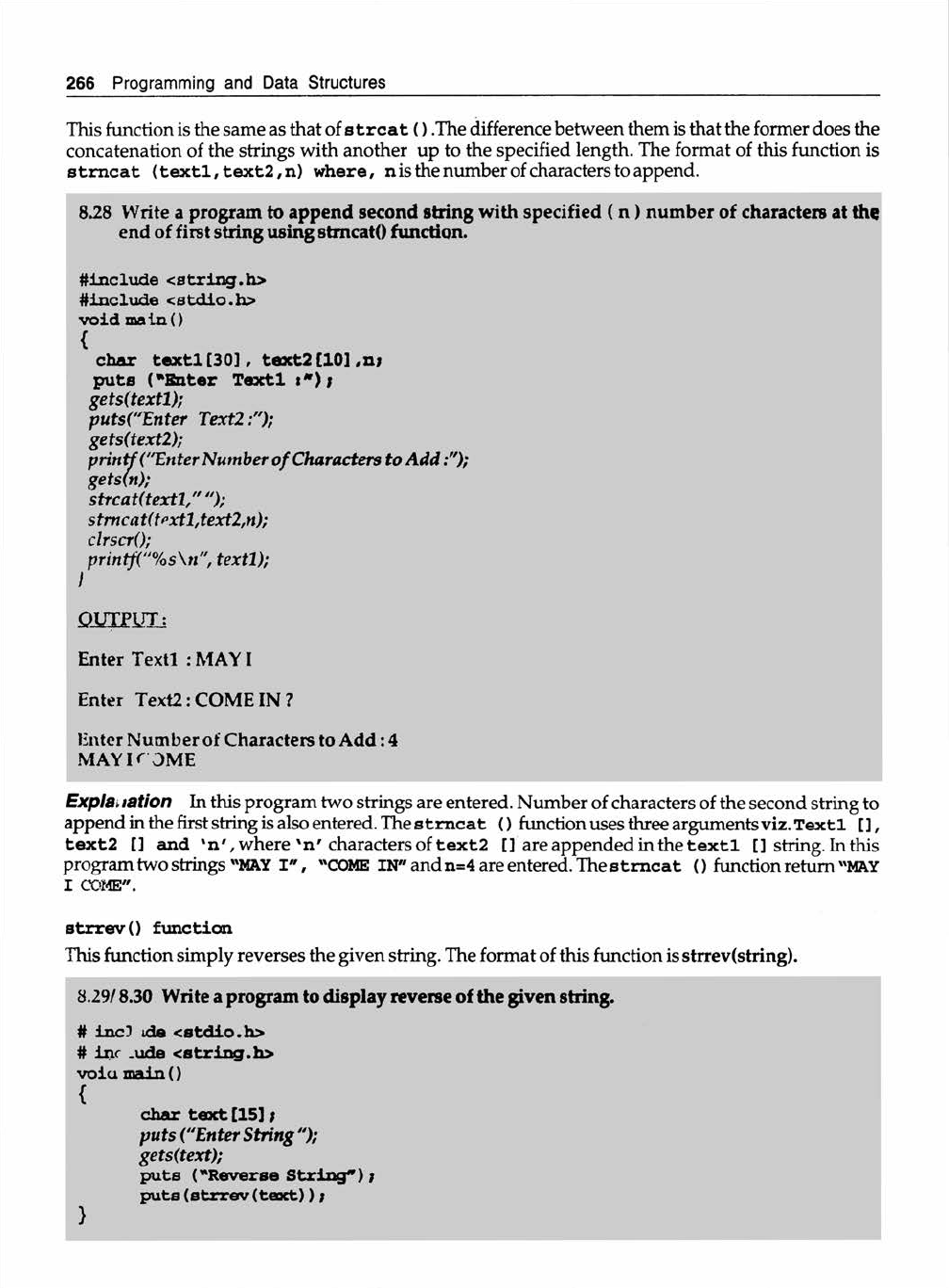
266 Programming and Data Structures
This function is the same as that of s treat () .The difference between them is that the former does the
concatenation of the strings with another up to the specified length. The format of this function is
stracat (textl, text2,n) where, n is the number of characters to append.
8.28 Write a program to append second string with specified ( n ) number of characters at th*
end of first string using stmcatO function.
#include <string.h>
#include <stdio.h>
void mainO
{
char textl [30], text2 [10] ,n;
puts (*Bnter Textl i* ) j
gets(textl);
puts("Enter Text2:");
gets(text2);
printf ("Enter Number of Characters to A d d :");
getsfn);
strcatftextl," " );
stmcatf textl,text2,n);
clrscrO;
prin tf("% sn", textl);
)
OUTPUT:
Enter Textl : M AY I
Enter Text2: COME IN ?
Enter Number of Characters to A d d : 4
M A Y K O M E
Expla mtion In this program two strings are entered. Number of characters of the second string to
append in the first string is also entered. The stmcat () function uses three arguments viz. Textl [],
text2 [] and 'n ', wh ere' n ' characters of text 2 [] are appended in the te xtl [] string. In this
program two strings "MAY I " , "COME IN" and n=4 are entered. The stmcat () function return "MAY
I COME".
strrevO function
This function simply reverses the given string. The format of this function is strrev(string).
8.29/ 8.30 Write a program to display reverse of the given string.
# inc3 ids <stdio.h>
# inc .ude <string.h>
voia main ()
{
char text [15];
puts ("Enter String “);
gets(text);
puts ("Reverse String") /
puts (strrev(text));
}