Arrays: A Data Structure
We learned in Chapter 10 that iteration is a very important programming concept in all programming languages. To use iteration in our C# code, we have a number of construct options, and the best construct option to choose will depend on the particular task the code has to perform. The different constructs for iteration are the for construct, the while construct, the do while construct, and the foreach construct. Within the constructs, there are options to break out of the iterations completely or to break out of a particular iteration using the continue keyword. In terms of the project structure, we once again used the ability to have multiple classes within a package where each class must have a unique name.
List of integers
List of real numbers
List of characters
List of strings
Surface cleaners | This could be a list of strings. |
Hand soaps | This could be a list of strings. |
Product codes | This could be a list of integers. |
Insurance types | This could be a list of strings. |
Account numbers | This could be a list of integers. |
Insurance premiums | This could be a list of doubles. |
Vehicle manufacturers | This could be a list of strings. |
Vehicle models | This could be a list of strings. |
An array is therefore a list of related items that can be treated by C# as one object. For now, we could say that an array is a number of variables that can be treated as one object. So, when we think of an array, we should understand that we are dealing with individual variables or objects, but with the added advantage of them being organized for us in one object.
If we have the array object with the data items “lumped” together into one object, we are said to have what is called a data structure . In programming, data structures may be very complex or more simplistic and will be in the form of a sequence of data items such as a data record or array. In a program for a playing card game, like solitaire, we might want to keep a record that holds information about a card, that is, the suit and the value. This means we will have two fields in the record. With the C# programming language, we have access to data structures that we can use to accommodate this type of record. Such structures in C# include an array and a data structure called a struct.
The array must be assigned a data type.
After the data type will be an open square bracket followed by a closing square bracket.
The square brackets can come immediately after the data type, or there can be a space after the data type, just before the opening square bracket.
The array will have a single name, which is called its identifier.
The array is of fixed size and cannot be made bigger or smaller, so it is not a dynamic structure.
When we initialize or populate the array, we must ensure that each item in the array is of the same data type, as identified by the data type assigned to the array. We therefore say that an array is homogenous , having similarity in structure.
The square brackets come after the data type, for example, string[], and not after the identifier.
Putting the brackets after the identifier, for example, string claimAmounts[],is not permitted.
The size of the array is not part of its type, and this means we can declare an array using initial values, for example, string[] claimAmounts = { "Home", "Auto", "Life" };.
Single-dimensional arrays
Multidimensional arrays
Jagged arrays (also known as an array of arrays)
Declare the array.
Initialize the array.
Reference the members of the array.
We will see that there are different ways to declare and create arrays and different ways to initialize arrays, so it is important at the outset to understand that we will find our own preferred option from the various approaches. Each approach will have its advantages and disadvantages, but as a developer we will usually have a preferred option. On the other hand, as a developer we will spend much of our time maintaining code rather than writing new code, and often the code we maintain has not been written by us, so we need to understand all the approaches.
