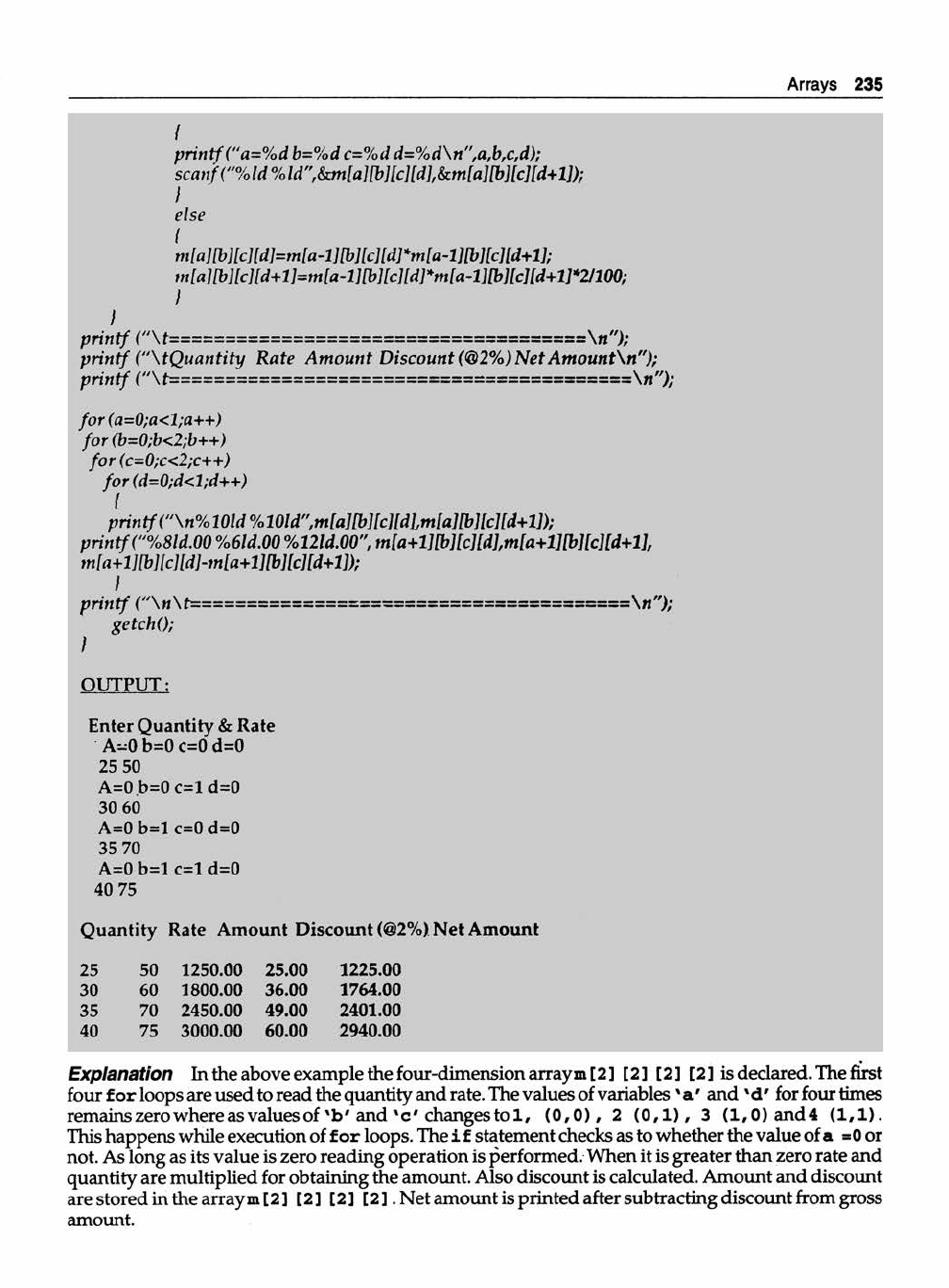
Arrays 235
I
printf ("a =% d b=%d c~%d d=% dn”,a,b,c,d);
scanf ("%ld%ld",& an[a][b][c][d],& cm [a][b][c][d+l]);
I
else
I
m [a ]M c ][d ]^ m [a -l ]M c ]ld ]* m [a -l ]M c ][d ^ V ;
m [a ][b ][c][d + l]= m [a -l][b][c ]ld ]*m [a -l][b ][c ][d +l]*2 ll0 0 ;
)
f
printf (//f=====================================n");
printf ("tQ uantity Rate Amount Discount (@ 2 % )Net Am oun tn");
printf ('//f============================i=============
t//);
for (a=0;a<l;a++)
for (b=0;b<2;b++)
fo r (c=0;c<2;c++)
fo r (d=0;d<l;d++)
I
printf ("n % 1 0ld % 1 0 ld ",m [a ][b][c ][dLm [a ][b ][c][d+l]);
printf ("%8ld.00 %6ld.00 %12ld.00”, m [a + l][b ][c ][d ],m [a + l][b ][c ][d + l],
m [a + l][b ][c ][d ]-tn [a + l][b ][c ][d + l]);
I
printf (//«^===============“ =====================w");
getchO;
I
OUTPUT:
Enter Quantity & Rate
A-0 b=0 c=0 d=0
25 50
A=0.b=0 c=l d=0
30 60
A=0 b=l c=0 d=0
35 70
A=0 b=l c=l d=0
40 75
Quantity Rate Amount Discount (@2%) Net Amount
25 50 1250.00 25.00 1225.00
30 60 1800.00 36.00 1764.00
35 70 2450.00 49.00 2401.00
40 75 3000.00 60.00 2940.00
Explanation In the above example the four-dimension arraym [2] [2] [2] [2] is declared. The first
four fo r loops are used to read the quantity and rate. The values of variables 'a ' and 'd # forfourtimes
remains zero where as values of 'b ' and xc # changes to 1, (0 ,0 ), 2 (0 ,1 ), 3 (l,0 )a n d 4 (1,1).
This happens while execution of fo r loops. The i f statement checks as to whether the value of a = 0 or
not. As long as its value is zero reading operation is performed. When it is greater than zero rate and
quantity are multiplied for obtaining the amount. Also discount is calculated. Amount and discount
are stored in the array m [2] [2] [2] [2] .Net amount is printed after subtracting discount from gross
amount.