C# Practice Exercises
The lab exercises that follow will give us an opportunity to practice what we have learned. We should complete the labs by referring to the book chapters when we are unsure about how to do something, but more importantly we should look at the previous code we have written. The code we have written should be an invaluable source of working code, and it is important not to “reinvent the wheel.” Use the code - copy, paste, and amend it if required. Reuse the code – that is what the professional developer would do and is expected to do. Professional software developers are expected to create applications as fast and accurately as possible, and reusing existing code is one technique they apply, so why should we be any different?
If we really get stuck, there are sample solutions following the labs and we can refer to these, but it is important we understand any code that we copy and paste. It is also important we enjoy the challenge of developing solutions for each lab. We will apply the learning from the chapters, but more importantly we will develop our own techniques and style for coding , debugging , and problem solving.
Life begins at the edge of our comfort zone.
We will inevitably feel at the edge of our programming ability, but every new thing we learn in completing each lab should make us feel better and encourage us to learn more. While we may be “frightened” and “uncomfortable” completing the coding labs, the process will lead us to grow and develop our coding skills and build our programming muscle. We might find it “painful” at times but that is the reality of programming. We will find it exciting and challenging as we are stretched and brought to a place we have not been to before.
Chapter 4 Labs: WriteLine( )
Lab 1
Write a C# console application, using the WriteLine() command, that will display the letter E using *’s to form the shape, for example, one line could be Console.WriteLine(“*******”);.
Lab 2
Write a C# console application, using the WriteLine() command, that will display the letter A using *’s to form the shape, for example, one line could be Console.WriteLine (“ *”);.
Lab 3
Write a C# console application that will display your name and address in a format that might look like a label for an envelope – name on the first line, address line 1 on the second line, etc.
Lab 4
Using the same code that you developed for Lab 3, the name and address label, add a statement between each of the name and address lines that will require the user to press Enter on the keyboard before the display moves to the next line .
Lab 1: Possible Solution with output shown in Figure 25-1

A window has a series of asterisks formed in the shape of an upper case letter E.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-2

A window has a series of asterisks formed in the shape of an upper case letter A.
Lab 2 output
Lab 3: Possible Solution with output shown in Figure 25-3

A window contains 5 lines that read as follows, Mister Gerard Byrne, 1 any street, any road, Belfast, B T 1 1 A N, respectively.
Lab 3 output
Lab 4: Possible Solution with output shown in Figure 25-4

A window has the texts, Mister Gerard Byrne, 1 any street, any road, Belfast, and B T 1 1 A N. Between each is the line, press any key to continue.
Lab 4 output
Chapter 6 Labs: Data Types
Lab 1
Write a C# console application that will calculate and display the area of a rectangle using a length of 20 and a breadth of 10, which should be hard-coded in the code. The formula for the area of a rectangle is length multiplied by breadth.
Lab 2
Write a C# console application that will calculate and display the area of a rectangle using the length and breadth that are input at the console by the user. The formula for the area of a rectangle is length multiplied by breadth.
Lab 3
Using the code from Lab 2, write a C# console application that will calculate and display the volume of a cuboid using the length, breadth, and height that are input at the console by the user. The formula for the volume of a cuboid is length multiplied by breadth multiplied by height.
Lab 4
Credit card number – Contains 16 digits and hyphens between each 4 digits
Card expiry month – A number from 1 to 12 (Jan to Dec)
Card expiry year – A two-digit number for the year, for example, 23
Card issue number – A single-digit number
Three-digit security code – A three-digit number
Card holder name on card – A string
Display to the console the details read from the user.
Lab 1: Possible Solution with output shown in Figure 25-5

A window contains a text that reads as follows, The area of the rectangle is 200 square meters.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-6
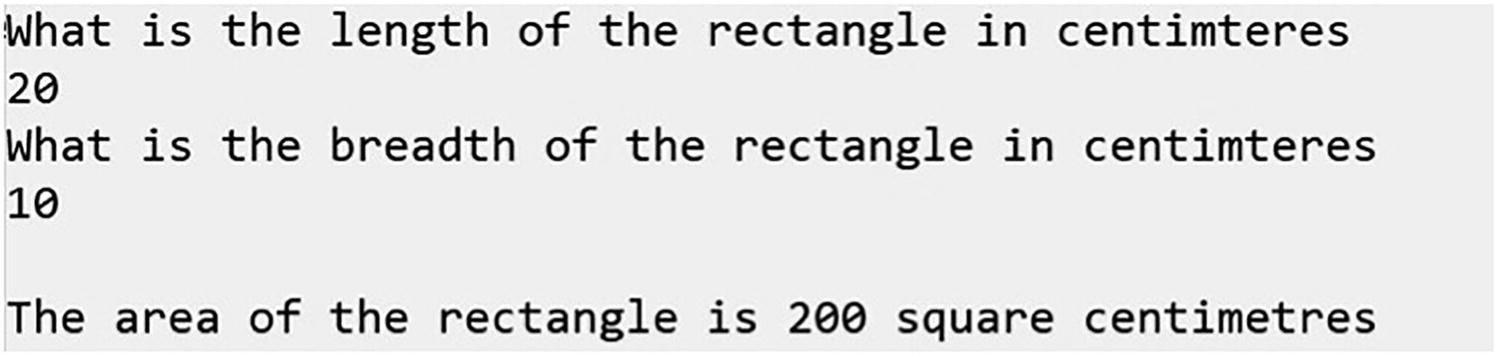
A window has 5 lines of text. The first and third lines ask for the length and breadth of the rectangle, the second and fourth are the answers, and the fifth states the area of the rectangle.
Lab 2 output
Lab 3: Possible Solution with output shown in Figure 25-7

