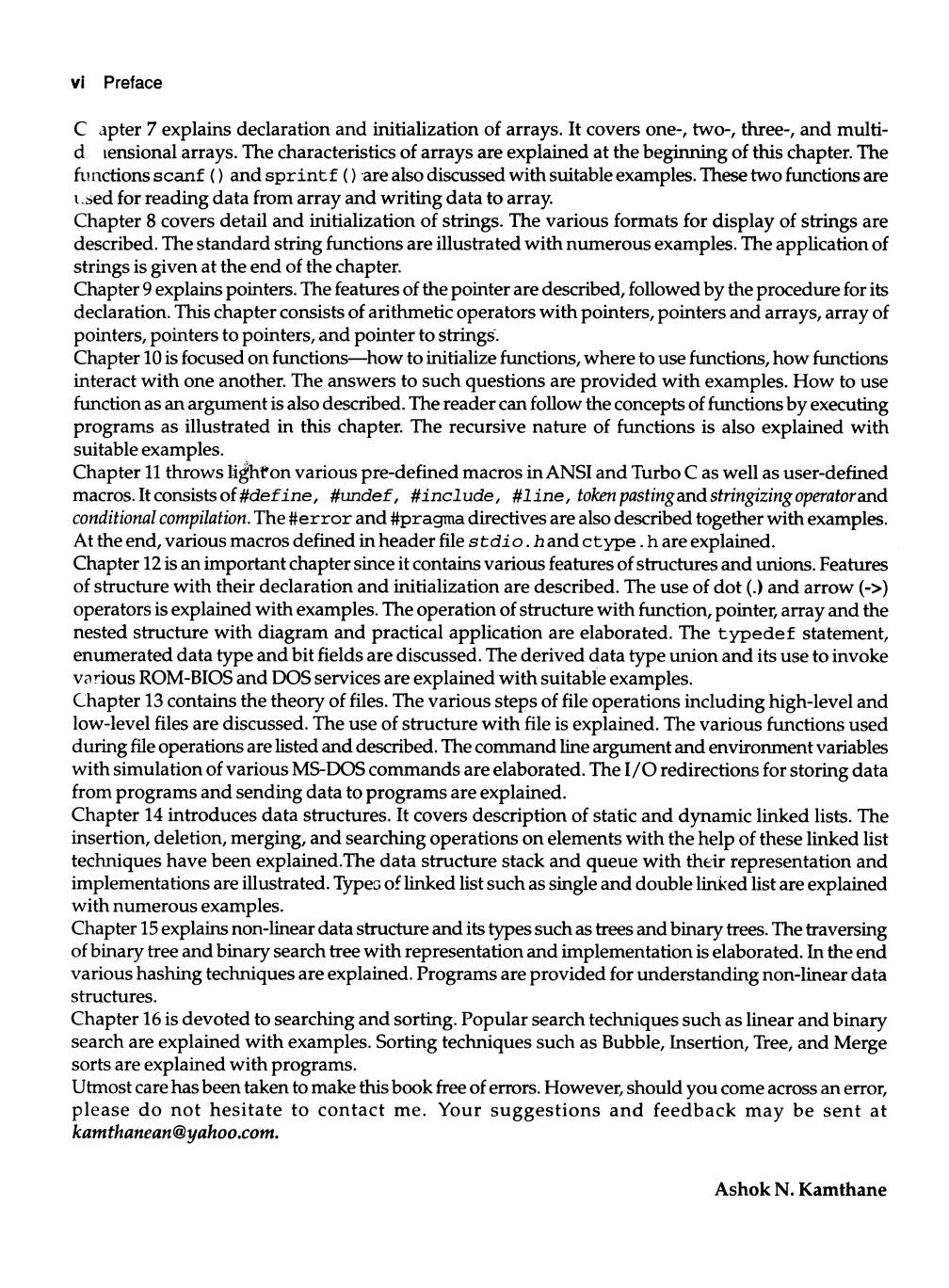
vi Preface
C apter 7 explains declaration and initialization of arrays. It covers one-, two-, three-, and multi-
d tensional arrays. The characteristics of arrays are explained at the beginning of this chapter. The
functions scanf () and sprint f () are also discussed with suitable examples. These two functions are
used for reading data from array and writing data to array.
Chapter 8 covers detail and initialization of strings. The various formats for display of strings are
described. The standard string functions are illustrated with numerous examples. The application of
strings is given at the end of the chapter.
Chapter 9 explains pointers. The features of the pointer are described, followed by the procedure for its
declaration. This chapter consists of arithmetic operators with pointers, pointers and arrays, array of
pointers, pointers to pointers, and pointer to strings.
Chapter 10 is focused on functions—how to initialize functions, where to use functions, how functions
interact with one another. The answers to such questions are provided with examples. How to use
function as an argument is also described. The reader can follow the concepts of functions by executing
programs as illustrated in this chapter. The recursive nature of functions is also explained with
suitable examples.
Chapter 11 throws lijfhf on various pre-defined macros in ANSI and Turbo C as well as user-defined
macros. It consists of # defin e, #undef, # in clu de, # lin e, token pasting and stringizing operator and
conditional compilation. The #error and #pragma directives are also described together with examples.
At the end, various macros defined in header file s t d i o . h and ctyp e. h are explained.
Chapter 12 is an important chapter since it contains various features of structures and unions. Features
of structure with their declaration and initialization are described. The use of dot (.) and arrow (->)
operators is explained with examples. The operation of structure with function, pointer, array and the
nested structure with diagram and practical application are elaborated. The typedef statement,
enumerated data type and bit fields are discussed. The derived data type union and its use to invoke
various ROM-BIOS and DOS services are explained with suitable examples.
Chapter 13 contains the theory of files. The various steps of file operations including high-level and
low-level files are discussed. The use of structure with file is explained. The various functions used
during file operations are listed and described. The command line argument and environment variables
with simulation of various MS-DOS commands are elaborated. The I/O redirections for storing data
from programs and sending data to programs are explained.
Chapter 14 introduces data structures. It covers description of static and dynamic linked lists. The
insertion, deletion, merging, and searching operations on elements with the help of these linked list
techniques have been explained.The data structure stack and queue with their representation and
implementations are illustrated. Types of linked list such as single and double linked list are explained
with numerous examples.
Chapter 15 explains non-linear data structure and its types such as trees and binary trees. The traversing
of binary tree and binary search tree with representation and implementation is elaborated. In the end
various hashing techniques are explained. Programs are provided for understanding non-linear data
structures.
Chapter 16 is devoted to searching and sorting. Popular search techniques such as linear and binary
search are explained with examples. Sorting techniques such as Bubble, Insertion, Tree, and Merge
sorts are explained with programs.
Utmost care has been taken to make this book free of errors. However, should you come across an error,
please do not hesitate to contact me. Your suggestions and feedback may be sent at
kamthanean@yahoo.com.
Ashok N. Kamthane