In this very first chapter, we will start by laying the foundation for our work by installing the tools we need in order to run webpack on our local machine. Then we will talk a little bit about the default configuration that webpack has introduced with version 4, called “zero config.” Next we will write our first “hello world” example, which as you might already know, is a mandatory standard when starting to learn anything new. Finally, we will talk very briefly about the webpack bundling command that we will use in order to build our final output files.
Installing Webpack
First of all, you need to have Node JS installed on your machine because webpack relies on it. If you don’t have Node installed, you can go to https://nodejs.org/en/download/ and follow the instructions based on your operating system.
This will create a file called package.json that will save references to our installed modules.
Via npm, the -y option is to answer yes to all prompted questions; it’s not that important for us at this stage, so we will just say yes to everything.
package.json: The basic generated JSON file
Note that whether you are in Linux, Mac, or Windows, the command is basically the same assuming you have NPM installed already on your machine, which is mostly the case if you followed the installation of Node JS correctly. The --save-dev option is to tell NPM that we need this just for our development purposes, meaning that these packages will be installed on our local machine only.

The package json file after installing webpack and webpack-cli
Notice that webpack and webpack-cli were added with each one’s version under the devDependencies. Also notice that a new folder called node_modules was created, as well as another file called package-lock.json .
So, on one hand, in addition to the basic generated properties (that we have seen above) like the name of project, version, author, etc., package.json lists all the packages that your project directly depends on, meaning that whenever you install a new package from your terminal, that package name and version number will be added to package.json. The package-lock.json, on