A window has 7 lines of text. The first, third, and fifth lines ask for the length, breadth, and height of the rectangle, the answers are below each, and the last line states the volume of the cuboid.
Lab 3 output
Lab 4: Possible Solution with output shown in Figure 25-8
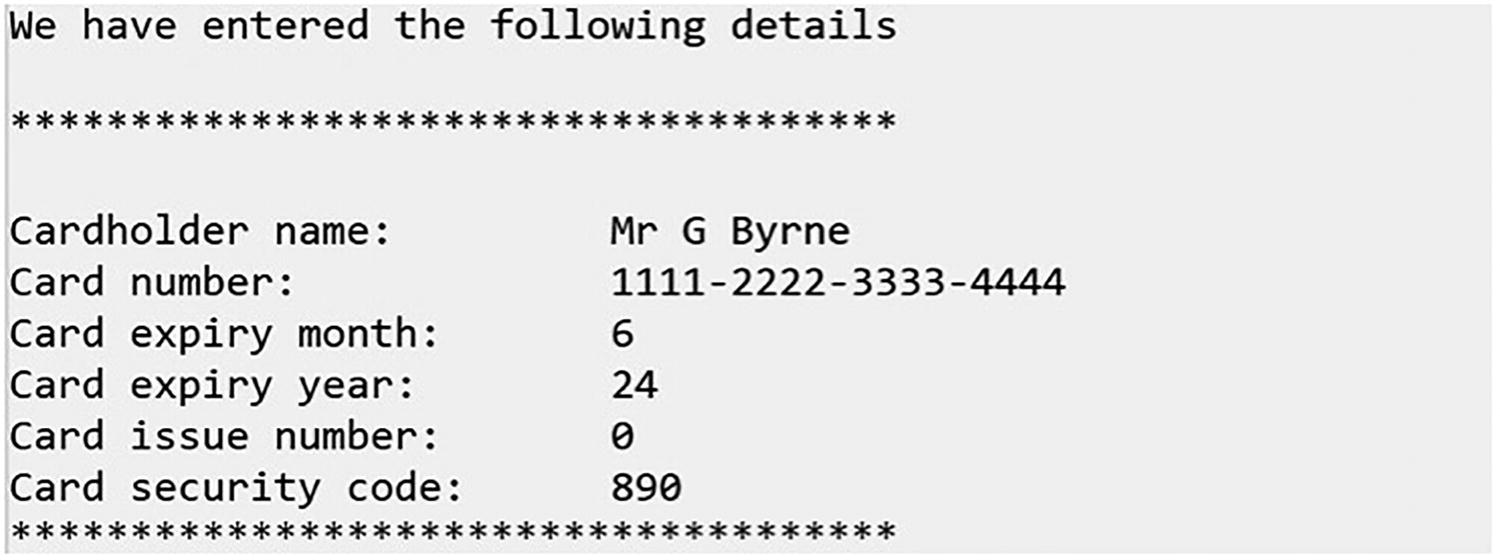
A window has the text, We have entered the following details. Below are details like the cardholder name, card number, and expiry year, among others.
Lab 4 output
Chapter 7 Labs: Data Conversion and Arithmetic
Lab 1
Write a C# console application that will calculate the number of points accumulated by a sports team during their season. The program should ask the user to input the number of games won, the number of games drawn, and the number of games lost. The program should total the number of games played and calculate the number of points won based on the facts that 3 points are given for a win, 1 point is given for a draw, and 0 points are given for a lost game. Display the number of games played and the number of points accumulated.
Lab 2
The total score for two examinations that a student undertakes
The average of the two scores
The two scores will be input by the user at the console and will be accepted as string values by the program code, so conversion will be needed.
Lab 1: Possible Solution with output shown in Figure 25-9

A window contains 10 lines of text including questions as to how many games were won, drawn, and lost, among others. The answers are given as well.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-10

A window has 8 lines of text including questions as to what the scores are for tests 1, and 2, among others. The answers are given as well.
Lab 2 output
Chapter 8 Labs: Arithmetic
Lab 1
Allow a user to input the number of hours worked by an employee.
Allow a user to input the rate per hour, which the employee is paid.
Calculate the gross wage, which is the hours worked multiplied by the rate per hour.
Calculate the amount of national insurance to be deducted, where the rate of national insurance is 5% of the gross wage.
Calculate the amount of income tax to be deducted, where the formula to be used is 20% of the gross wage after the national insurance has been deducted from the gross wage.
Display a simplified wage slip showing, for example, the gross wage, the deductions, and the net pay.
How many hours were worked? 40
What was the rate per hour? £10.00
- Payslip
Hours – 40
Rate – £10.00
Gross – £400.00
National insurance deductions – £20.00
Tax deductions – £76.00
Net pay – £304.00
Lab 1: Possible Solution with output shown in Figure 25-11

A window has text for the number of hours worked and the hourly rate. A pay slip below details the hours worked, gross, and net pay, among others.
Lab 1 output
Chapter 9 Labs: Selection
Lab 1
Write a C# console application that will ask the user to input a numeric value representing the month of the year, 12, and the number of days in that month will be displayed. Use a switch construct.
Lab 2
Marks greater than or equal to 90 receive Distinction.
Marks greater than or equal to 75 receive Pass.
Marks lesser less than 75 receive Unsuccessful.
Use an if-else construct.
Lab 3
Write a C# console application that will ask the user to input the name of one of the programming languages – C#, Python, or Java – and a short description of the language will be displayed.
Use an if-else construct and be careful when comparing the String values.
Lab 1: Possible Solution with output shown in Figure 25-12

A window contains 3 lines of text that read as follows, enter the numeric number of the month, 6, and month 6 has 30 days.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-13

A window has 3 lines of text. The first line reads, enter the examination mark, colon, and the second and third, 78, and 78 marks is a pass grade.
Lab 2 output
Lab 3: Possible Solution with output shown in Figure 25-14

A window has 6 lines of text where the first line reads, enter the programming language, colon. The other lines detail the programming language used.
Lab 3 output
Chapter 10 Labs: Iteration
Lab 1
Write a C# console application that will display a table showing a column with pound sterling values (£) and a second column showing the equivalent amount in US dollars ($). The pound amounts should be from £1 to £10, and the exchange rate to be used is $1.25 for each £1.00.
Lab 2
Write a C# console application that will display a table showing a column with pound sterling values (£) and a second column showing the equivalent amount in US dollars ($). Remember, reuse code. Lab 1 code might be a great starting point.
The application will ask the user to enter the number of pounds they wish to start their conversion table at and then ask them to enter the number of pounds they wish to stop their conversion table at. The application will display a table showing a column with pound values (£), starting at the user’s start value and ending at the user’s end value, and a second column showing the equivalent amount in US dollars ($). The exchange rate to be used is $1.25 for each £1.00.
Lab 3
Write a C# console application that will continually ask the user to input the name of a programming language, and the message “There are many programming languages including (the language input by the user)” will be displayed . The question will stop being asked when the user inputs X as the language. The message should not be displayed when X has been entered. The program will just exit.
Example Output
There are many programming languages including C#.
There are many programming languages including JavaScript.
Lab 4
Write a C# console application that will ask the user to input how many new vehicle registration numbers they wish to input. The application will continually ask the user to input a vehicle registration number until the required number of registrations have been entered. When the vehicle registration number has been entered, a message will display the number of entries that have been made.
Lab 1: Possible Solution with output shown in Figure 25-15

A window has two columns of text with headers, pounds sterling and United States dollar. Each column contains 10 lines of numerical entries.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-16
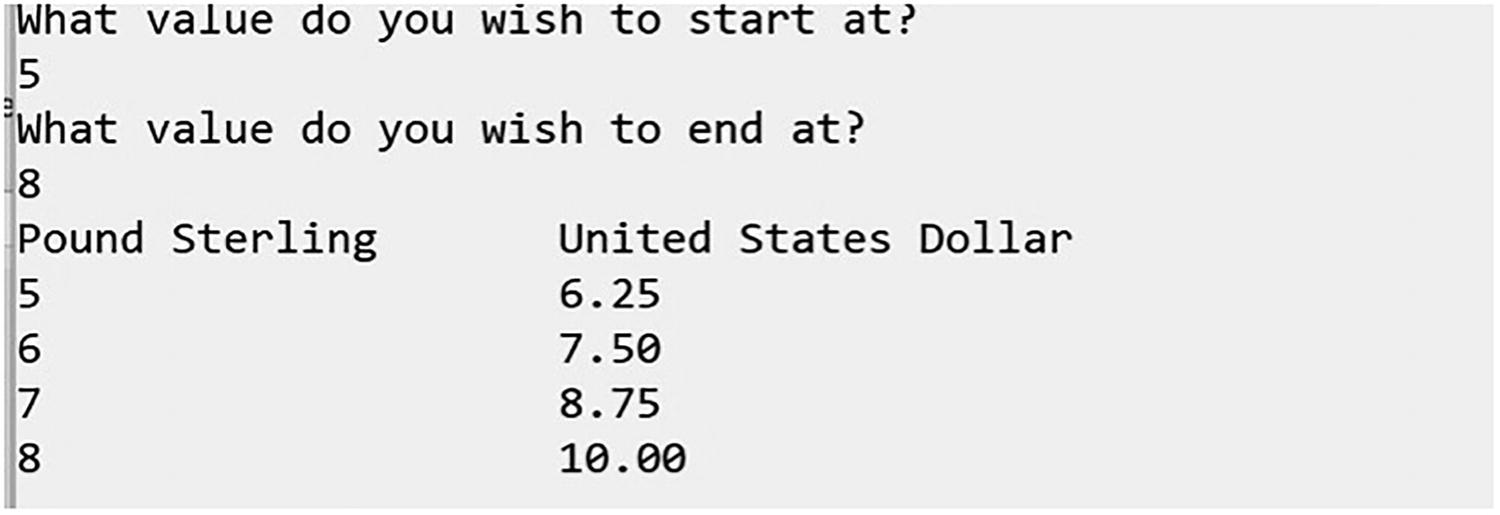
A window has questions as to what value you wish to start and end at, and their respective answers. 2 columns below have headers, pound sterling, and United States dollar.
Lab 2 output
Lab 3: Possible Solution with output shown in Figure 25-17

A window has 9 lines of text. It includes questions about what is the programming language, and the respective answers, among others.
Lab 3 output
Lab 4: Possible Solution with output shown in Figure 25-18

A window has 9 lines of text. It includes questions about how many new vehicle registrations are entered, and what the registration numbers are, among others.
Lab 4 output
Chapter 11 Labs: Arrays
Lab 1
Write a C# console application that will use an array with the claim values: 1000.00, 4000.00, 3000.00, 2000.00. The application should calculate and display the total, average, minimum, and maximum value of the claims.
Lab 2
Write a C# console application that will ask the user to enter four employee names, store them in an array, and then iterate the array to display the names.
Lab 3
Write a C# console application that will read an array that contains a list of staff names alongside their salary and then increase the salary by 10% (1.10), and write the new details to a new array. The application should then iterate the new array and display the employee’s name in column 1 and their new salary in column 1.
Lab 1: Possible Solution with output shown in Figure 25-19