Single-Dimensional Arrays
Insurance types | This could be a list of strings. |
Account numbers | This could be a list of integers. |
Insurance premiums | This could be a list of doubles. |
Vehicle manufacturers | This could be a list of strings. |
Vehicle models | This could be a list of strings. |
A list of insurance types of data type string, for example:
{ "Auto", "SUV 4x4", "Motorcycle", "Motorhome", "Snowmobile", "Boat"};
Representation of the insuranceTypes single-dimensional array
Array name | [0] | [1] | [2] | [3] | [4] | [5] |
---|---|---|---|---|---|---|
insuranceTypes | Auto | SUV 4x4 | Motorcycle | Motorhome | Snowmobile | Boat |
String[] insuranceTypes = new String[6]; | ||
insuranceTypes[0] | = | "Auto"; |
insuranceTypes[1] | = | "SUV 4x4"; |
insuranceTypes[2] | = | "Motorcycle"; |
insuranceTypes[3] | = | "Motorhome"; |
insuranceTypes[4] | = | "Snowmobile"; |
insuranceTypes[5] | = | "Boat"; |
A list of account numbers (integers):
{ 000001, 001122, 002233, 003344, 004455, 005566};
Representation of the accountNumber single-dimensional array
Array name | [0] | [1] | [2] | [3] | [4] | [5] |
---|---|---|---|---|---|---|
accountNumber | 000001 | 001122 | 002233 | 003344 | 004455 | 005566 |
int[] accountNumber = new int[6]; | ||
accountNumber [0] | = | 000011; |
accountNumber [1] | = | 001122; |
accountNumber [2] | = | 002233; |
accountNumber [3] | = | 003344; |
accountNumber [4] | = | 004455; |
accountNumber [5] | = | 005566; |
A list of insurance costs (doubles):
{ 104.99, 105.99, 106.99, 107.99, 108.99, 109.99};
Representation of the insurancePremiums single-dimensional array
Array name | [0] | [1] | [2] | [3] | [4] | [5] |
---|---|---|---|---|---|---|
insurancePremiums | 104.99 | 105.99 | 106.99 | 107.99 | 108.99 | 109.99 |
double[] insurancePremiums = new double[6]; | ||
insurancePremiums [0] | = | 104.99; |
insurancePremiums [1] | = | 105.99; |
insurancePremiums [2] | = | 106.99; |
insurancePremiums [3] | = | 107.99; |
insurancePremiums [4] | = | 108.99; |
insurancePremiums [5] | = | 109.99; |
Now that we have the concept of an array being a collection, a container, or a store for items of the same data type, we can look at how to code the implementation of an array. Like many things in life, we have choices. So C# gives us choices, different techniques, that allow us to set up and use arrays.
Choice 1: Declaring and Creating an Array in Two Stages
Stage 1: Declare
When we say that we are declaring an array in C#, we are actually saying that we want to use an array that will consist of items of the data type stated, but it will not exist yet.
Stage 2: Create
Now, when an array has been declared, it needs to be created. To create the array, it must be instantiated, and this can be achieved by using the new keyword syntax.
string[] insuranceTypes; | declaration | |
insuranceTypes = | new string[6]; | creation |
int[] accountNumber; | declaration | |
accountNumber = | new int[6]; | creation |
double[] insurancePremiums; | declaration | |
insurancePremiums = | new double[6]; | creation |
In instantiating the array, we are setting aside the required memory resources for the array of the specified size and data type.
Choice 2: Declaring and Creating an Array in One Stage
Declaration | Creation | |
---|---|---|
string[] insuranceTypes | = | new string[6]; |
int[] accountNumber | = | new int[6]; |
double[] insurancePremiums | = | new double[6]; |
Declaring the data type of the array – string, int, or double
Stating that the array is single dimensional – this is the [ ] part
Giving the array its name – insuranceTypes, accountNumber, or insurancePremiums
Instantiating the array with the new keyword
Stating that it will contain six elements