the other hand, is the full representation of the dependency tree of your project, including the indirect dependencies. For example, when you install webpack, other packages that it depends on will get installed as well. Package-lock.json will also contain the specific version of each module, so that if someone took your project later and ran “npm install” on their machine, they will get the exact same versions you installed. That way there will be no breakdown on the application (i.e., due to a package that got updated and has some break changes).
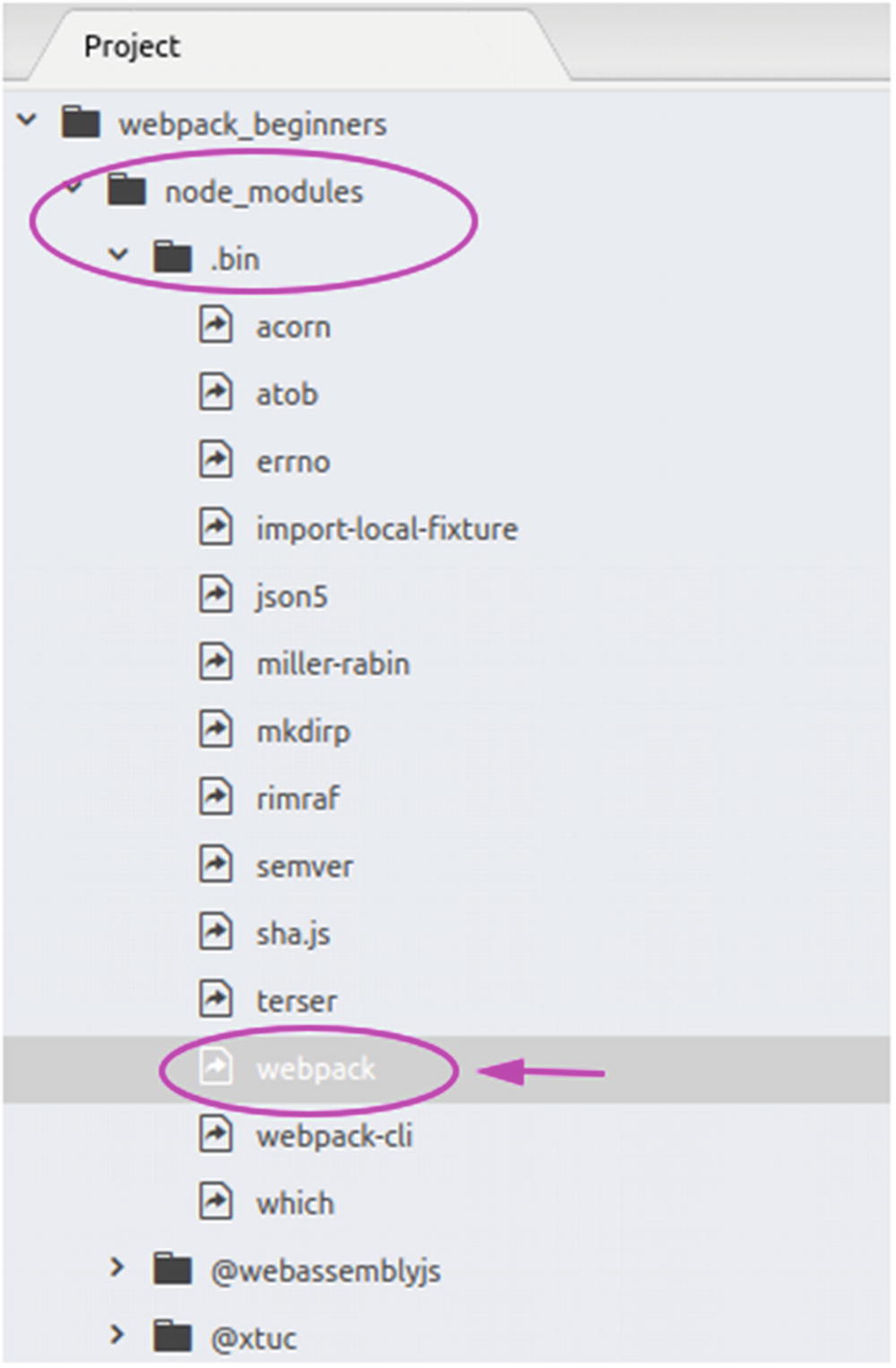
The file “node_modules/.bin/webpack” responsible for running webpack command
The file “webpack” above (under “node_modules/.bin”) is where the “Webpack command” comes from, so every time we need to compile our JavaScript, we'll call this file from our terminal. However, there is a better way to do this (which we will see in the next section) by making an alias command that will call that file for us instead of specifying the path to it. With that in mind, it’s time to write some basic code and start exploring the power of webpack.
Webpack 4 Zero Config
Configuration
Out of the box, webpack won't require you to use a configuration file. However, it will assume the entry point of your project is src/index and will output the result in dist/main.js minified and optimized for production.
What this means is that webpack expects you to create an entry file called index.js inside an src folder, and then it will create and output the result in dist/main.js for you, without the need to create the configuration file yourself or do anything else.

Our “webpack” folder tree after adding an “src” folder and index.js file
What we did here is to tell webpack to bundle our JS. If you wonder what a node_modules/.bin/webpack is, it’s just the path to our webpack command, which is basically a JavaScript file itself. There is a better way to call it for sure, and we will see this in a few moments.
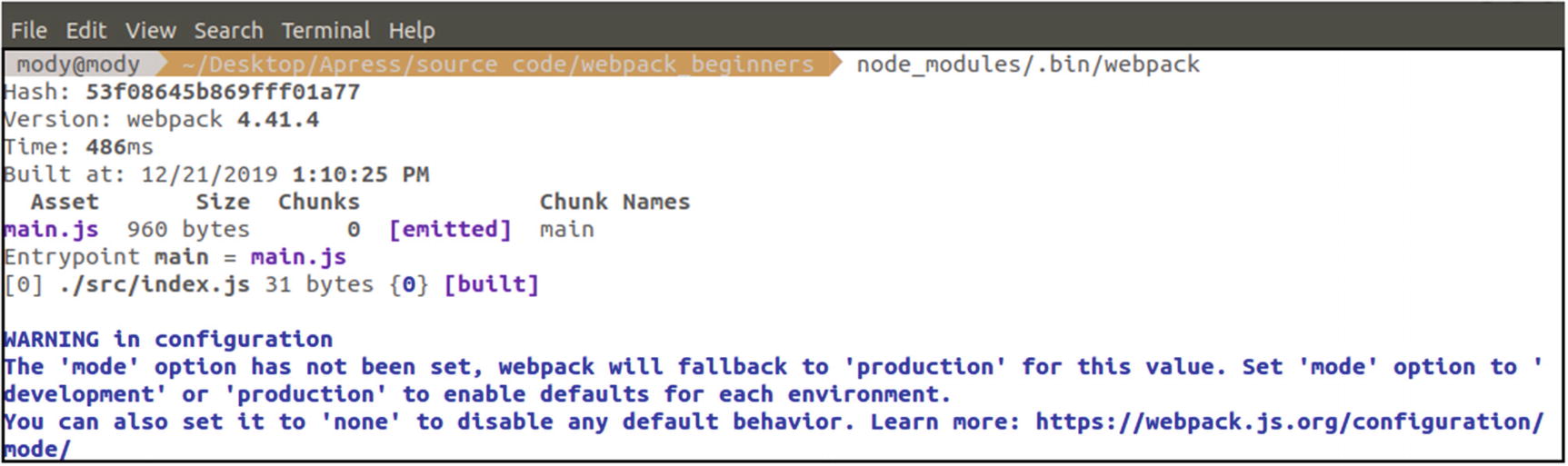
Terminal output of running “node_modules/.bin/webpack” command
WARNING in configuration.