A window has 4 lines of text which include the total of the claims, the average, maximum, and minimum claim values. The amounts for each are given.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-20

A window has 12 lines of text which include questions as to what the names of employees 1 to 4 are. The answers are given after each question.
Lab 2 output
Lab 3: Possible Solution with output shown in Figure 25-21

A window has two columns of text with headers, employee name, and new salary. Under employee names are, Gerry Byrne, Peter Johnston, and Ryan Jones.
Lab 3 output
Chapter 12 Labs: Methods
Lab 1
Calculate the total of the claim values (void method).
Calculate the average of the claim values (void method).
Calculate the minimum of the claim values (void method).
Calculate the maximum of the claim values (void method).
Display a message that states each of the calculated values (void method).
(Refer to Chapter 11 Lab 1 as the code is the same, but it is sequential.)
Lab 2
Total of the claim values (value method, returns a double)
Average of the claim values (value method, returns a double)
Minimum of the claim values (value method, returns a double)
Maximum of the claim values (value method, returns a double)
and a PARAMETER method that accepts the four calculated values to display a message that states each of the calculated values. This parameter method will not return a value; it is also a void method.
The application should only use variables that are local to the methods we use. The declaration of the array can be at the class level.
Lab 1: Possible Solution with output shown in Figure 25-22

A window has 4 lines of text which include the total of the claims, the average, maximum, and minimum claim values. The amounts for each are given.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-23

A window has 4 lines of text which include the total of the claims, the average, maximum, and minimum claim values. The amounts for each are given.
Lab 2 output
Chapter 13 Labs: Classes
Lab 1
- A class called CalculatedValues , with no Main() method, and inside it
Declare an array with the claim values: 1000.00, 4000.00, 3000.00, 2000.00.
- Use separate VALUE methods to calculate the
Total of the claim values (value method, returns a double)
Average of the claim values (value method, returns a double)
Minimum of the claim values (value method, returns a double)
Maximum of the claim values (value method, returns a double)
Declare a PARAMETER method that accepts the four calculated values to display a message that states each of the calculated values. This parameter method will not return a value; it is also a void method.
- A class called ClaimCalculator , with the Main() method, and inside it
Instantiate the CalculatedValues class.
Call each of the four value methods and assign the returned values to variables.
Pass the four variables to the parameter method, which will display the values.
The application should only use variables that are local to the methods we use. The declaration of the array can be at the class level.
Lab 2
- A class called QuoteMethodsClass and inside it
- Create separate methods to ask the user to input
Their name
The age of their vehicle
The engine capacity of their vehicle
Calculate the quote value based on the following formula:
100 * (engine capacity/1000) * (10/age of vehicle)
Create a method to display the quote amount.
Example Test 1
Engine cc 1600
Age of vehicle 2
Quote value = 100 * (1600/1000) * (10/2) = 100 * 1.6 * 5 = 800
Example Test 2
Engine cc 3000
Age of vehicle 10
Quote value = 100 * (3000/1000) * (10/10) = 100 * 3 * 1 = 300
- A class called QuoteCalculatorClass and inside it
Instantiate the QuoteMethodsDetails class.
Call each of the five methods.
The display should show the quote amount and the details that were input.
Lab 1: Possible Solution with output shown in Figure 25-24

A window has 4 lines of text which include the total of the claims, the average, maximum, and minimum claim values. The amounts for each are given.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-25

A window has 8 lines of text that include questions like the name of the customer, and the age of the vehicle. The answers to the questions are given.
Lab 2 output
Chapter 14 Labs: Interfaces
Lab 1
- An interface called IVehicleInsuranceQuote and inside it there will be
An interface method that returns no value, has no parameters, and is called AskForDriverAge
An interface method that returns no value, has a parameter of type int to hold the age, and is called AskForVehicleValue
An interface method called CalculateQuote that returns no value and has two parameters, one of type int to hold the driver age and the other of type double to hold the vehicle value
- A class called VehicleInsuranceQuote that implements the IVehicleInsuranceQuote interface and the methods will
Ask the user to input their age at their last birthday.
Ask the user to input the value of the vehicle being insured.
Calculate the monthly premium based on the following formula:
(60/age of driver) * (vehicle value/5000) * 10
Example: 20-year-old driver with a car of value 50000
Monthly premium is (60/20) * (50000/5000) * 10, which is (3) * (10) * 10, which is 300.
Have an additional method to display the quote details – driver age, vehicle value, and quote amount.
A class called QuoteCalculator with a Main() method that calls the AskForDriverAge() method. The AskForDriverAge() method should call the AskForVehicleValue() method, passing it the age. The AskForVehicleValue() method should call the CalculateQuote() method, passing it the age and value. And the CalculateQuote() method should call the DisplayQuote() method, passing it the age, value, and quote amount.
Lab 1: Possible Solution with output shown in Figure 25-26