A screenshot depicts an array named account Number with 6 values for the data type 'int'. The default value for the data type int is 0.
Array filled with default values for the int type
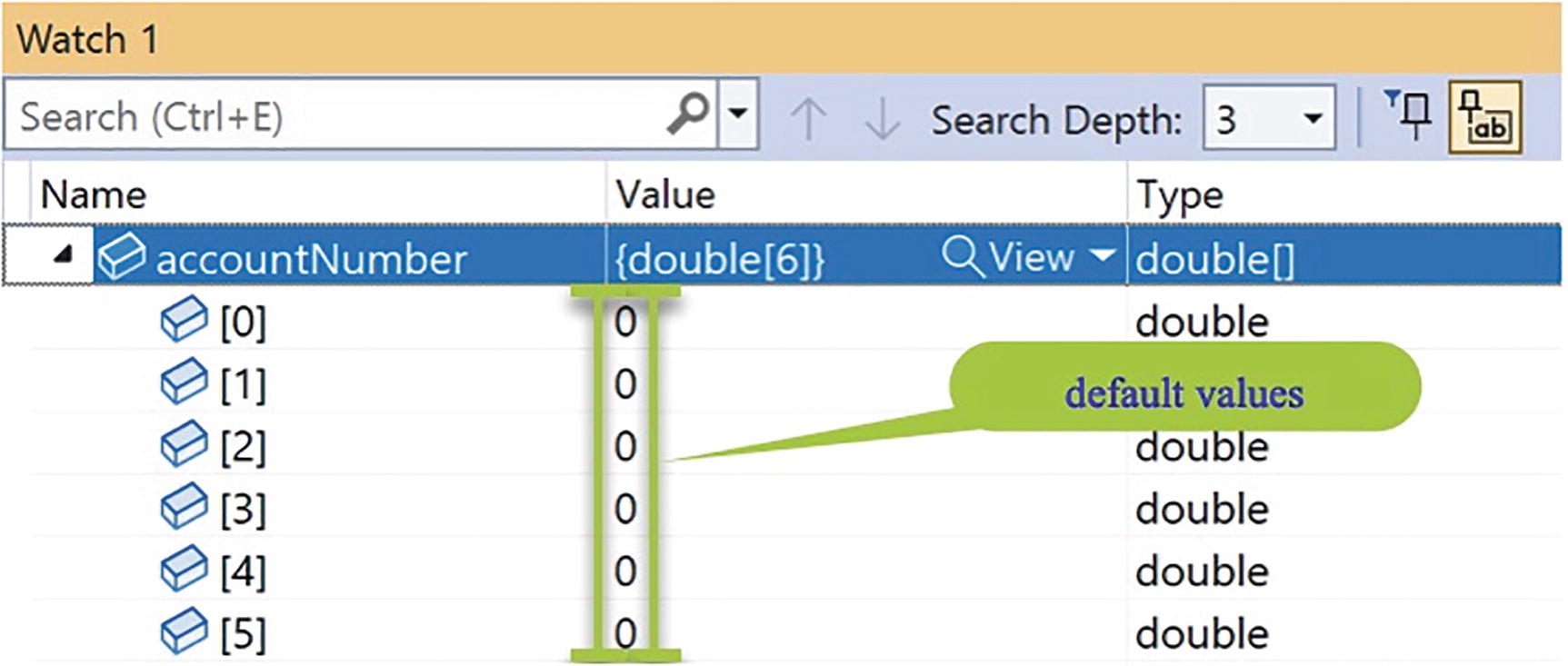
A screenshot depicts an array named account Number with 6 values for the data type 'double'. The default value for the data type double is 0.
Array filled with default values for the double type
Referencing the Array Elements
The array name
The numeric position of the element we wish to access, remembering what was said earlier about C# using zero-based referencing
The syntax is arrayname[position in array – 1]
Example: insuranceTypes[2]
Insurance type single-dimensional array as shown in Table 11-1: | |
insuranceTypes [0] = "Auto"; | First item is indexed as 0. |
insuranceTypes [1] = "SUV 4x4"; | Second item is indexed as 1. |
insuranceTypes [2] = "Motorcycle"; | Third item is indexed as 2. |
insuranceTypes [3] = "Motorhome"; | Fourth item is indexed as 3. |
insuranceTypes [4] = "Snowmobile"; | Fifth item is indexed as 4. |
insuranceTypes [5] = "Boat"; | Sixth item is indexed as 5. |
Account number single-dimensional array as shown in Table 11-2: | |
accountNumber [0] = 000011; | First item is indexed as 0. |
accountNumber [1] = 001122; | Second item is indexed as 1. |
accountNumber [2] = 002233; | Third item is indexed as 2. |
accountNumber [3] = 003344; | Fourth item is indexed as 3. |
accountNumber [4] = 004455; | Fifth item is indexed as 4. |
accountNumber [4] = 004455; | Sixth item is indexed as 5. |
Insurance cost single-dimensional array as shown in Table 11-3: | |
insurancePremium [0] = 104.99; | First item is indexed as 0. |
insurancePremium [1] = 105.99; | Second item is indexed as 1. |
insurancePremium [2] = 106.99; | Third item is indexed as 2. |
insurancePremium [3] = 107.99; | Fourth item is indexed as 3. |
insurancePremium [4] = 108.99; | Fifth item is indexed as 4. |
insurancePremium [4] = 109.99; | Sixth item is indexed as 5. |
- 1.
Right-click the solution CoreCSharp.
- 2.
Choose Add.
- 3.
Choose New Project.
- 4.
Choose Console App from the listed templates that appear.
- 5.
Click the Next button.
- 6.
Name the project Chapter11 and leave it in the same location.
- 7.
Click the Next button.
- 8.
Choose the framework to be used, which in our projects will be .NET 6.0 or higher.
- 9.
Click the Create button.
- 10.
Right-click the project Chapter11 in the Solution Explorer panel.
- 11.
Click the Set as Startup Project option.
- 12.
Right-click the Program.cs file in the Solution Explorer window.
- 13.
Choose Rename.
- 14.
Change the name to Arrays.cs.
- 15.
Press the Enter key.
- 16.
Double-click the Arrays.cs file to open it in the editor window.
- 17.
In the editor window, add the code in Listing 11-1.
Class template with the Main() method
Note that the class name matches the filename, Arrays.
The repair shop unique id, data type string
The vehicle insurance policy number, data type string
The claim amount, data type double
The date of the claim, data type Date
When we coded this program as part of the last chapter on iteration, we were aware that any data entered was not stored by the program code. We were made aware that this “flaw” would be rectified using an array. So now the time has come to amend the last program so that the data entered by the repair shop will be stored, for the duration that the program runs. It will not be available after the program is closed; that is why we marked the word permanent as “permanent” at the start of the chapter, indicating that permanent relates to the duration of the application run rather than forever. If we require the data after the application is exited, we could store the data in a text file or database, and later in Chapter 16, we will see how to store data permanently in a file.
