We saw with the Send and Sync traits that the first one allows for a variable to be sent between threads, but how does that work? Can we just use a variable created in the main thread inside our secondary thread? Let's try it:
use std::thread;
fn main() {
let my_vec = vec![10, 33, 54];
let handle = thread::Builder::new()
.name("my thread".to_owned())
.spawn(|| {
println!("This is my vector: {:?}", my_vec);
})
.expect("could not create the thread");
if handle.join().is_err() {
println!("Something bad happened :(");
}
}
What we did was create a vector outside the thread and then use it from inside. But it seems it does not work. Let's see what the compiler tells us:
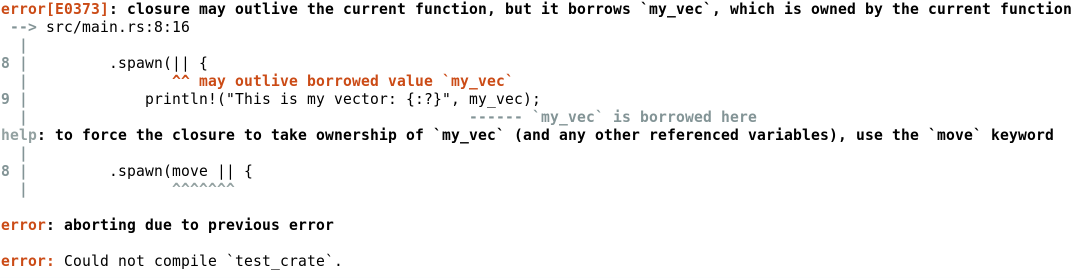
That's interesting. The compiler noticed that the my_vec binding would be dropped at the end of the main() function, and that the inner thread could live longer. This is not the case in our example, since we join() both threads before the end of the main() function, but it could happen in a scenario where a thread is creating more threads and then ending itself. This would make the reference inside the thread invalid, and Rust does not allow that.