A window has 7 lines of text that include questions about the age of the driver and the value of the vehicle. The answers are given as well.
Lab 1 output
Chapter 15 Labs: String Handling
Lab 1
- Have a class called Registrations and inside it
Declare an array of strings with the following vehicle registrations: ABC 1000, FEA 2222, QWA 4444, FAC 9098, FEA 3344.
- Have a method to
Find all vehicle registrations beginning with an F and display them in the console window.
Lab 2
- Have a class called ClaimsPerState and inside it
Declare an array of strings with the following claim details: 1000IL, 2000FL, 1500TX, 1200CA, 2000NC, 3000FL.
- Have separate methods to
Display the full array of claim details in alphabetical order.
Check whether a given string ends with the contents of another string and, if it does, write it to the console. In this example we will look for the string FL.
Read the claim values and find the total of all the claim values given that the claim values are the first four numbers in the claim string.
Lab 1: Possible Solution with output shown in Figure 25-27

A window has 4 lines of text where the first line reads as, registrations beginning with F. The next three lines are registration numbers.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-28

A window has 11 lines of text which include entries under sorted array elements and claims for the state of F L. The total claims value is given.
Lab 2 output
Chapter 16 Labs: File Handling
Lab 1
Have a class called WriteRegistrationsToFile.
Ask a user to input five vehicle registrations with the following format: three letters followed by a space followed by four numbers, for example, ABC 1234.
Write each of the five vehicle registrations to a new line in a text file called vehicleregistrations.txt.
Lab 2
Have a class called ReadRegistrationsFromFile.
Declare an array of strings called vehicleRegistrations.
Read the five lines from the vehicleregistrations.txt file created in Lab 1 and add them to the array.
Iterate the array and display each vehicle registration.
Lab 1: Possible Solution with output shown in Figure 25-29
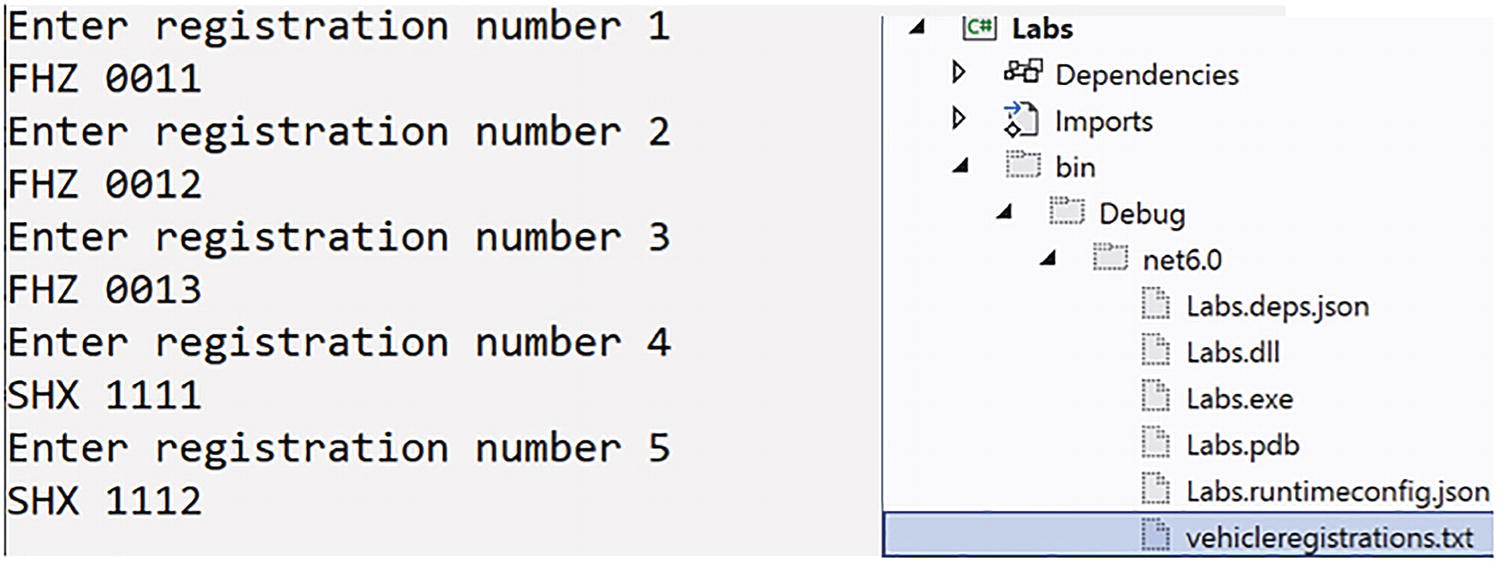
A window has 10 lines of text for registration number entries 1 to 5. On the right is a folder tree with the file, vehicle registrations dot t x t, highlighted.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-30

A window is titled, vehicle registrations dot t x t. A console is open on its right with a list of vehicle registration numbers.
Lab 2 output
Chapter 17 Labs: Exceptions
Lab 1
- Have a class called OutOfBoundsException, which will contain a Main() method that will have code to
Declare an array of integers called claimValues containing the following claim values: 1000, 9000, 0, 4000, 5000.
Iterate the array of values and display each value to the console.
Have a try block that contains the code to display the sixth array item.
Have a catch block that will catch an IndexOutOfRangeException and display the exception message.
Lab 2
- Have a class called MultipleTryCatch, which will contain a Main() method that will have code to
Declare an array of integers called claimValues containing the following claim values: 1000, 9000, 0, 4000, 5000.
Have a try block that contains the code to iterate the array of values and divide each value into 10000 displaying the answer in the console.
Have a catch block that will catch an IndexOutOfRangeException and display the exception message.
Have a catch block that will catch a DivideByZeroException and display the exception message.
Have a finally block that will display a message to say “In finally block tidying up”.
Lab 1: Possible Solution with output shown in Figure 25-31

