We know how to perform some basic actions, such as clicking on a button and typing text into a textbox; however, there are many scenarios where we have to perform multiple actions at the same time, for example, keeping the Shift button pressed and typing text for uppercase letters, and the dragging and dropping mouse actions.
Let's see a simple scenario here. Open the http://guidebook.seleniumacademy.com/Selectable.html. A box of tiles numbered 1 to 12 will appear, as seen in this screenshot:
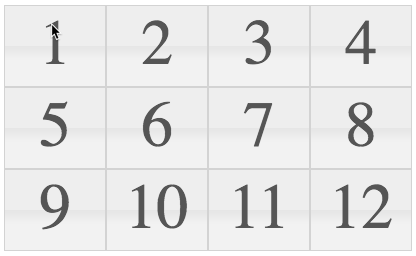
If you inspect the elements with browser developer tools, you will see an ordered list tag:
<ol id="selectable" class="ui-selectable">
<li class="ui-state-default ui-selectee" name="one">1</li>
<li class="ui-state-default ui-selectee" name="two">2</li>
<li class="ui-state-default ui-selectee" name="three">3</li>
<li class="ui-state-default ui-selectee" name="four">4</li>
<li class="ui-state-default ui-selectee" name="five">5</li>
<li class="ui-state-default ui-selectee" name="six">6</li>
<li class="ui-state-default ui-selectee" name="seven">7</li>
<li class="ui-state-default ui-selectee" name="eight">8</li>
<li class="ui-state-default ui-selectee" name="nine">9</li>
<li class="ui-state-default ui-selectee" name="ten">10</li>
<li class="ui-state-default ui-selectee" name="eleven">11</li>
<li class="ui-state-default ui-selectee" name="twelve">12</li>
</ol>
If you click a number, its background color changes to orange. Try selecting the tiles 1, 3, and 5. You do that by holding down Ctrl + tile 1 + tile 3 + tile 5. This involves performing multiple actions, that is, holding Ctrl continuously and clicking on tiles 1, 3, and 5. How do we perform these multiple actions using WebDriver? The following code demonstrates how:
@Test
public void shouldPerformCompositeAction() {
driver.get("http://guidebook.seleniumacademy.com/Selectable.html");
WebElement one = driver.findElement(By.name("one"));
WebElement three = driver.findElement(By.name("three"));
WebElement five = driver.findElement(By.name("five"));
// Add all the actions into the Actions builder.
Actions actions = new Actions(driver);
actions.keyDown(Keys.CONTROL)
.click(one)
.click(three)
.click(five)
.keyUp(Keys.CONTROL);
// Generate the composite action.
Action compositeAction = actions.build();
// Perform the composite action.
compositeAction.perform();
}
Now, if you refer to the code, we are getting introduced to a new class named Actions. This Actions class is the one that is used to emulate all the complex user events. Using this, the developer of the test script could combine all the necessary user gestures into one composite action. We have declared all the actions that are to be executed to achieve the functionality of clicking on the numbers 1, 3, and 5. Once all the actions are grouped together, we build that into a composite action. Action is an interface that has only the perform() method, which executes the composite action. When we execute the test, tiles 1, 3, and 5 will be selected one by one. Finally, tile 5 will be selected, as shown in this screenshot:

So, to make WebDriver perform multiple actions at the same time, you need to follow a three-step process of using the user-facing API of the actions class to group all the actions, then build the composite action, and perform the action. This process can be made into a two-step process, as the perform() method internally calls the build() method. So the previous code will look as follows:
@Test
public void shouldPerformAction() {
driver.get("http://guidebook.seleniumacademy.com/Selectable.html");
WebElement one = driver.findElement(By.name("one"));
WebElement three = driver.findElement(By.name("three"));
WebElement five = driver.findElement(By.name("five"));
// Add all the actions into the Actions builder.
Actions actions = new Actions(driver);
actions.keyDown(Keys.CONTROL)
.click(one)
.click(three)
.click(five)
.keyUp(Keys.CONTROL);
// Perform the action
actions.perform();
}
In the preceding code, we have directly invoked the perform() method on the Actions instance, which internally calls the build() method to create a composite action before executing it. In the subsequent sections of this chapter, we will take a closer look at the Actions class. All the actions are basically divided into two categories: mouse-based actions and keyboard-based actions. In the following sections, we will discuss all the actions that are specific to the mouse and keyboard available in the Actions class.