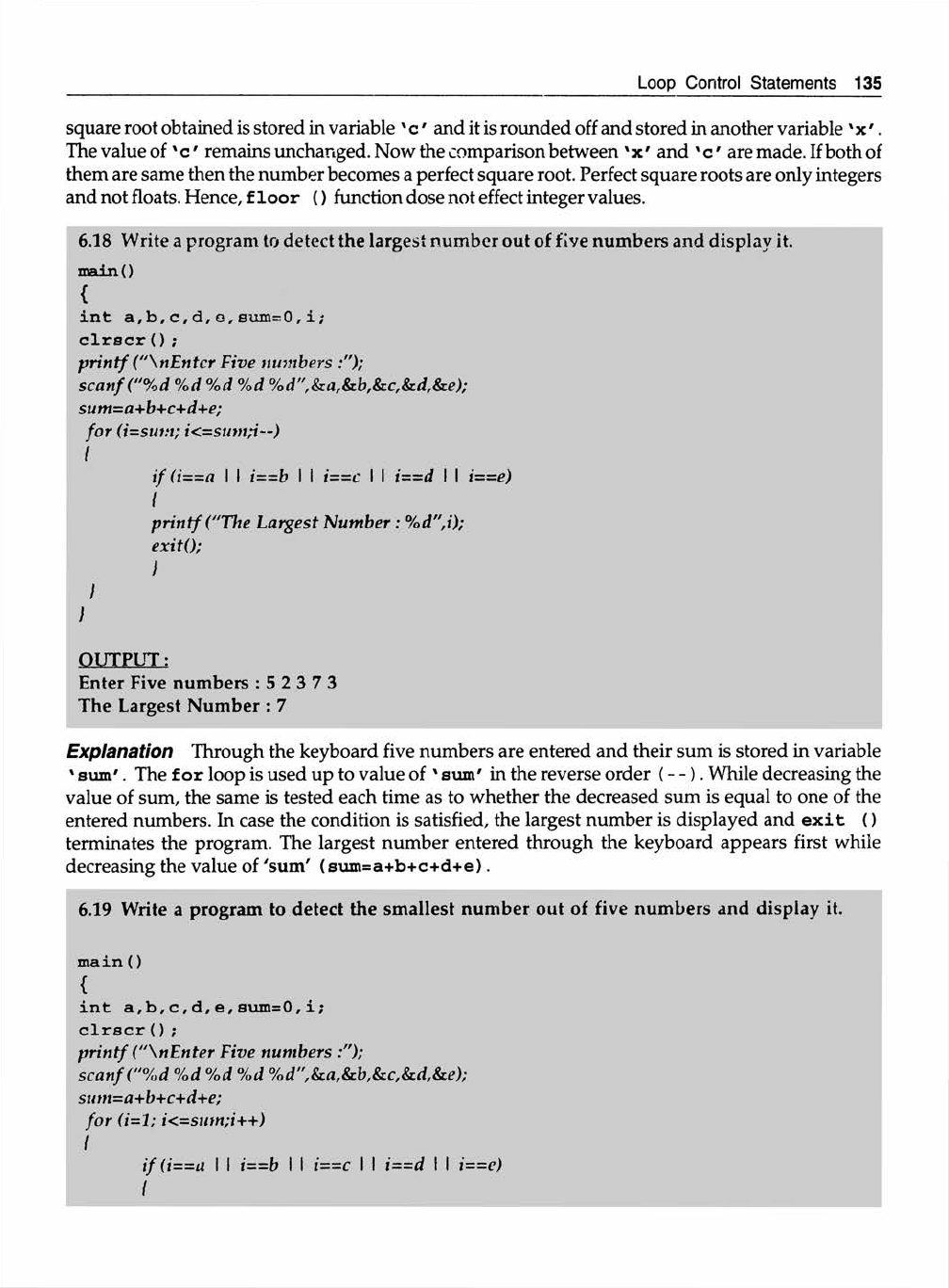
Loop Control Statements 135
square root obtained is stored in variable ' c ' and it is rounded off and stored in another variable ' x '.
The value of xc ' remains unchanged. Now the comparison between 'x ' and * c' are made. If both of
them are same then the number becomes a perfect square root. Perfect square roots are only integers
and not floats. Hence, f lo o r () function dose not effect integer values.
6.18 Write a program to detect the largest number out of five numbers and display it.
main()
{
i n t a,b,c,d,o ,sum=0,i;
clrsc r() ;
printf ("nEntcr Five numbers
scanf ("%d %d %d %d %d",&za,8zb,&i:c,&cd,&Le);
sum=a+b+c+d+e;
for (i=sutn; i<=sutnrf~)
{
if (i==a I I i==b I I i==c I I i==d I I i==e)
{
printf ("The Largest Number: %d”,i);
exit();
I
I
OUTPUT:
Enter Five numbers : 5 2 3 7 3
The Largest Number : 7
Explanation Through the keyboard five numbers are entered and their sum is stored in variable
% sum '. The f o r loop is used up to value of ' sum' in the reverse order (- - ) . While decreasing the
value of sum, the same is tested each time as to whether the decreased sum is equal to one of the
entered numbers. In case the condition is satisfied, the largest number is displayed and e x it ()
terminates the program. The largest number entered through the keyboard appears first while
decreasing the value of 'sum' (sum =a+b+c+d+e).
6.19 Write a program to detect the smallest number out of five numbers and display it.
main ()
{
in t a ,b ,c ,d ,e ,s u m = 0 ,i;
c l r s c r ( ) ;
printf ("nEnter Five numbers
scanf ("%d %d %d %d
sum=a+b+c+d+e;
for (i=l; i<=sum;i++)
I
if (i==a I I i~ b I I i==c I I i==d I I i==e)
I