A window has 6 lines. The first 5 lines are number entries, and the last line reads, exception is, dash, index was outside the bounds of the array.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-32

A window has 4 lines of text. The first 3 lines are about dividing 10000 by 1000, 9000, and 0, respectively, along with their answers.
Lab 2 output
Chapter 18 Labs: Serialization of a Class
Lab 1
Have a class called Vehicle, which implements Serializable.
The Vehicle class will haveTwo private string properties called vehicleManufacturer and vehicleType
One private nonserialized property called vehicleChassisNumber
A constructor using all three properties
Getters and setters for the properties
- Have a class called VehicleJson, which will
Instantiate the Vehicle class and pass details of a vehicle to the constructor, for example:
Vehicle myVehicle = new Vehicle("Ford", "Mondeo", "VIN 1234567890");
Write the serialized data to a file called vehicleserialized.ser.
Read the serialized data file and display the vehicle details.
Lab 2
- Have a class called AgentEntity , which implements Serializable
- The AgentEntity class will have
- The following private properties:
agentNumber, which is of data type int
agentYearsOfService, which is of data type int
agentFullName, which is of data type string
- The following private nonserialized properties:
agentDOB, which is of data type string
agentCapitalInvestment, which is of data type double
A constructor using all the properties
Getters and setters for all the properties
- Have a class called AgentJson, which will
- Instantiate the AgentEntity class and pass details of an agent to the constructor, for example:AgentEntity myAgentEntity = new AgentEntity(190091, 25, "Gerry Byrne", "01/01/1970", 50000.00);
Write the serialized data to a file called agentserialized.ser.
Read the serialized data file and display the agent details.
Lab 1: Possible Solution with output shown in Figure 25-33

A 5-line code on the left mentions the vehicle manufacturer, type, and chassis number. A tree folder on the right has the file, vehicle serialized dot s e r, highlighted.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-34

A window has a 3-line code on top mentioning agent details. Below are the agent details which include the agent number, and full name, among others.
Lab 2 output
Chapter 19 Labs: Structs
Lab 1
- Have a class called AutoInsurance , which will have
- A struct called Vehicle, which will have
Variables to hold the vehicle manufacturer, chassis number, color, and engine capacity
A constructor that accepts values for all four variables
Getters and setters for the variables
A method to display the manufacturer name
A method to display the engine capacity
- Have a Main() method that will have code to
- Instantiate the Vehicle struct and pass details of a vehicle to the constructor, for example:Vehicle myVehicle = new Vehicle("Ford", "VIN 1234567890", "Blue",1600);
Call the method that displays the manufacturer name.
Call the method that displays the engine capacity.
Lab 2
- Have a class called PropertyInsurance , which will have
- A struct called Apartment, which will have
Variables to hold the number of rooms, area of the floor, and the estimated value
A constructor that accepts values for all three variables
A constructor that accepts values for the number of rooms variable and the area of the floor variable and sets the estimated value to be 1000000
A method to display a message stating the values of the three struct members
- Have a Main() method that will have code to
Instantiate an Apartment struct and pass details of an apartment with all three values to the constructor, for example:
Apartment studioOne= new Apartment(2, 50, 120000);Instantiate an Apartment struct and pass details of an apartment with only two values to the constructor, for example:
Apartment studioTwo= new Apartment(3, 60);Call the method that displays the apartment details for studioOne.
Call the method that displays the apartment details for studioTwo.
Lab 1: Possible Solution with output shown in Figure 25-35

A window has 2 lines of text where the first line reads as follows, the vehicle manufacturer is Ford. The second line reads the engine capacity is 1600.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-36

A window has 9 lines of text. The details of apartments are given like the number of rooms, floor area, and estimated value, among others.
Lab 2 output
Chapter 20 Labs: Enumerations
Lab 1
- Have a class called AutoInsurance, which will have
An enumeration called Manufacturer, which will have the values Ford, Chevrolet, Jeep, and Honda
An enumeration called Color, which will have the values Red, Blue, and Green
- A struct called Vehicle, which will have
Variables to hold the vehicle manufacturer of enum type Manufacturer and the vehicle color of enum type Color
A constructor that accepts values for the two variables of enum type Manufacturer and enum type Color
A method to display the manufacturer name and the vehicle color
- Have a Main() method that will have code to
Instantiate a Vehicle struct and pass details of a vehicle to the constructor, for example:
Vehicle myVehicleOne = new Vehicle(Manufacture.Ford, Color.Blue);Call the method that displays the vehicle details.
Instantiate a Vehicle struct and pass details of a vehicle to the constructor, for example:
Call the method that displays the vehicle details.
Lab 2
- Have a class called PropertyInsurance, which will have
An enumeration called InsuranceRiskEnum, which will have the values Low = 1, Medium = 10, and High = 20
An enumeration called LocationFactorEnum, which will have the values NotNearRiver, NearRiver
An enumeration called PropertyTypeEnum, which will have the values Bungalow, House, and Apartment
- Have a Main() method that will have code to
Call an AskForPropertyType() method that returns an int and assign it to a variable of type int called propertyType.
The method will display a menu and return the integer value entered:Console.WriteLine("What is the property type, 1 2 or 3?");Console.WriteLine("1. Bungalow ");Console.WriteLine("2. House");Console.WriteLine("3. Apartment");int propertyType = Convert.ToInt32(Console.ReadLine());return propertyType;Call an AskForPropertyLocation() method that returns a string and assign it to a variable of type string called isNearARiver.
The method will ask if the property is within 50 meters of a river and returns the value entered, either Y or N:Console.WriteLine("Is the property within 50 metres " +"of a river?");string nearARiver = Console.ReadLine();return nearARiver;Call an AskForPropertyValue() method that returns a double and assign it to a variable of type double called estimatedValue.
The method will ask for the property value and return the estimated value:Console.WriteLine("What is the estimated value of " +"the property?");double estimatedValue = Convert.ToDouble(Console.ReadLine());return estimatedValue;Call the method QuoteAmount(), passing it the values for the propertyType, isNearARiver, and estimatedValue.
Calculate the quote amount based on the following formula:
double quoteAmount = (propertyTypeRiskFactor *(propertyValue / 10000) * locationFactorThe propertyTypeRiskFactor is based on the property type in the enum InsuranceRiskEnum – Bungalow is High, House is Medium, and Apartment is Low.
The locationFactor is whether the property is located near a river and uses the enum LocationFactorEnum – Y (Yes) is 10, N (No) is 1.Call the method that displays the quote details.
Lab 1: Possible Solution with output shown in Figure 25-37

