Creating a command-line interface is not always easy. In C/C++, you need to start parsing arguments and then decide which flags are set and whether they comply with all the conditions. This is a non-issue in Rust thanks to Command-Line Argument Parser (CLAP). The CLAP crate, enables us to create very complex command-line interfaces with just a bit of code.
Not only that; it will create the help menus for us and it will also be maintainable since it will be easy to add or remove parameters and flags. It will ensure that the input we receive is valid and it will even create command-line completion scripts for the most-used shells.
You can generate the complete CLI with macros, but I personally prefer to use simple Rust code. It has a few helper macros, though, to gather some information. Remember to add clap to your Cargo.toml file and let's see how we would create a simple command-line interface:
#[macro_use]
extern crate clap;
use clap::{App, Arg};
fn main() {
let matches = App::new(crate_name!())
.version(crate_version!())
.about(crate_description!())
.author(crate_authors!())
.arg(
Arg::with_name("user")
.help("The user to say hello to")
.value_name("username")
.short("u")
.long("username")
.required(true)
.takes_value(true)
)
.get_matches();
let user = matches.value_of("user")
.expect("somehow the user did not give the username");
println!("Hello, {}", user);
}
As you can see, we defined a CLI with the crate name, description, version, and authors, which will be taken from the Cargo.toml file at compile time so that we do not need to update it for every change. It then defines a required user argument, which takes a value and uses it to print the value. The expect() here is safe because clap makes sure that the argument is provided, since we asked it to with required(true). If we simply execute cargo run, we will see the following error:

It tells us that it needs the username parameter and points us to the --help flag, automatically added by clap along with the -V flag, to show the crate version information. If we run it with cargo run -- --help, we will see the help output. Note that any argument to cargo after a double dash will be passed as an argument to the executable. Let's check it:
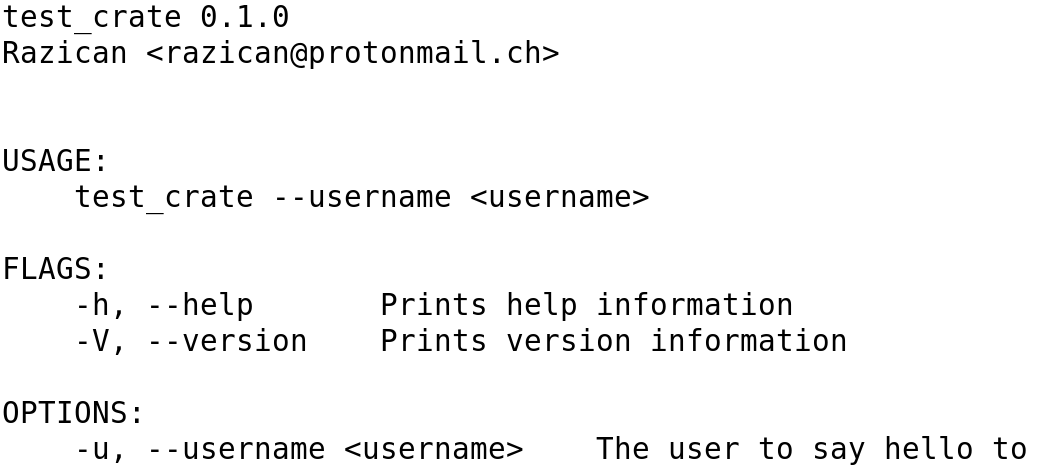
As we can see, it shows really well-formatted help text. If we want to actually see the result of passing a proper username, we can execute it with cargo run -- -u {username}:
