The HC-SR04 is a cheap ultrasonic sensor. It is used to measure the range between itself and an object. Each HC-SR04 module includes an ultrasonic transmitter, a receiver, and a control circuit. You can see that the HC-SR04 has four pins: GND, VCC, Triger, and Echo. You can buy HC-SR04 from SparkFun, at https://www.sparkfun.com/products/13959, as shown in the next image. You can also find this sensor at SeeedStudio: https://www.seeedstudio.com/Ultra-Sonic-range-measurement-module-p-626.html. To save money, you can buy the HC-SR04 sensor from Aliexpress.

The HR-SR04 has four pins. There are VCC, GND, Echo, and Trigger. We can use it with Arduino, Raspberry Pi, or other IoT boards.
For demonstration, we can develop a simple application to access the HC-SR04 on an Arduino board. You can do the following wiring:
- HC-SR04 VCC is connected to Arduino 5V
- HC-SR04 GND is connected to Arduino GND
- HC-SR04 Echo is connected to Arduino Digital 2
- HC-SR04 Trigger is connected to Arduino Digital 4
You can see the hardware wiring in the following figure:
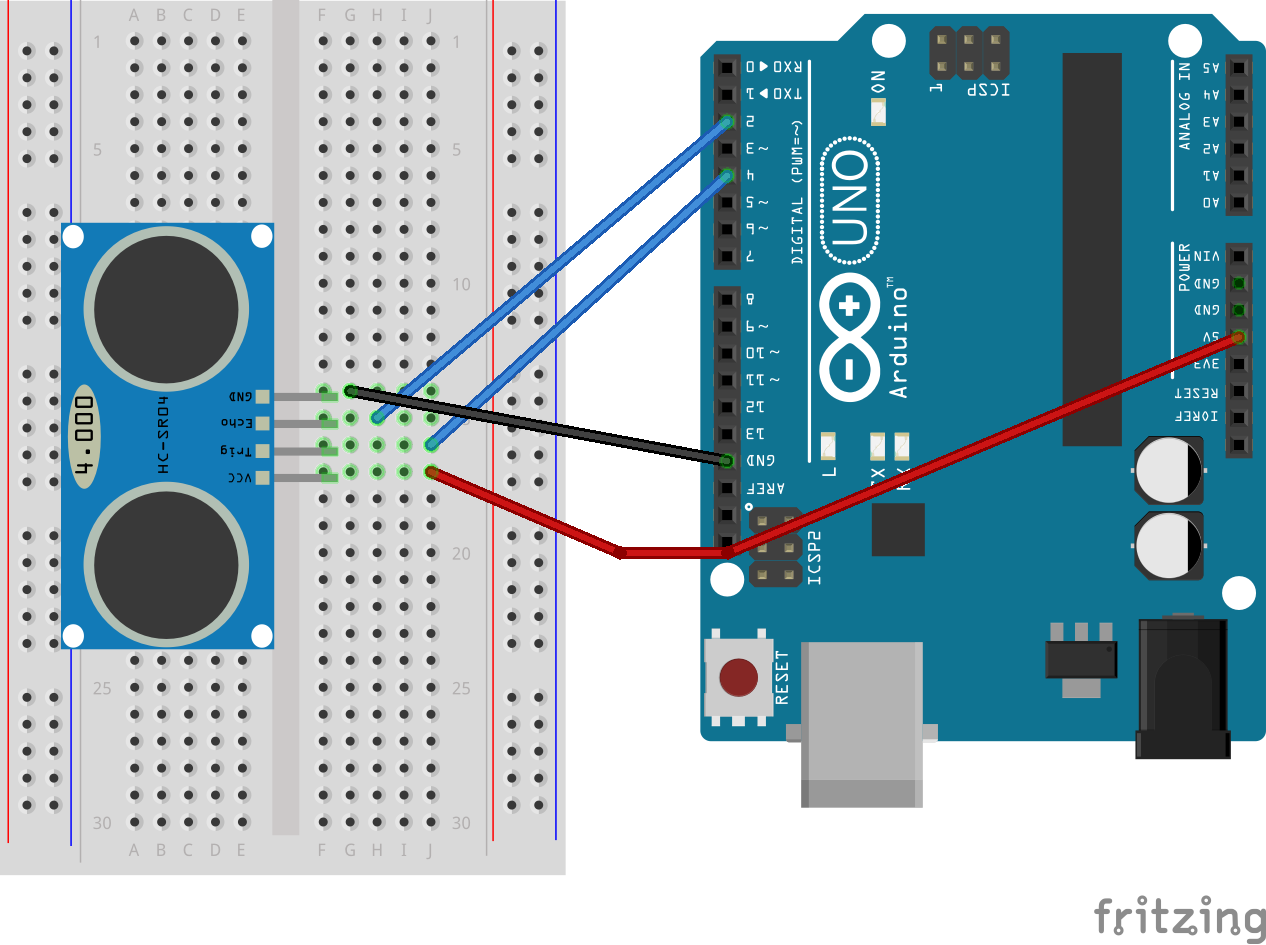
To work with the HC-SR04 module, we can use the NewPing library on our Sketch program. You can download it from http://playground.arduino.cc/Code/NewPing and then deploy it into the Arduino libraries folder. After it is deployed, you can start writing your Sketch program.
Now you can open your Arduino software. Then write the following complete code for our Sketch program:
#include <NewPing.h> #define TRIGGER_PIN 2 #define ECHO_PIN 4 #define MAX_DISTANCE 600 NewPing sonar(TRIGGER_PIN, ECHO_PIN, MAX_DISTANCE); long duration, distance; void setup() { pinMode(13, OUTPUT); pinMode(TRIGGER_PIN, OUTPUT); pinMode(ECHO_PIN, INPUT); Serial.begin(9600); } void loop() { digitalWrite(TRIGGER_PIN, LOW); delayMicroseconds(2); digitalWrite(TRIGGER_PIN, HIGH); delayMicroseconds(10); digitalWrite(TRIGGER_PIN, LOW); duration = pulseIn(ECHO_PIN, HIGH); //Calculate the distance (in cm) based on the speed of sound. distance = duration/58.2; Serial.print("Distance="); Serial.println(distance); delay(200); }
Save this sketch as ArduinoHCSR04.
This program will initialize a serial port at baudrate 9600 and activate digital pins for the HC-SR04 in the setup() function. In the loop() function, we read the value of the distance from the sensor and then display it on the serial port.
You can compile and upload this sketch to your Arduino board. Then you can open the Serial Monitor tool from the Arduino IDE menu. Make sure you set your baudrate to 9600.