A window has 4 lines of text. The first and third lines mention the vehicle manufacturers, and in the second and fourth, the colors of the vehicles.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-38

A window has 14 lines of text. It contains questions and answers regarding the type of property, the closeness to the river, and the estimated value.
Lab 2 output
Chapter 21 Labs: Delegates
Lab 1
- Have a class called CustomerAccount and inside it
Declare a static variable double currentBalance.
Declare a method called AddFunds . The method is a value method that returns a value of type double, accepts a value of type double, adds the value passed in to the current balance, and returns the new balance.
Define the delegate at the namespace level (this is step 1 of 3):
public delegate double AmendFundsDelegate(double amount); - Have a Main() method that will
Instantiate the CustomerAccount class as
CustomerAccount myCustomer = new CustomerAccount();Instantiate the delegate AmendFundsDelegate amendBalance; and then create the new object, passing it the AddFunds method (this is step 2 of 3):
amendBalance = new AmendFundsDelegate(myCustomer.AddFunds);Have a variable of type double called transactionAmount, assigning it a value of 100.00.
Invoke the delegate, passing it the transactionAmount (this is step 3 of 3).
Display the new account balance.
Lab 2
Define the delegate at the namespace level (this is step 1 of 3):
- Have a class called Calculator and inside it
Declare a method called Add. The method is a void method that accepts two values of type int and displays the sum of the two values.
Declare a method called Subtract. The method is a void method that accepts two values of type int and displays the difference between the two values.
- Have a class called CalculatorApplication and inside it
- Have a Main() method that will
Instantiate the Calculator class as
Calculator my Calculator = new Calculator();- Instantiate the delegate (this is step 2 of 3):CalculatorDelegate myDelegate1 = new CalculatorDelegate(myCalculator.Add);
Invoke the delegate, passing it the two required values 8 and 2 (this is step 3 of 3):
myDelegate1.Invoke(8, 2);
Repeat steps 2 and 3 for the Subtract method, passing in 8 and 2.
Lab 1: Possible Solution with output shown in Figure 25-39

A window has a line of text that reads as follows, the new balance is, colon, 100.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-40

A window has 2 lines of text where the first line reads, the total of 8 and 2, comma, is 10. The second line reads, the difference between 8 and 2, comma, is 6.
Lab 2 output
Chapter 22 Labs: Events
Lab 1
- Have a class called Calculator and inside it
Define the delegate:
public delegate void CalculatorDelegate();Define the event that links to the delegate:
public event CalculatorDelegate NumberGreaterThanNine;- Have an Add() method that
Accepts two integers as its parameters.
Adds the two numbers and assigns the answer to a variable called answer.
Will have a selection construct to check if the answer is greater than 9. If it is, the event NumberGreaterThanNine() is called; otherwise, no action is needed.
Display the two numbers and the answer in the console.
- Have another class called CalculatorApplication, in the same namespace, and inside it
- Have a Main() method that will
Instantiate the Calculator class as
Calculator myCalculator = new Calculator();- Bind the event with the delegate and point to the EventMessage() method:myCalculator.numberGreaterThanNine +=new Calculator.CalculatorDelegate(EventMessage);
Call the Add() method from the Calculator class, passing it the values 8 and 2:
myCalculator.Add(8,2);Outside the Main() method but inside the CalculatorApplication class- Create the EventMessage() method so that it outputs a message:static void EventMessage(){Console.WriteLine("*Number greater than 9 detected*");}
Lab 2
- Have a class called RepairClaimCheckerLogic and inside it
- Define the delegate:public delegate void RepairClaimCheckerDelegate(int claimNumber, double claimValue);
- Define the event that links to the delegate:public event RepairClaimCheckerDelegate OverLimit;
Declare and create an array to hold three values of data type double:
double[] repairClaimsAmounts = new double[3];- Have a GetRepairClaimData() method that
Accepts no parameters
Will have an iteration construct to iterate three times and ask the user to input the repair claim amount, storing each input value in the array of doubles
- Have a ReadAndCheckRepairClaims() method that
Accepts no parameters
Will have an iteration construct to iterate three times and read the repair claim amount from the array of doubles and check if the value is greater than 5000. If the value is greater than 5000, then the overLimit event is called, passing it the position of the value in the array and the value that is exceeding the 5000 limit.
- Have another class called RepairClaimChecker, in the same namespace, and inside it
- Have a Main() method that will
- Instantiate the RepairClaimCheckerLogic class asRepairClaimCheckerLogic myRepairClaimCheckerLogic = new RepairClaimCheckerLogic();
Call the GetRepairClaimData() method from the RepairClaimCheckerLogic class:
myRepairClaimCheckerLogic.GetRepairClaimData();- Bind the event with the delegate and point to the OverLimitMessage() method:myRepairClaimCheckerLogic.overLimit+= new RepairClaimCheckerLogic.RepairClaimCheckerDelegate(OverLimitMessage);
Outside the Main() method but inside the RepairClaimChecker class, create the OverLimitMessage() method so that it outputs a message:
static void OverLimitMessage(int claimNumber, double value){Console.WriteLine($"*** Claim {claimNumber} for {value} needs to be verified***");} Call the ReadAndCheckRepairClaims () method from the RepairClaimCheckerLogic class:
myRepairClaimCheckerLogic.ReadAndCheckRepairClaims();
Lab 1: Possible Solution with output shown in Figure 25-41

