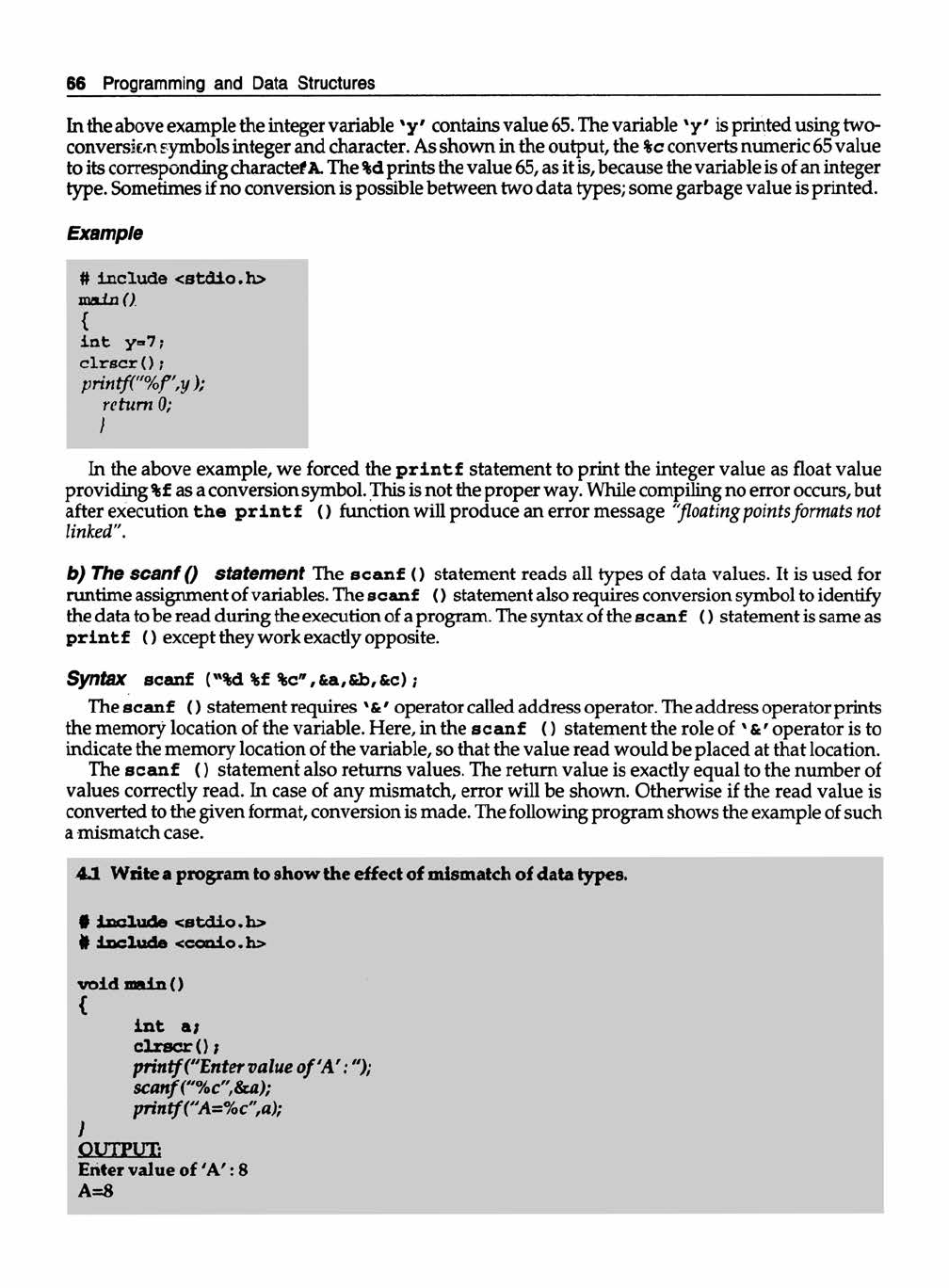
66 Programming and Data Structures
In the above example the integer variable 'y ' contains value 65. The variable 'y ' is printed using two-
conversic-n symbols integer and character. As shown in the output, the %c converts numeric 65 value
to its corresponding character A. The %d prints the value 65, as it is, because the variable is of an integer
type. Sometimes if no conversion is possible between two data types; some garbage value is printed.
Example
# include <stdio.h>
main (7
{
in t y« 7 ;
c lr s c r ( );
printf("%f',y );
return 0;
I
In the above example, we forced the p r in tf statement to print the integer value as float value
providing %f as a conversion symbol. This is not the proper way. While compiling no error occurs, but
after execution th e p r i n t f () function will produce an error message "floating points formats not
linked
b) The scant () statem ent The scan f () statement reads all types of data values. It is used for
runtime assignment of variables. The scanf () statement also requires conversion symbol to identify
the data to be read during the execution of a program. The syntax of the scanf () statement is same as
p rin tf () except they work exactly opposite.
Syntax scanf ("%d %f He” , &a,&b,&c);
The scanf () statement requires ' &' operator called address operator. The address operator prints
the memory location of the variable. Here, in the sc a n f () statement the role of ' &' operator is to
indicate the memory location of the variable, so that the value read would be placed at that location.
The scan f () statement also returns values. The return value is exactly equal to the number of
values correctly read. In case of any mismatch, error will be shown. Otherwise if the read value is
converted to the given format, conversion is made. The following program shows the example of such
a mismatch case.
4JL Write a program to show the effect of mismatch of data types.
9 include <stdio.h>
# include cconio. h>
void main ()
{
in t a ;
c lrs c r () ;
printf ("Enter value of'A ':");
scanf ("%c",&a);
printf ("A=%c"fa);
I
OUTPUT:
Enter value of 'A ': 8
A=8