Declare an array, having decided what data type the array will hold.
Remember the information at the start of this chapter when we read that an array can only hold variables of the same data type – an array is homogenous. We have strings, a double, and a Date, so what data type will we use? Well, one answer is the string data type. This will mean that the Date will have to be converted to a string value. We could even have a separate array for each data type, but for simplicity we are just going to use data type string.
Use a name for the array. Here we will use the name repairShopClaims.
Create the array using the new keyword and stating the size of the array.
Add the values to the array in the correct position.
- 18.
Amend the code, as in Listing 11-2, to declare and create the array that will hold the eight items of data input by the user.
Declare and create the array with eight values
- 19.
Amend the code, as in Listing 11-3, to add the variables to be used.
Add the variables
- 20.
Amend the code, as in Listing 11-4.
Ask user for number of claims and convert it to an integer
- 21.
Amend the code, as in Listing 11-5.
Adding the start of a do while loop
- 22.
Amend the code , as in Listing 11-6.
Ask user for repair shop id and read the value
- 23.
Amend the code, as in Listing 11-7.
Add input to the array and increment the counter
- 24.
Amend the code, as in Listing 11-8.
Ask user for policy number and read the value
- 25.
Amend the code, as in Listing 11-9.
Add input to the array and increment the counter
- 26.
Amend the code, as in Listing 11-10.
Ask user for claim amount and read the value
- 27.
Amend the code, as in Listing 11-11.
Add input to the array and increment the counter
- 28.
Amend the code, as in Listing 11-12, to ask the user to input the date of the claim and then read the user input .
Ask user for claim date, read the value, and convert it to a Date
- 29.
Amend the code , as in Listing 11-13.
Add input to the array and increment the counter
- 30.
Amend the code, as in Listing 11-14.
Increment the numberOfClaimsEntered counter
- 31.
Amend the code, as in Listing 11-15, to finish the do while loop by adding the Boolean condition to be tested.
Complete the do while iteration construct
Depending on the number of claims the user wishes to make, the do while loop will be executed again the required number of times. This is great, but our only problem will be verifying that the details have been stored in the array. This now offers us a great opportunity to use the last type of iteration, foreach, that was mentioned in the last chapter.
foreach Loop
var represents the data type of the array or collection items but we can use any data type from the C# language.
item is a variable representing the member of the array. The item in the array at the current position.
The name item is a variable name, and we can call it whatever we like, for example, thememberofthearray.
in is a keyword and must be used in this position.
collection represents the name of the array or collection we wish to iterate.
foreach loop
var represents the data type of the array or collection items.
item has been replaced with the variable name itemInTheClaimsArray.
in is the keyword.
repairShopClaims represents the collection.
In the write line statement, the variable itemInTheClaimsArray has been displayed.
- 32.
Amend the code, as in Listing 11-17, to add the foreach iteration .
foreach iteration
- 33.
Click the File menu.
- 34.
Choose Save All.
- 35.
Click the Debug menu.
- 36.
Choose Start Without Debugging.
- 37.
Type 2 and press the Enter key.
- 38.
Type RS000001 for the repair shop id and press the Enter key.
- 39.
Type VP000001 and press the Enter key.
- 40.
Type 1999.99 and press the Enter key.
- 41.
Type 2021/10/01 and press the Enter key.