A window has 2 lines of text. The first line reads, asterisk symbol, number greater than 9 detected, asterisk symbol.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-42

A window has 8 lines of text which include the questions about what the repair claim amount is. The last 2 lines indicate that claims have to be verified.
Lab 2 output
Chapter 23 Labs: Generics
Lab 1
- Have a class called Calculator, which accepts any type. It is generic <T> and this class will
- Have a method called AddTwoValues() , which has two parameters. The first parameter is called valueOne of type T and the second parameter is called valueTwo of type T, and inside the method
There is a variable called firstValue, which is of data type dynamic, and it is assigned to valueOne.
There is a variable called secondValue, which is of data type dynamic, and it is assigned to valueTwo.
The answer variable is assigned the “sum” of firstValue and secondValue (firstValue + secondValue).
The method is a value method and it returns the variable answer.
- Have a class called CalculatorApplicaton and inside it
- A Main() method that
Instantiates the Calculator class with type <int>, naming the instantiation intCalculator
Then calls the AddTwoValues() method, passing it the values 80 and 20, and writes the returned value to the console
Instantiates the Calculator class with type <string>, naming the instantiation stringCalculator
Then calls the AddTwoValues() method, passing it the values “Gerry” and “Byrne”, and writes the returned value to the console
Repeat the instantiation and method call for float and double data types.
Lab 2
- Have a class called ClaimLogic and inside it
- There will be a method to create an ArrayList with the following values:"POL1234", 2000.99, "POL1235", 3000.01, "POL1236", 599.99, "POL1237", 399.01, "POL1238", 9000, "POL1239"
Then this ArrayList is passed to the next method.
A method is created that accepts the ArrayList and iterates the values, and for each value it passes the value to another method that is generic and accepts any value type. The generic method then returns true if the value passed to it is an int or a double and false if it is any other type.
When the value returned to the iteration is Boolean true, add the value to an accumulated total of the claims, and increment a number of valid claims variable by 1.
When the value returned to the iteration is Boolean false, increment a number of policy ids variable by 1.
Finally, display the total of the claims, the number of claims, and the number of policy ids.
- Have a class called CompareClaims and inside it
Have a Main() method that calls the method that creates the ArrayList.
Lab 1: Possible Solution with output shown in Figure 25-43
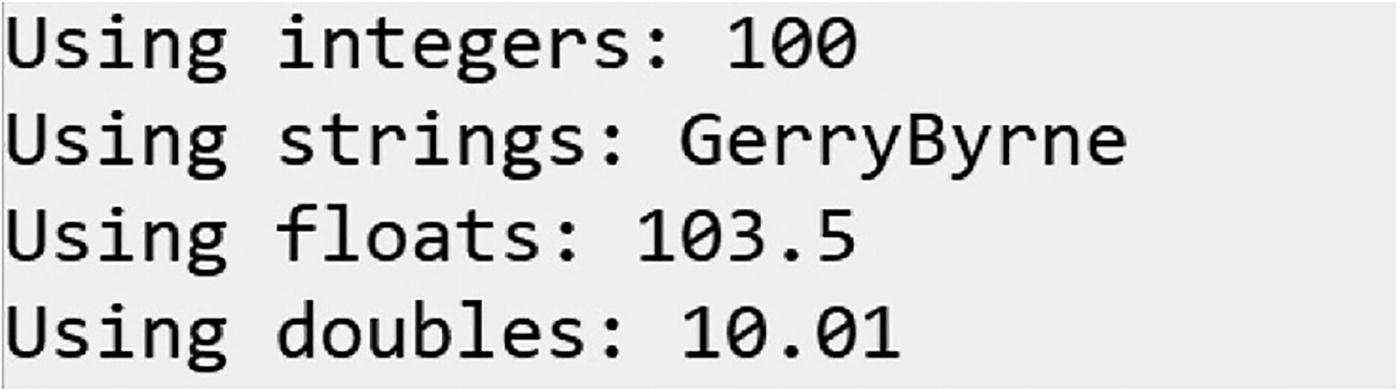
A window has 4 lines of text. The text mention using integers, strings, floats, and doubles, as well as their corresponding values.
Lab 1 output
Lab 2: Possible Solution with output shown in Figure 25-44

A window has 3 lines of text where line 1 reads, there were 5 claims. Lines 2 and 3 indicate the total claims amount and the number of policies.
Lab 2 output
Chapter Summary
Well, that was a lot of coding, and hopefully we tried to use our own learnings and code style rather than just looking at the basic solutions given for the labs.
As we finish this penultimate chapter, we can say we have achieved so much. We should be immensely proud of the learning to date. We are getting so close to our target we can almost touch it, but we have just one more small step to take.

An illustration of concentric circles with a text below that reads, our target is getting closer. The two circles at the center are shaded in green.