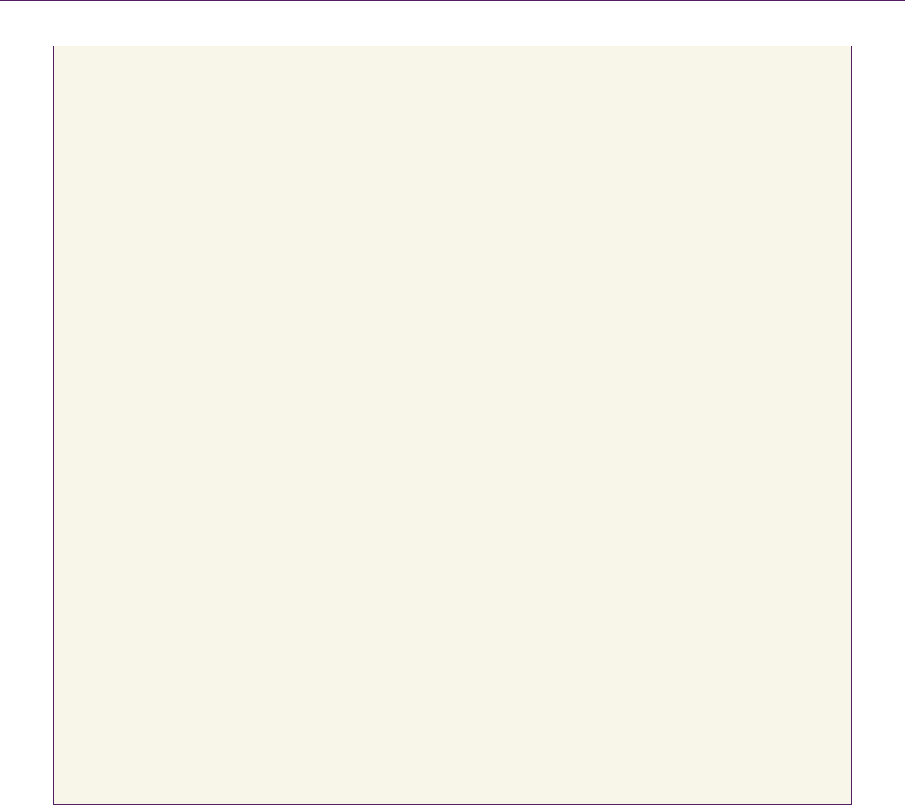
10.5UsingGeometryShadertoRenderStereoPairs 159
// Creating center/left/right view-projection matrices.
void buildViewProjectionMatrices()
{
// Get current monoscopic camera in scene.
Camera camera = getCurrentCamera();
// Create right vector from normalized up and direction vector.
float3 right = Cross(&camera.up, &camera.dir);
// Calculate horizontal camera offsets and frustum plane offsets.
float3 cameraOffset = 0.5F * camera.Wa * right;
float planeOffset = 0.5F * camera.Wa * camera.zn / camera.z0;
// Create left eye camera from center camera.
Camera cameraLeft = camera;
cameraLeft.pos -= cameraOffset;
cameraLeft.l += planeOffset;
cameraLeft.r += planeOffset;
// Create right eye camera from center camera.
Camera cameraRight = camera;
cameraRight.pos += 0.5F * camera.Wa * right;
cameraRight.l -= planeOffset;
cameraRight.r -= planeOffset;
// Store camera view-projection matrices.
g_viewProjMatrix = getViewProjMatrix(camera);
g_viewProjMatrixLeft = getViewProjMatrix(cameraLeft);
g_viewProjMatrixRight = getViewProjMatrix(cameraRight);
}
Listing 10.1. Pseudocode for camera structure (left-handed space).
10.5UsingGeometryShadertoRenderStereoPairs
On some stereo display formats, the left eye and right eye may be packed in the
same back buffer, and we can use viewports to control which eye to render to. In
Direct3D 10 and OpenGL 4.1, the geometry shader can be used to generate ge-
ometry as well as redirect output to a specific viewport. Instead of rendering ste-
reo in multiple passes, one can use the geometry shader to generate geometry for