A screenshot depicts a console screen with questions and inputs for iteration 1. The questions are about the number of claims, repair shop i d, vehicle policy number, claim amount, and repair date.
Iteration 1
- 42.
Type RS000001 for the repair shop id and press the Enter key.
- 43.
Type VP000002 and press the Enter key.
- 44.
Type 2999.99 and press the Enter key.
- 45.
Type 2021/10/01 and press the Enter key.

A screenshot depicts a console screen with questions and input for iteration 2. The questions are about repair shop i d, vehicle policy number, claim amount, and repair date.
Iteration 2

A screenshot depicts a console screen with 8 array items fetched from the 2 iterations.
Foreach iteration shows the eight array items
- 46.
Press any key to close the console window.
Now that we have the basics of an array, we can now explore arrays further and see some of the possible errors associated with them.
- 1.
Right-click the Chapter11 project in the Solution Explorer panel.
- 2.
Choose Add.
- 3.
Choose Class.
- 4.
Name the class ArrayErrors.cs.
- 5.
Click the Add button.
- 6.
Create a Main() method within the class, as this was not produced automatically, and delete the unwanted imports.
- 7.
Right-click the Chapter11 project in the Solution Explorer panel.
- 8.
Choose Properties from the pop-up menu.
- 9.
Choose the ArrayErrors.cs class in the Startup object drop-down list.
- 10.
Close the Properties window.
- 11.
Amend the code, as in Listing 11-18, to declare and create the array using a variable for the size of the array.
Create a variable and use it for the array size

A screenshot depicts an error that a variable name, 'number of entries being made', is not initialized.
Error when variable not initialized
The error message shown in Figure 11-6 is saying that we cannot use an unassigned variable for the array size. So we will simply add a line of code that will ask the user to input the number of entries they are going to make and assign this value to the variable. Now the program will be happy as it will have a value for the variable – the variable is not unassigned. What we can see is that even though the actual value of the variable is not known, the program is happy as it will know the value before the array is created.
- 12.
Amend the code, as in Listing 11-19.
Read the user input – after array declaration
- 13.
Amend the code, as in Listing 11-20, to move the block of code we have just entered to above the array declaration statement .
Read the user input – before array declaration
Great, the red underline has disappeared, and the compiler is happy. So now we know that we must tell the compiler the size of the array to make it happy. We can use a variable, but this must be known when the array is declared and created.
- 14.
Amend the code, as in Listing 11-21, to add the other variables we will use.
Adding the extra variables we require
- 15.
Amend the code, as in Listing 11-22, to add the loop and the questions.
Adding the do while loop and the user questions
- 16.
Amend the code, as in Listing 11-23, to add the iteration construct and display the array values.
Adding the foreach iteration to display the array values
- 17.
Click the File menu.
- 18.
Choose Save All.
- 19.
Click the Debug menu.
- 20.
Choose Start Without Debugging.
- 21.
Type 2 for the number of entries to be made.
- 22.
Press the Enter key.
- 23.
Type VP000001 for the vehicle policy number.
- 24.
Press the Enter key.
- 25.
Type 10000.
- 26.
Press the Enter key.
- 27.
Type VP000002 for the vehicle policy number.
- 28.
Press the Enter key.
- 29.
Type 20000.
- 30.
Press the Enter key.

