Concept of a Struct as a Structure Type
In the previous chapter, we looked at serialization, which allows us to store the state of an object, where an object is an instance of a specific class. We looked at different ways to serialize and store the data, and two such formats were XML and JSON. We also learned how to deserialize the serialized data, which is usually stored in a file, and therefore convert it to the same class structure it was in originally. The reason we serialize an object or objects is to allow it to be transferred across a network or shared with other people. Serialization also works across programming languages . In Chapter 13 we gained knowledge of classes and objects, which formed the foundations for the serialization and deserialization processes. Classes are a structure, and in this chapter we will extend our knowledge of structures by looking at the C# struct.
In C# a struct is a lightweight alternative to a class, but it is not a class. The struct can have members and methods, or put another way, we say it can have data and functionality. A struct in C# is defined using the keyword struct. Interestingly, we have, without thinking, used simple structs already when coding our applications, since all the value types we have used – for example, int, float, double, bool, char, etc. – are structs. Before we begin, let us take a look at the difference between value types and reference types in relation to structs and classes.
Value and Reference Types
When a value type instance is made, there is one single space allocated in memory to store the value. We have discussed primitive data types such as int and float and these are value types like a struct. When the runtime deals with the value type, it is dealing directly with the data.
On the other hand, with reference types, an object is created in memory and dealt with through a pointer.
then myCustomer2Struct would be completely independent of myCustomerStruct and have its own fields.
then myCustomer2Class would be simply a copy of the reference CustomerClass, and therefore myCustomerClass and myCustomer2Class point to the same object.
Difference Between Struct and Class
Structs are value types, and we use the actual struct. On the other hand, a class is a reference type, and we point to the actual class.
Structs cannot be coded by us to have a constructor that has no parameters, a default constructor , since the default constructor is automatically defined and not available to change.
Structs cannot inherit from other structs or classes, whereas a class can inherit from other classes.
Structs can implement one or more interfaces .
Structs cannot declare a finalizer , which is a destructor used to garbage collect.
If we use the new keyword to create an object of the struct, the default constructor is called and the object is created. A struct can be instantiated without using the new keyword, whereas a class cannot be instantiated without using the new keyword.
If the struct is used without the new keyword, the members of the struct will remain unassigned and we cannot use the struct object until we have initialized all the members.
Members, fields, cannot be initialized, for example, public int policy_number = 0; causes an error in C# 10 or lower.
Let's code some C# and build our programming muscle.
We will create a Customer struct in a similar manner to the Customer class we created when we completed Chapter 13 on classes and objects.
- 1.
Right-click the solution CoreCSharp.
- 2.
Choose Add.
- 3.
Choose New Project.
- 4.
Choose Console App from the listed templates that appear.
- 5.
Click the Next button.
- 6.
Name the project Chapter19 and leave it in the same location.
- 7.
Click the Next button.
- 8.
Choose the framework to be used, which in our projects will be .NET 6.0 or higher.
- 9.
Click the Create button.
- 10.
Right-click the Chapter19 project in the Solution Explorer panel.
- 11.
Click the Set as Startup Project option.
- 12.
Right-click the Program.cs file in the Solution Explorer window.
- 13.
Choose Rename.
- 14.
Change the name to CustomerExample.cs.
- 15.
Press the Enter key
- 16.
Double-click the CustomerExample.cs file to open it in the editor window.
Struct with a Default Constructor Only
- 17.
Amend the code, as in Listing 19-1.
Customer struct inside namespace
- 18.
Amend the code, as in Listing 19-2.
Assign values to the Customer struct fields and display the data
- 19.
Click the File menu.
- 20.
Choose Save All.
- 21.
Click the Debug menu.
- 22.
Choose Start Without Debugging.

A series of codes on the console window indicates the specifics of the customer struct objects.
Struct details displayed for the instance
- 23.
Press the Enter key to close the console window.
Struct with a User Constructor
Using the default constructor method to give default values of the members
Having a user-defined constructor method to assign values to the members
- 24.
Right-click the Chapter19 project in the Solution Explorer window.
- 25.
Choose Add.
- 26.
Choose Class.
- 27.
Name the class PolicyExample.cs.
- 28.
Open the PolicyExample.cs file in the editor window.
- 29.
Amend the code, as in Listing 19-3, to create a Policy struct outside the class and inside the namespace.
Create a struct called Policy
Struct Instantiation Without the New Keyword
- 30.
Amend the code as in Listing 19-4.
Instantiate the Policy struct without using the new keyword
- 31.
Hover over the policyNumber code that has the red underline and look at the error message, as shown in Figure 19-2.