The ‘mode’ option has not been set, so webpack will fall back to ‘production’ for this value. Set 'mode' option to 'development' or ‘production’ to enable defaults for each environment.
You can also set it to 'none' to disable any default behavior. Learn more at https://webpack.js.org/configuration/mode/

main.js: the file generated by webpack under dist folder

Our “Hello webpack world” wrapped in its own module
If you noticed, our alert is at the very bottom, but you can see that there is a bunch of code that precedes it, which you don’t have to worry about or understand because it’s specific to webpack and how it makes the modules requiring functional.
The next step is to create an HTML file and make a link to our dist/main.js to see if everything works fine.
index.html: referencing our dist/main.js file
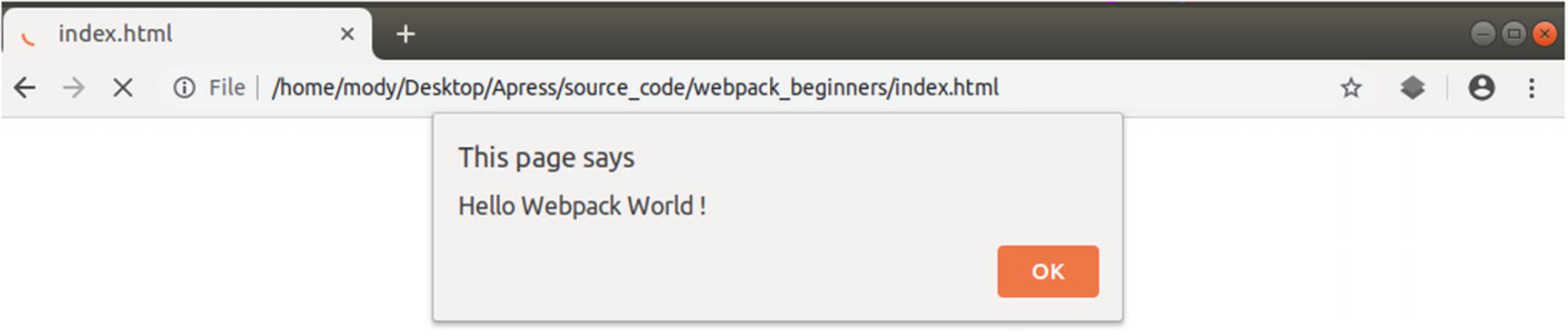
Your first “Hello world” alert
While this is a very basic example of our first working JavaScript file bundled by webpack, it’s time to congratulate yourself! You did it, and you should be proud as you just made your first step in the webpack world. But before we move to more serious things, let’s talk a little bit about the bundling command and how we can use it in a friendlier way to tell webpack to compile our JavaScript.
The Bundling Command
So whatever you name it, you should call it with its name, but you will find that most people generally use “build” or “dev” in the webpack world.
Summary
In this chapter, we introduced the necessary commands to install webpack on our machine. We have seen the package.json file and what it basically does. Then we created our first JavaScript file and bundled it with webpack. Also, we explored the entry file and how it should be named in order for webpack zero config to work correctly. Additionally, we have learned how to run webpack command from our terminal.