A screenshot depicts a console screen with questions and inputs of 2 iterations. The questions are about vehicle policy number and odometer reading.
Both iterations completed

A screenshot depicts a console screen with 4 array items fetched from the 2 iterations.
Foreach iteration displays the array items
Array depiction
[0] | [1] | [2] | [3] |
---|---|---|---|
VP000001 | 10000 | VP000002 | 20000 |
IndexOutOfBounds Exception
An array is of fixed size, and if we try to read or write a value that is outside the boundary of the array, we will be causing an exception. In C# the error is known as an IndexOutOfBounds exception because it happens when we have made the value of the index, the counter, larger than the size of the array. Remember that the index starts at 0, not 1.
- 31.
Amend the code, as in Listing 11-24, to add 1 to the end of the do while.
Adding +1 to the end of the do while loop
- 32.
Click the File menu.
- 33.
Choose Save All.
- 34.
Click the Debug menu.
- 35.
Choose Start Without Debugging.
- 36.
Type 2 for the number of entries to be made.
- 37.
Press the Enter key.
- 38.
Type VP000001 for the vehicle policy number.
- 39.
Press the Enter key.
- 40.
Type 10000.
- 41.
Press the Enter key.
- 42.
Type VP000002 for the vehicle policy number.
- 43.
Press the Enter key.
- 44.
Type 20000.
- 45.
Press the Enter key.
- 46.
Type VP000003 for the vehicle policy number.
- 47.
Press the Enter key.
Our array was made to have a size of
2 entries multiplied by the 2 values in each entry = 4
This means that the positions available in the array are
odometerReadings[0]
odometerReadings[1]
odometerReadings[2]
odometerReadings[3]
Our variable is 4, and we are therefore trying to write to position
odometerReadings[4]

A screenshot depicts a runtime error. It is about an unhandled exception as the index was out of bounds of the array.
Array out-of-bounds exception
Removing the +1 from the end of the do while loop
- 48.
Click the File menu.
- 49.
Choose Save All.
Ranges and Indices: C# 8 and Above
- 1.
Right-click the project Chapter11 in the Solution Explorer panel.
- 2.
Choose Add.
- 3.
Choose Class.
- 4.
Name the class IndicesAndRanges.cs.
- 5.
Click the Add button.
- 6.
Create a Main() method within the class, as this was not produced automatically, and delete the unwanted imports.
Remember the shortcut to create the Main() method is to type svm and then press the Tab key twice.
- 7.
Right-click the Chapter11 project in the Solution Explorer panel.
- 8.
Choose Properties from the pop-up menu.
- 9.
Choose the IndicesAndRanges.cs class in the Startup object drop-down list.
- 10.
Close the Properties window.
C# 8 introduced the concept of ranges to collections along with two new operators. When using ranges, we now have the index and the range, which can be used to index and slice the collection. Both index and range are part of the System namespace. When we think about what we have done so far with arrays, we have worked from the start of the zero-indexed array and never really thought about starting from the end of the array, and we use the index of the element or elements when we need access to them. Now, in C# 8 we have been given an index expression that allows us to access the collection from the end. The hat operator, ^, means “index from end,” so ^2 would give us the second element from the end. The syntax is the ^ followed by an integer value or a variable that can be converted to an integer, and we should still be aware that we can still get an IndexOutOfRange exception if we use an incorrect integer value.
the fact that we have tried to skip 12 on line 1 when there are only 4 items and take 12 on line 2 when there are only 2 items remaining does not cause an out-of-range exception. However, if the source was null, we would get a System.ArgumentNullException: Value cannot be null error.
C# 8 however changes things, and we are now able to use the new range operator, start..howmany, and we can leave out the start or the end. The .. syntax is called the range operator.
- 11.
Amend the code, as in Listing 11-26, to declare and initialize the array.
Declare and create the array
We will amend the code to iterate the array and use the index from end within the console output . In this example we use ^(employeeAndSalary.Length – (counter)), which means
Find the length of the array.
Subtract the counter value from it, for example, 6 – 0 = 6.
But with the ^ it becomes ^6, which means from the end take the sixth element, which we know is really the first item in the array or index zero.
- 12.
Add the new code within the Main() method, as in Listing 11-27.
Iterate the array and use the hat operator , index from end
- 13.
Click the File menu.
- 14.
Choose Save All.
- 15.
Click the Debug menu.
- 16.
Choose Start Without Debugging.

A screenshot depicts a console screen with 6 values. The screen displays the names of 3 employees and their salaries based on hat operator and index from the end expression.
Using the hat operator ^, “index from end”
- 17.
Press the Enter key to close the console window.
- 18.
Amend the code, as in Listing 11-28, to use the index from end to display the second element from the end of the array.
Using the index from end, ^, to display the second element
- 19.
Click the File menu.
- 20.
Choose Save All.
- 21.
Click the Debug menu.
- 22.
Choose Start Without Debugging.

A screenshot depicts a console screen with array elements based on index and hat operator. The output line is repeated twice.
Traditional index and the hat operator
- 23.
Press the Enter key to close the console window.
- 24.
Amend the code, as in Listing 11-29, to use the length and index from end to display the last element of the array.
Using the length and index from end , ^
- 25.
Click the File menu.
- 26.
Choose Save All.
- 27.
Click the Debug menu.
- 28.
Choose Start Without Debugging.

A screenshot depicts a console screen that displays the last item of an array using the length property and hat operator. The output line is repeated twice.
Length and the hat operator
- 29.
Press the Enter key to close the console window.
Range
In this code we will look at the traditional method GetRange(), from the LINQ library, to get a sequence of values from the array. We will also use the Skip() and Take() methods, which are also from the LINQ library. We will then use the new range operator .. to locate elements.
- 30.
Amend the code as in Listing 11-30.
Using the ToList() and GetRange() methods
- 31.
Click the File menu.
- 32.
Choose Save All.
- 33.
Click the Debug menu.
- 34.
Choose Start Without Debugging.

A screenshot depicts a console screen that lists 4 array items from elements 2 to 6 using get range and to list functions.
GetRange() and ToList() methods start at the third item for four items
- 35.
Press the Enter key to close the console window.
- 36.
Amend the code, as in Listing 11-31, to use the Skip() and Take() methods and display the items.
Using the Skip() and Take() methods
- 37.
Click the File menu.
- 38.
Choose Save All.
- 39.
Click the Debug menu.
- 40.
Choose Start Without Debugging.

A screenshot depicts a console screen that lists 4 array items from elements 2 to 6 using skip and take functions.
Skip() and Take() methods
- 41.
Amend the code to use the range operator and display the items.
Using the range operator [2..^2]
- 42.
Click the File menu.
- 43.
Choose Save All.
- 44.
Click the Debug menu.
- 45.
Choose Start Without Debugging.

A screenshot depicts a console screen that lists 2 array elements using range operator. The range starts from index 2 and stops at element 2 from the end.
Range operator [2.. ^2]
Chapter Summary
Arrays are used to hold a collection of items all of the same data type.
An array is homogeneous.
Arrays are of fixed size. Once we declare the size of the array, it cannot be altered.
Arrays hold the data for the duration that the program runs.
There are single-dimensional arrays, which we have used in this chapter, but there are also multidimensional arrays.
Items in an array are referenced by their index, also called the subscript.
The indexes start at 0, not 1 – arrays are zero indexed.
The foreach loop is an ideal iterator to use with arrays; however, it is not suitable if we need to reference a counter since no counter exists in the foreach construct.
If we try to exceed the maximum index of the array, we will get an IndexOutOfBounds exception.
There are different ways to access the elements of the array using the range features like the hat operator, ^, which means “index from end,” and the range operator .., which effectively lets us “slice” the array.
We can have more than one class in a project.
We are making great progress in our programming of C# applications and we should be proud of our achievements. In finishing this chapter and increasing our knowledge, we are advancing to our target.

An illustration depicts concentric circles. Some inner circles are shaded. A label reads, Our target is getting closer.