A series of codes on the console window using a field that is the policy number, which may or may not has been assigned.
Errors because of unassigned values
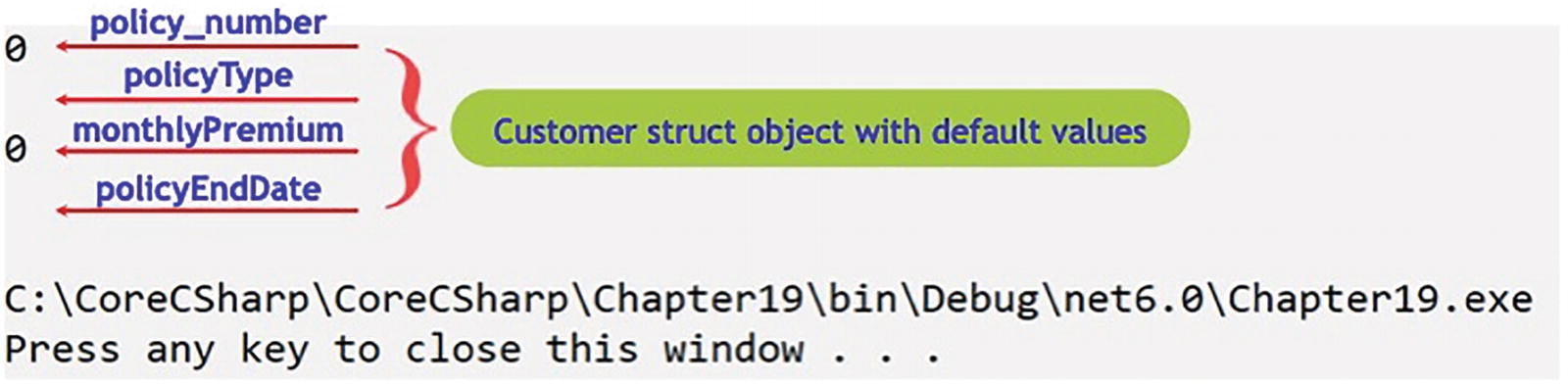
A series of codes on the console window depicts the customer struct object with default values as 0.
Struct default values 0 and null by using the default constructor
If the struct is used without the new keyword, the members of the struct will remain unassigned and we cannot use the struct object until we have initialized all the members.
Struct Instantiation with the New Keyword
- 32.
Amend the code, as in Listing 19-5. Only one line of code needs amended.

A series of codes on the console window indicates the customer struct object with the values that were specified by the customer constructor.
Struct values as set by the instance using the custom constructor
Instantiate the Policy struct using the new keyword
- 33.
Right-click the Chapter19 project in the Solution Explorer panel.
- 34.
Choose Properties from the pop-up menu.
- 35.
Choose the Chapter19.PolicyExample class in the Startup object drop-down list.
- 36.
Close the Properties window.
- 37.
Click the File menu.
- 38.
Choose Save All.
- 39.
Click the Debug menu.
- 40.
Choose Start Without Debugging.
The console window will appear, as shown in Figure 19-3, and show details of the policy. The display shows the default values of 0 for the int and double and null for the two strings.
- 41.
Press the Enter key to close the console window.
Creating a Constructor
The default constructor is automatically defined and not available for change. Remember, a default constructor is parameterless; it accepts no values. However, a struct allows a constructor to be added that contains parameters.
- 42.
Amend the code, as in Listing 19-6.
Add a constructor to the struct
- 43.
Amend the code to add the four values to be passed to the constructor of the struct. Only the one line of code needs amended, as Listing 19-7:
Instantiate using the custom constructor
- 44.
Click the File menu.
- 45.
Choose Save All.
- 46.
Click the Debug menu.
- 47.
Choose Start Without Debugging.
The console window will appear, as shown in Figure 19-4, and show details of the policy with the new initialized values passed to the constructor from the instance.
- 48.
Press the Enter key to close the console window.
Creating Member Properties (Get and Set Accessors)
- 49.
Amend the code, as in Listing 19-8, to make the members of the struct have access modifiers of private.
Make members private

A series of codes on the console window indicates that the policy number cannot be accessed due to the high level of protection it provides.
Inaccessible members due to access modifier
- 50.
Highlight the four members of the struct.
- 51.
Right-click in the highlighted code.
- 52.
Choose Quick Actions and Refactorings as shown in Figure 19-6.

