We can convert strings to SymPy expressions. Just as in Python, we can use the ** operator for the exponent. The following code (Listing 9-5) shows this:
Listing 9-5. prog05.py
from sympy import *
str = "x**3 + 4*y - 1/5"
expr = sympify(str)
print(expr)
The sympify() method converts a string to a SymPy expression.
We can also use the evalf() method for evaluating expressions to a floating point number. It has a default precision of 15 digits after the decimal; however, we can pass the precision as an argument. The following (Listing 9-6) shows the example use cases of the evalf() method:
Listing 9-6. prog06.py
from sympy import *
expr = sqrt(10)
print(expr.evalf())
print(pi.evalf(20))
x = symbols('x')
expr = sin(2*x)
print(expr.evalf(subs={x: 2.4}))
The output is as follows,
3.16227766016838
3.1415926535897932385
-0.996164608835841
Printing in SymPy
Sympy’s Printing Functionality
SymPy has many printers . In any environment, use of init_session() method at the command prompt will start an interactive session. The following is an example of a sample interactive session. The commands I typed into the console are highlighted in bold.
pi@pi001:∼/book/code/chapter09 $ python3
Python 3.4.2 (default, Oct 19 2014, 13:31:11)
[GCC 4.9.1] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> from sympy import *
>>> init_session()
Python console for SymPy 1.0 (Python 3.4.2-32-bit) (ground types: python)
These commands were executed:
>>> from __future__ import division
>>> from sympy import *
>>> x, y, z, t = symbols('x y z t')
>>> k, m, n = symbols('k m n', integer=True)
>>> f, g, h = symbols('f g h', cls=Function)
>>> init_printing()
Documentation can be found at http://docs.sympy.org/1.0/
>>> Integral(sqrt(1/x), x)
⌠
⎮ ___
⎮ ╱ 1
⎮ ╱ ─ dx
⎮╲╱ x
⌡
>>> sqrt(x)
√x
>>> (sqrt(x) + sqrt(y))**2
2
(√x + √y)
>>>
This is how we can print expressions in a nice format in the interactive console.
Simplification in SymPy
We can use the simplify() method to simplify mathematical expressions to the best possible extent. A large number of expressions are covered under this. The following (Listing 9-8) is an example of this:
Listing 9-8. prog08.py
from sympy import *
x = symbols('x')
print(simplify(sin(x)**2 + cos(x)**2))
print(simplify((x**3 + x**2 - x - 1)/(x**2 + 2*x + 1)))
print(simplify(gamma(x)/gamma(x - 2)))
The simplified output is as follows:
1
x - 1
(x - 2)*(x - 1)
There are more simplification methods in SymPy. We can use expand() for a polynomial expression’s expansion as shown below (Listing 9-9).
Listing 9-9. prog09.py
from sympy import *
x, y = symbols('x y')
print(expand((x + y)**2))
print(expand((x + 3)*(y + 5)))
The following is the expanded output:
x**2 + 2*x*y + y**2
x*y + 5*x + 3*y + 15
Similarly, we can use the factor() method (Listing 9-10) for finding irreducible factors of polynomials.
Listing 9-10. prog10.py
from sympy import *
x = symbols('x')
print(factor(x**3 - x**2 + x))
The output is as follows:
x*(x**2 - x + 1)
Calculus
We can even use SymPy for calculus. We can use the diff() method to calculate the derivatives as follows (Listing 9-11):
Listing 9-11. prog11.py
from sympy import *
x = symbols('x')
print(diff(x**3 - x**2 + x, x))
print(diff(x**5, x))
print(diff(sin(x), x))
The output is:
3*x**2 - 2*x + 1
5*x**4
cos(x)
We can also find the higher order derivative of the expressions as follows (Listing 9-12):
Listing 9-12. prog12.py
from sympy import *
x = symbols('x')
print(diff(10*x**4, x, x, x))
print(diff(10*x**4, x, 3))
The output is as follows:
240*x
240*x
We can also calculate integrals with SymPy using the integrate() method. The following code (Listing 9-13) demonstrates this:
Listing 9-13. prog13.py
from sympy import *
x = symbols('x')
print(integrate(sin(x), x))
The output is as follows:
-cos(x)
We can also have integration with limits as follows (Listing 9-14):
Listing 9-14. prog14.py
from sympy import *
x = symbols('x')
print(integrate(exp(-x), (x, 0, oo)))
Here, we are integrating the exponent of -x from zero to infinity (denoted by oo). Run this and check the output. We can also calculate multiple integrals with multiple limits as follows (Listing 9-15):
Listing 9-15. prog15.py
from sympy import *
x, y = symbols('x y')
print(integrate(exp(-x)*exp(-y), (x, 0, oo), (y, 0, oo)))
Run the code (Listing 9-15) and verify the output by carrying out the integration manually.
Note
SymPy is really a big topic. It cannot be covered completely in a single chapter. I recommend readers explore more on the websites http://docs.sympy.org and www.sympy.org .
Conclusion
In this chapter, we got started with SymPy and learned how to perform symbolic computation in Python. In the next chapter, we will get started with NumPy and Matplotlib.