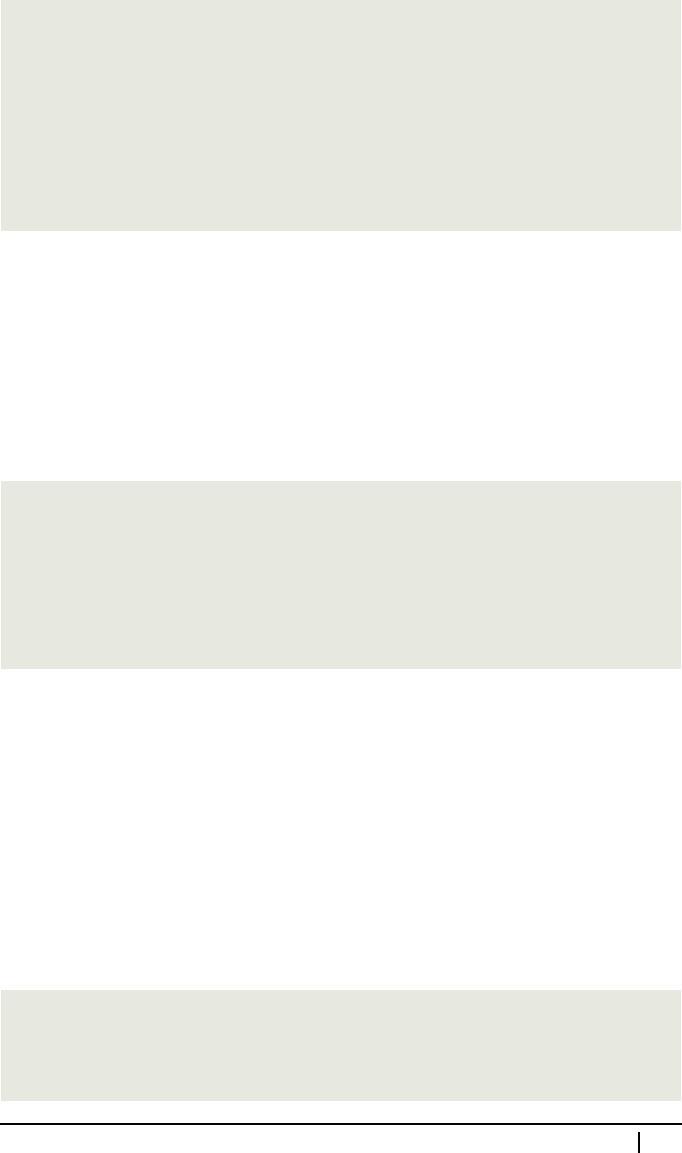
Maria: Exactly. Precisely this observation led us to the conclusion that we were
actually computing the remainder of a division. So we tried the following so lution:
var a = 63, b = 98;
while (a != b) {
if (a > b) {
a %= b;
}
if (a < b) {
b %= a;
}
}
document.write("GCD is " + a);
But u nfortunately it doesn’t work.
Professor: Let’s take one more look at the sequence of values pr oduced by your first
progr am. In the third line of the Console, the value of a is exactly four times that of b.
The statement a %= b; will therefore make a equal zero. Because a is now smaller
than b, the statement b %= a; is executed next. Since the remainder of division by
zero is not defined, b becomes NaN. End of story.
If you want to use the rem ainder operator, this is a possible solution:
var a = 63, b = 98;
var tmp;
while (b != 0) {
tmp = b;
b = a % b;
a = tmp;
}
document.write("GCD is " + a);
The trick is that you copy the old value of b to a with help fr om a temporary variable
tmp. Meanwhile, you use the old value of a to co mpute the remainder and assign it to
b. If you do that, a is always greater than b at the end of each iteration. Notice also
the changed loop condition, which now com pares b to zero.
Interesting in this last solu tion is that the first iteration of while just swaps the values
of both variables if the run starts off with b greater than a.
Mike: By the way, we also extended the multiplication pr ogram you gave us on
page 135 to work with negative integers. First we computed the sign of the prod-
uct and absolute values of multiplicands, and in the end we multiplied the product
with the sign:
var product = 0, x = 6, y = 8;
var sign = x * y >= 0 ? 1 : -1;
x = x >= 0 ? x : -x;
y = y >= 0 ? y : -y;
8.1. Homework Discussion 149