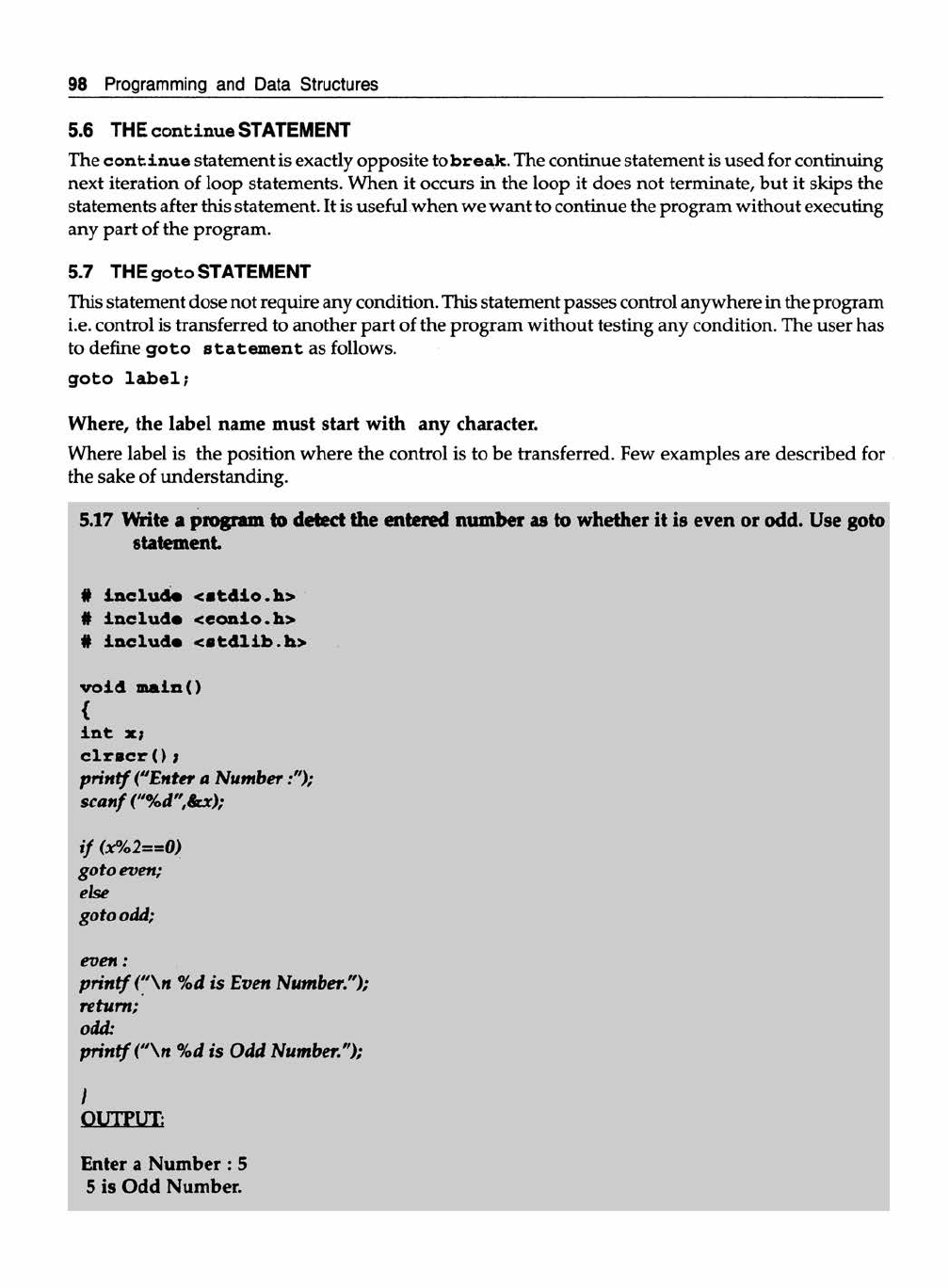
98 Programming and Data Structures
5.6 THE continue STATEMENT
The continue statement is exactly opposite tobreak. The continue statement is used for continuing
next iteration of loop statements. When it occurs in the loop it does not terminate, but it skips the
statements after this statement. It is useful when we want to continue the program without executing
any part of the program.
5.7 THE goto STATEMENT
This statement dose not require any condition. This statement passes control anywhere in the program
i.e. control is transferred to another part of the program without testing any condition. The user has
to define
goto statement as follows.
goto label;
Where, the label name must start with any character.
Where label is the position where the control is to be transferred. Few examples are described for
the sake of understanding.
5.17 Write a program to delect the entered number as to whether it is even or odd. Use goto
statement
# include <atdio.h>
# include <eonio«h>
# include <stdlib.h>
void main()
{
int x;
clrscr ();
printf ("Enter a Number
scanf ("%d",6oc);
if (x% 2~0)
goto even;
else
goto odd;
even:
printf ("
%d is Even Number/');
return;
odd:
printf ("
%d is Odd Number/');
I
OUTPUT;
Enter a Number : 5
5 is Odd Number.