Let's take a test script that we have executed locally; that is, where the test scripts and the browser were on the same machine:
@BeforeClass
public void setup() {
System.setProperty("webdriver.chrome.driver",
"./src/test/resources/drivers/chromedriver");
driver = new ChromeDriver();
}
The preceding test script creates an instance of Chrome Driver and launches the Chrome browser. Now, let's try to convert this test script to use Selenium Standalone Server that we started earlier. Before we do that, let's see the constructor of RemoteWebDriver, which is as follows:
RemoteWebDriver(java.net.URL remoteAddress, Capabilities desiredCapabilities)
The input parameters for the constructor include the address (hostname or IP) of Selenium Standalone Server running on the remote machine and the desired capabilities required for running the test (for example name of the browser and/or operating system). We will see these desired capabilities shortly.
Now, let's modify the test script to use RemoteWebDriver. Replace WebDriver driver = new ChromeDriver(); with the following code:
@BeforeMethod
public void setup() throws MalformedURLException {
DesiredCapabilities caps = new DesiredCapabilities();
caps.setBrowserName("chrome");
driver = new RemoteWebDriver(new URL("http://10.172.10.1:4444/wd/hub"), caps);
driver.get("http://demo-store.seleniumacademy.com/");
}
We have created a RemoteWebDriver instance that tries to connect to http://10.172.10.1:4444/wd/hub, where Selenium Standalone Server is running and listening for requests. Having done that, we also need to specify which browser your test case should get executed on. This can be done using the DesiredCapabilities instance.
For this example, the IP used is 10.172.10.1. However, in your case, it will be different. You need to obtain the IP of the machine where the Selenium Standalone Server is running and replace the example IP used in this book.
Before running tests, we need to restart the Selenium Standalone Server by specifying the path of ChromeDriver:
java -jar -Dwebdriver.chrome.driver=chromedriver selenium-server-standalone-3.12.0.jar
Running the following test with RemoteWebDriver will launch the Chrome browser and execute your test case on it. So the modified test case will look as follows:
public class SearchTest {
WebDriver driver;
@BeforeMethod
public void setup() throws MalformedURLException {
DesiredCapabilities caps = new DesiredCapabilities();
caps.setBrowserName("chrome");
driver = new RemoteWebDriver(new URL("http://10.172.10.1:4444/wd/hub"), caps);
driver.get("http://demo-store.seleniumacademy.com/");
}
@Test
public void searchProduct() {
// find search box and enter search string
WebElement searchBox = driver.findElement(By.name("q"));
searchBox.sendKeys("Phones");
WebElement searchButton =
driver.findElement(By.className("search-button"));
searchButton.click();
assertThat(driver.getTitle())
.isEqualTo("Search results for: 'Phones'");
}
@AfterMethod
public void tearDown() {
driver.quit();
}
}
Now execute this test script from your local machine to establish a connection between the RemoteWebDriver client and Selenium Standalone Server. The Server will launch the Chrome browser. The following is the output you will see in the console where the Server is running:
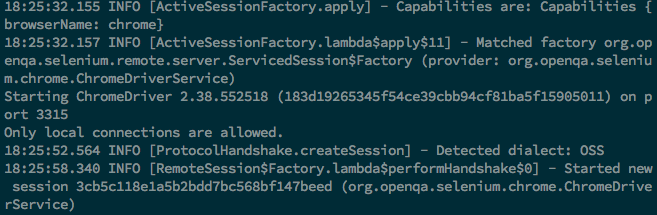
It says that a new session with the desired capabilities is being created. Once the session is established, a session ID will be printed to the console. At any point in time, you can view all of the sessions that are established with Selenium Standalone Server by navigating to the host or IP of the machine where the Selenium server is running http://<hostnameOrIP>:4444/wd/hub.
The Selenium Standalone Server, by default, listens to port number 4444. We can change the default port by passing the -port argument.
It will give the entire list of sessions that the server is currently handling. The screenshot of this is as follows:

This is a very basic portal that lets the test-script developer see all of the sessions created by the server and perform some basic operations on it, such as terminating a session, taking a screenshot of a session, loading a script to a session, and seeing all of the desired capabilities of a session. The following screenshot shows all of the default desired capabilities of our current session.
You can see the popup by hovering over the Capabilities link, as shown in the following screenshot:

Those are the default desired capabilities that are set implicitly by the server for this session. Now we have successfully established a connection between our test script, which is using a RemoteWebDriver client on one machine, and the Selenium Standalone Server on another machine. The original diagram of running the test scripts remotely is as follows:
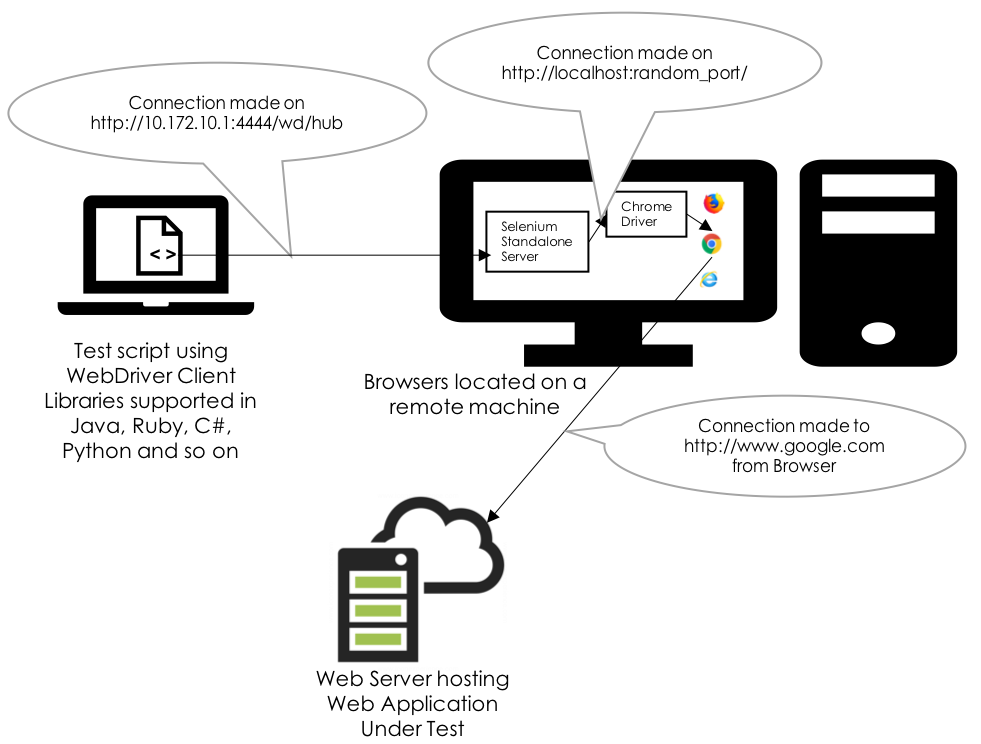