A console window depicts the popup window, which includes quick actions and refactorings, rename, remove and sort usings with their keyboard shortcut.
Quick Actions and Refactorings to add properties
- 53.
Choose Encapsulate field (but still use field).
Member properties created, the get and the set
- 54.
Amend the code, as in Listing 19-10, to refer to the property.
Call the property, not the member, with a capital letter
- 55.
Click the File menu.
- 56.
Choose Save All.
- 57.
Click the Debug menu.
- 58.
Choose Start Without Debugging.

A series of codes on the console window indicates the values for individual customers that were retrieved using getters and setters.
Values retrieved using property accessors
- 59.
Press the Enter key to close the console window.
Encapsulation
The code we have just run produced the same result as the previous example, but we have made use of the properties, get and set, for the private variables. This has therefore given us a little exposure to encapsulation, where we refer to encapsulation as hiding the variables of a class or struct so that they can only be accessed from outside the class or struct by special methods called properties. We achieve encapsulation, as we have just seen, by making the access modifier of the fields private and then generating a property for each member. We do not need to have a property for every member if we do not want to, and we do not have to have a get and a set in each property. This will depend on what we wish for the given situation.
A struct can include concrete methods just like classes can include concrete methods. We have not included methods in our examples, but it would be easy to do so, in the same way we did when we coded the applications in Chapter 13 on classes and objects.
Readonly Struct
struct – In which case the whole struct is readonly. We could therefore say that the structure is immutable; it is “final.” In this case all the members are readonly, and consequently every member property does not require a set accessor, a setter – remember YAGNI. The only way to change the member values is at the time of creating the instance object, which calls the constructor whose purpose is to set the initial values of the members. The members of the readonly struct are readonly, and therefore no method in the struct can change its value, which essentially means that we will not see a statement like myPolicy.PolicyType = "Jewellery".
Member of the struct – From C# 8, a member can be set to readonly, in which case the value of the member cannot be changed. If the member is readonly, then its property does not require a set accessor, a setter. Allowing readonly members means we do not need to make the whole struct readonly.
Creating a Readonly Struct
- 60.
Open the PolicyExample.cs file in the Chapter19 project.
- 61.
Amend the code, as in Listing 19-11, to make the Policy struct readonly.
Readonly struct

A console window in which the code starts with the line, read only struct policy. In the next line, the declared variable policy number is highlighted. A pop up says that instance fields of read only structs must be read only.
Readonly struct needs readonly fields
- 62.
Amend the code, as in Listing 19-12, to make the member fields readonly.
Readonly fields
- 63.
Amend the code, as in Listing 19-13, to comment the setters.
Setters are not required; comment them
- 64.
Click the File menu.
- 65.
Choose Save All.
- 66.
Click the Debug menu.
- 67.
Choose Start Without Debugging.

A series of codes on the console window indicates that the struct can only be read and that the data are retrieved using the getters.
Readonly struct, readonly fields with no setters – code works
- 68.
Press the Enter key to close the console window.
- 69.
Amend the code in the Main() method to attempt to assign a new value to the policyNumber as shown in Listing 19-14.
Attempt to assign a value to a readonly struct
- 70.
Hover over the red underline of PolicyNumber as shown in Figure 19-10.

A console indicates the option that the policy number cannot be applied to the read-only attribute of the variable.
Cannot change readonly struct member
- 71.
Remove the partial line of code.
- 72.
Click the File menu.
- 73.
Choose Save All.
C# 8 readonly Members
Prior to C# 8 we could have a readonly struct and all the fields had to be readonly, as we have just seen in the example we coded. From C# 8 we were introduced to a new feature for the struct , which allows us to declare members of the struct as readonly without the struct being readonly. This has the advantage of making the code more specific and more granular, because we target those fields that need to be readonly rather than having to make all the fields readonly.
- 74.
Right-click the Chapter19 project in the Solution Explorer window.
- 75.
Choose Add.
- 76.
Choose Class.
- 77.
Name the class PolicyExampleReadOnlyMember.cs.
- 78.
Amend the PolicyExampleReadOnlyMember class code, as shown in Listing 19-15.
Creating the struct with readonly fields
- 79.
Amend the class code, as in Listing 19-16.
Instantiate the struct and try to assign values to readonly fields
- 80.
Hover of the red underline of any of the assignment lines, and note the message, as shown in Figure 19-11.

