Let me restate, for the record, that console.log statements shall never be checked in to your repository. In general, they are a waste of your time, because it requires editing code and later cleaning up your code. Furthermore, Augury already provides the state of your components, so in straightforward cases, you should be able to leverage it observe or coerce state.
There are some niche use cases, where console.log statements can be useful. These are mostly asynchronous workflows that operate in parallel and are dependent on timely user interaction. In these cases, console logs can help you better understand the flow of events and interaction between various components.
Augury is not yet sophisticated enough to resolve asynchronous data or data returned via functions. There are other common cases, where you would like to observe the state of properties as they are being set, and even be able to change their values on the fly to force your code to execute branching logic in if-else or switch statements. For these cases, you should be using break point debugging.
Let's presume that some basic logic exists on HomeComponent, which sets a displayLogin boolean, based on an isAuthenticated value retrieved from an AuthService, as demonstrated:
src/app/home/home.component.ts
...
import { AuthService } from '../auth.service'
...
export class HomeComponent implements OnInit {
displayLogin = true
constructor(private authService: AuthService) {}
ngOnInit() {
this.displayLogin = !this.authService.isAuthenticated()
}
}
Now observe the state of the value of displayLogin and the isAuthenticated function as they are being set, then observe the change in the value of displayLogin:
- Click on the View Source link on HomeComponent
- Drop a break point on the first line inside the ngOnInit function
- Refresh the page
- Chrome Dev Tools will switch over to the Source tab, and you'll see your break point hit, as highlighted in blue here:
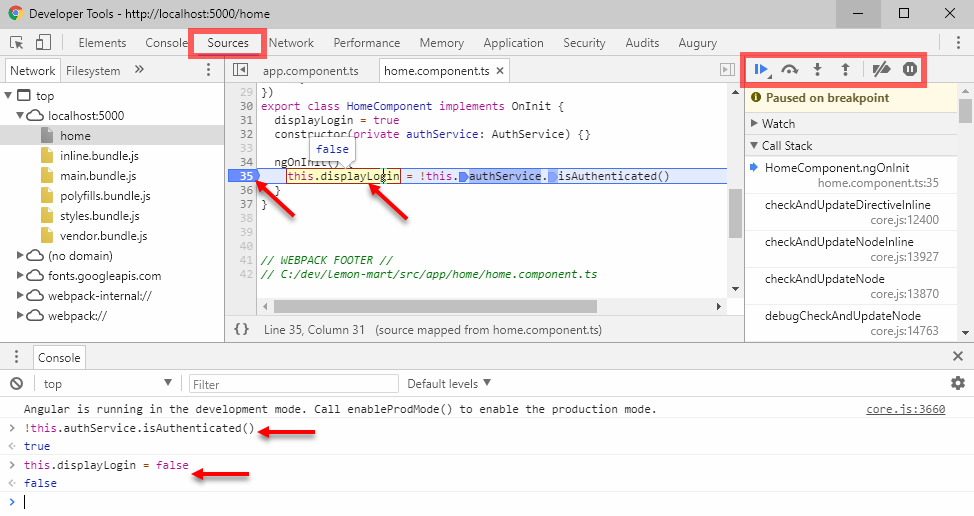
- Hover over this.displayLogin and observe that its value is set to true
- If hovering over this.authService.isAuthenticated(), you will not be able to observe its value
While your break point is hit, you can access the current scope of the state in the console, which means you can execute the function and observe its value.
- Execute isAuthenticated() in the console:
> !this.authService.isAuthenticated()
true
You'll observe that it returns true, which is what this.displayLogin is set to. You can still coerce the value of displayLogin in the console.
- Set displayLogin to false:
> this.displayLogin = false
false
If you observe the value of displayLogin, either by hovering over it or retrieving it from the control, you'll see that the value is set to false.
Leveraging break point debugging basics, you can debug complicated scenarios without changing your source code at all.