In addition to the manifest permissions, Android needs explicit runtime permissions for Bluetooth and Coarse Location. Irrespective of the application flow, these permissions should be asked at the start of the application lifetime. To get the coarse location permission, you can add:
if (this.checkSelfPermission(android.Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) { requestPermissions(new String[]{android.Manifest.permission.ACCESS_COARSE_LOCATION}, REQUEST_CODE_COARSE_PERMISSION);
}
The method checkSelfPermission confirms if the location permission is not already given. On the other hand, the REQUEST_CODE_COARSE_PERMISSIONis a static integer which can be caught by overriding onRequestPermissionResult()method:
@Override public void onRequestPermissionsResult(int requestCode, String[] permissions, int[] grantResults) { super.onRequestPermissionsResult(requestCode, permissions, grantResults);
if(requestCode == REQUEST_CODE_COARSE_PERMISSION){ Toast.makeText(this, "Coarse Permission Granted...", Toast.LENGTH_LONG).show(); }
}
The UI dialog for location permission will look like this:
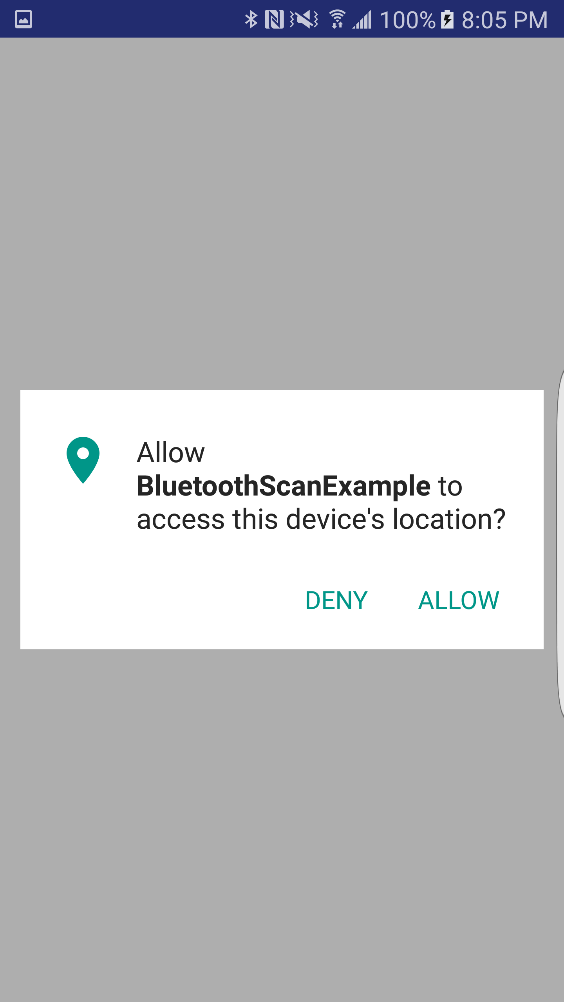
Following the location permission, the next permission is the Bluetooth permission. It is the most important permission and for all the Android examples in this book. In order to give the permission, you need to add following code:
if (!mBluetoothAdapter.isEnabled()){
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_CODE_BLUETOOTH_PERMISSION);
}
mBluetoothAdapter is a global instance of the class BluetoothAdapter. isEnabled() method tells if the Bluetooth is enabled in the cellphone. If the Bluetooth is not enabled, we can call a pre-defined intent by using ACTION_REQUEST_ENABLE. All the startActivityForResult() calls are returned back to the activity and can be caught in onActivityResult();
@Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { super.onActivityResult(requestCode, resultCode, data); if(requestCode == REQUEST_CODE_BLUETOOTH_PERMISSION){ // Do something }
}
The Bluetooth permission dialog will look like this:
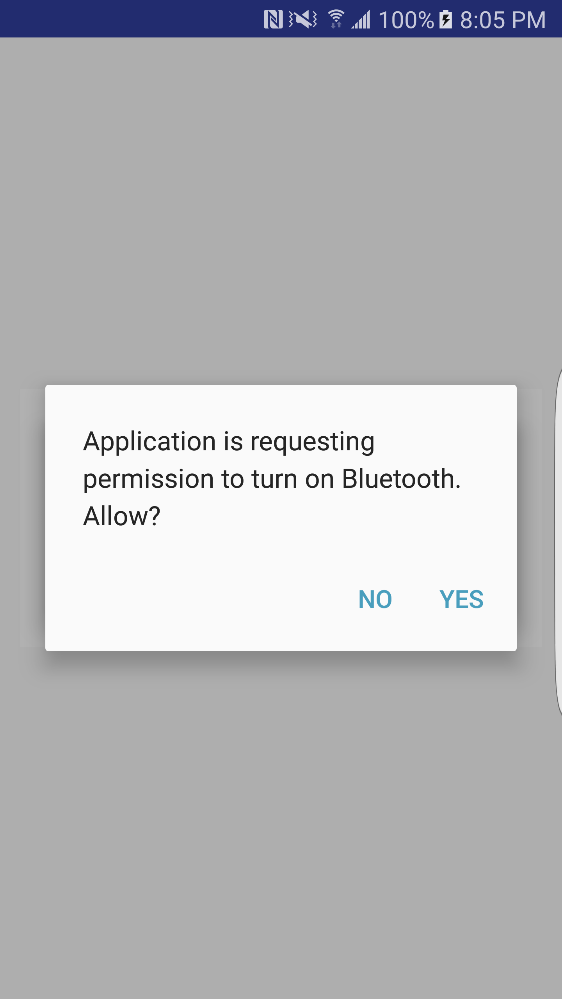
Once these runtime permissions are given, the next step is to start the scanning process.