A series of codes on the console window indicates that the policy members and the policy end date cannot be given to its read-only.
Readonly error message only on readonly fields
- 81.
Comment the two lines that are causing the error , as in Listing 19-17.
Comment the lines causing the error (or delete them)
- 82.
Right-click the Chapter19 project in the Solution Explorer panel.
- 83.
Choose Properties from the pop-up menu.
- 84.
Choose the PolicyExampleReadOnlyMember class in the Startup object drop-down list.
- 85.
Close the Properties window.
- 86.
Click the File menu.
- 87.
Choose Save All.
- 88.
Click the Debug menu.
- 89.
Choose Start Without Debugging.

A series of codes on the console window indicates that the two fields could not be edited and could only be read. It exhibits the values set with the constructor.
Using readonly fields
So we have shown that readonly members cannot be assigned new values. The values are set through the custom constructor, if there is one, or the parameterless default constructor.
C # 8 Nullable Reference Types
In Chapter 6 on data types, we looked at conversion and parsing, and we read about the C# 8 nullable reference type . We read how we could use the ? to ensure that a null value was acceptable, given that from C# 8, reference types are non-nullable by default. In our reading we used two code examples using strings, one using the ? and the other not using it:
For some reinforcement, we will apply the nullable reference type to the struct example we have just completed, but this will require the project to have Nullable enabled, which is done within the project’s .csproj file.
Null-state analysis and variable annotations are disabled by default for existing projects – meaning that all reference types continue to be nullable. Starting in .NET 6, they're enabled by default for new projects.
Enable Nullable in the .csproj file
The nullable annotation context and nullable warning context can be set for a project using the <Nullable> element in your .csproj file. This element configures how the compiler interprets the nullability of types and what warnings are emitted.
Note:
Deference warnings occur when the application deferences (tries to obtain the address of something held in a location from a pointer) and expects it to be valid but is returned a null.
An assignment warning is issued when we try to assign incorrectly, for example, in an if construct, we might try to do if(amount = amountLimit) but we would get an assignment warning as it should be if(amount == amountLimit).
The Microsoft site also gives us details about the contexts we can use:
All reference types are nullable.
We do not need to use the ? suffix.
Deference warnings are disabled.
Assignment warnings are disabled.
All reference types are non-nullable unless declared using the ?.
We can use the ? suffix.
Deference warnings are enabled.
Assignment warnings are enabled.
All reference types are nullable but members are considered not null at the opening brace of methods.
We can use the ? suffix but it produces a warning.
Deference warnings are enabled.
Assignment warnings are not applicable.
If we use annotations, then
All reference types are non-nullable unless declared using the ?.
We can use the ? suffix and it declares the nullable type.
Deference warnings are disabled.
Assignment warnings are disabled.
- 90.
Amend the PolicyReadOnlyMembers struct so that the policyType is assigned a null value within the constructor, as in Listing 19-19.
Assign a null value to the policyType field
- 91.
Hover over the word null, as in Figure 19-13, and read the warning message.

A series of codes on the console window indicates that it is impossible to convert the null literal to a reference type that cannot be null.
Null literal error message
We will now amend the declaration of the policyType within the struct, so that the type string is marked with the ?:
private string ? policyType;
- 92.
Amend the code, as in Listing 19-20.
Using the ? to permit a null value
- 93.
Click the File menu.
- 94.
Choose Save All.
- 95.
Click the Debug menu.
- 96.
Choose Start Without Debugging.

A series of codes on the console window indicates that the null value can be used whenever the private string is being accessed.
Null value used for policyType field
Chapter Summary
So, finishing this chapter on structs, we can see the similarity with classes and objects. A struct is a value type, which must have a default parameterless constructor, but they can also have custom constructors. The fields can be set to private and we can use properties, getters and setters, to access them. The whole struct can be set to readonly and this means all fields must also be readonly, but from C# 8 we can have readonly fields without the struct being readonly. Finally, we looked at nullable references and the use of the ? to permit null values.
Wow, what an achievement. This is not basic coding. We are doing some wonderful things with our C# code. We should be immensely proud of the learning to date. In finishing this chapter, we have increased our knowledge further and we are advancing to our target.

An illustration of concentric circles with two differently colored regions above a text that reads, Our target is getting closer.