This is an area where you may spend a significant amount of time. If we were following the 80-20 principal, pixel-perfect tweaks usually end up being the last 20% that takes 80% of the time to complete. Let's examine the differences between our implementation and the design and what it would take to bridge the gap:

The date needs further customization. The numeric ordinal th is missing; to accomplish this, we will need to bring in a third-party library such as moment or implement our own solution and bind it next to the date on the template:
- Update current.date to append an ordinal to it:
src/app/current-weather/current-weather.component.html
{{current.date | date:'EEEE MMM d'}}{{getOrdinal(current.date)}}
- Implement a getOrdinal function:
src/app/current-weather/current-weather.component.ts
export class CurrentWeatherComponent implements OnInit {
...
getOrdinal(date: number) {
const n = new Date(date).getDate()
return n > 0
? ['th', 'st', 'nd', 'rd'][(n > 3 && n < 21) || n % 10 > 3 ? 0 : n % 10]
: ''
}
...
}
Note that the implementation of getOrdinal boils down to a complicated one-liner that isn't very readable and is very difficult to maintain. Such functions, if critical to your business logic, should be heavily unit tested.
The temperature implementation needs to separate the digits from the unit with a <span> element, surrounded with a <p>, so the superscript style can be applied to the unit, such as <span class="unit">℉</span>, where unit is a CSS class to make it look like a superscript element.
- Implement a unit CSS class:
src/app/current-weather/current-weather.component.css
.unit {
vertical-align: super;
}
- Apply unit:
src/app/current-weather/current-weather.component.html
...
7 <div fxFlex="55%">
...
10 <div fxFlex class="right no-margin">
11 <p class="mat-display-3">{{current.temperature | number:'1.0-0'}}
12 <span class="mat-display-1 unit">℉</span>
13 </p>
We need to experiment with how much of space the forecast image should have, by tweaking the fxFlex value on line 7. Otherwise, the temperature overflows to the next line and your setting can further be affected by the size of your browser window. For example, 60% works well with a small browser window, but when maximized, it forces an overflow. However, 55% seems to satisfy both conditions:
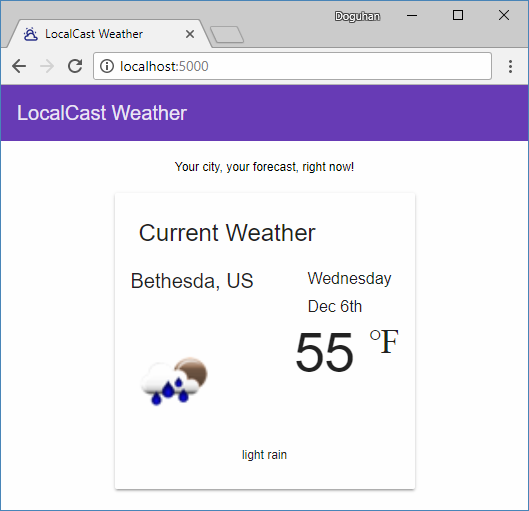
As always, it is possible to further tweak margins and paddings to further customize the design. However, each deviation from the library will have maintainability consequences down the line. Unless you're truly building a business around displaying weather data, you should defer any further optimizations to the end of the project, as time permits, and if experience is any guide, you will not be making this optimization.
With two negative margin-bottom hacks, you can attain a design fairly close to the original, but I will not include those hacks here and leave it as an exercise for the reader to discover on the GitHub repository. Such hacks are sometimes necessary evils, but in general, they point to a disconnect between design and implementation realities. The solution leading up to the tweaks section is the sweet spot, where Angular Material thrives:
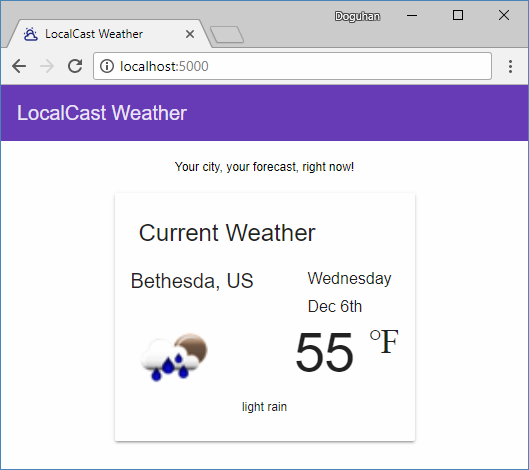