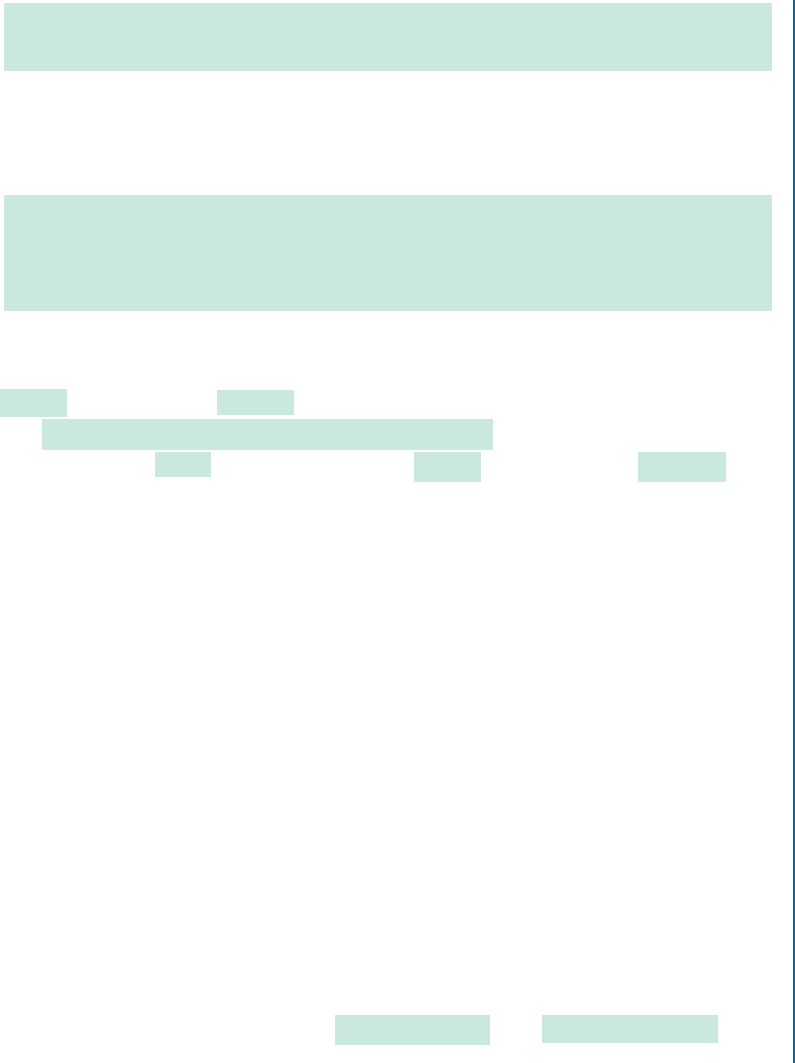
L7. LAB 7: IIR FILTERING AND ADAPTIVE FIR FILTERING 115
f=[0 0.2 0.4 0.41 1]; %frequency bands
m=[0 1 1 0 0]; %desired gain
Study the round-off error between the direct form and the second-order cascade form
using MATLAB. Use the MATLAB function tf2sos to convert the transfer function into
cascade form and then apply the filter sos as indicated below:
Z = x;
for i=1:size(sos);
Z = filter(sos(i,1:3),sos(i,4:6), Z);
end
Examine the effect of various word lengths on the output and report your observations.
Recall that you can quantize the filter by using the MATLAB fixed-point toolbox function
sfi() . For example, if coeffs denotes double precision filter coefficients, the expres-
sion ficoeffs = sfi(coeffs,bits,bits-intgr-1) can be used to convert to quan-
tized values with bits denoting wordlength, intgr integer bits, and intgr-1 frac-
tional bits. Although this issue did not arise during the exercise involving FIR coefficient
quantization, it needs to be noted that the number of integer bits must be sufficient to
accommodate IIR filter coefficients whose magnitude is greater than 1.
First, compare the frequency spectra of the filtered outputs of the direct form filter based
on the quantized and unquantized coefficients. en, compare the frequency spectra of the
filtered outputs based on the direct form quantized coefficients and the quantized second-
order sections coefficients.
2. Over time, the output of the FIR filter should converge to that of the IIR filter. Confirm
this by comparing the output of the two filters or by examining the decline in the error
term. Experiment with different step size ı and filter length N and report your observa-
tions.
Next, add a delay to the adaptive FIR filtering pipeline to make the real-time processing
fail on purpose. A possible solution to address the real-time processing aspect is to up-
date only a fraction of the coefficients using the LMS equation during each iteration. For
example, you may update all even coefficients during the first iteration, and then all odd
coefficients during the second iteration. Implement such a coefficient update scheme and
report the results in terms of the tradeoff between convergence rate, convergence accuracy,
and processing time.
Hints (Android target) – e function clock_gettime with CLOCK_MONOTONIC as the
time source can be used to obtain high-resolution timing. An implementation of this function