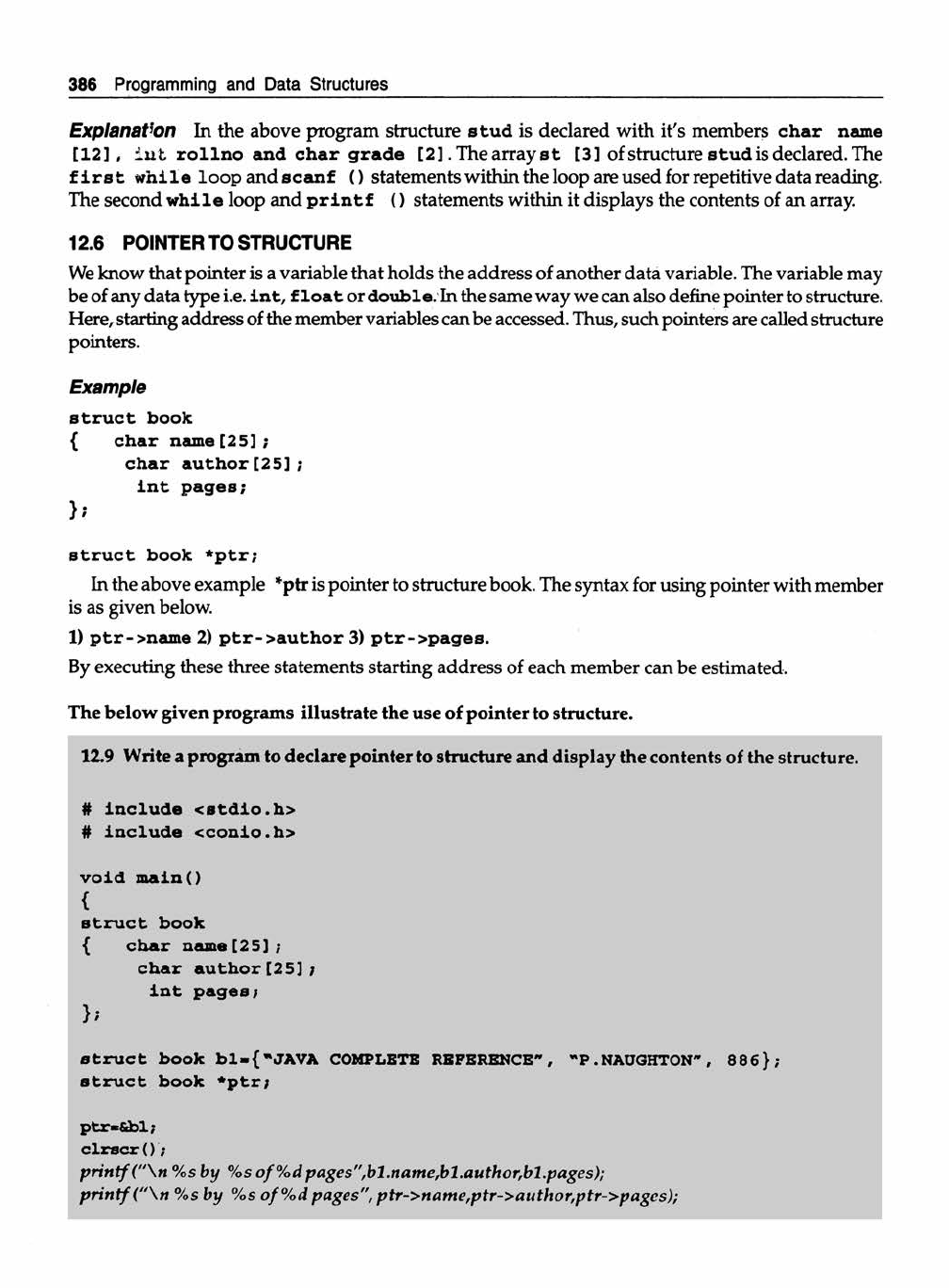
386 Programming and Data Structures
Explanation In the above program structure stud is declared with it's members char name
[1 2], in t ro lln o and char grade [2 ]. The array st [3] of structure stud is declared. The
f i r s t while loop and scanf () statements within the loop are used for repetitive data reading.
The second w hile loop and p rin tf () statements within it displays the contents of an array.
12.6 POINTER TO STRUCTURE
We know that pointer is a variable that holds the address of another data variable. The variable may
be of any data type i.e. int, flo a t or double. In the same way we can also define pointer to structure.
Here, starting address of the member variables can be accessed. Thus, such pointers are called structure
pointers.
Exam ple
struct book
{ char name[25 ];
char author[2 5 ];
in t pages;
j*
struct book *p tr;
In the above example *ptr is pointer to structure book. The syntax for using pointer with member
is as given below.
1) ptr->name 2) ptr->author 3) p tr- >pages.
By executing these three statements starting address of each member can be estimated.
The below given programs illustrate the use of pointer to structure.
12.9 Write a program to declare pointer to structure and display the contents of the structure.
# include <stdio.h >
# include <conio.h>
void main()
{
struct book
{ char name[2 5];
char autho r[2 5 ];
in t pages;
} ;
stru ct book b l-{"JA V A COMPLETE REFERENCE", "P .NAUGHTON", 886};
struct book *p tr;
ptr*&bl;
clrscr ();
printf ("n %s by %s of %dpages",bl.name,bl.author,bl.pages);
printf (" n %s by %s o f% d pages", ptr->name,ptr->author,ptr->pages);