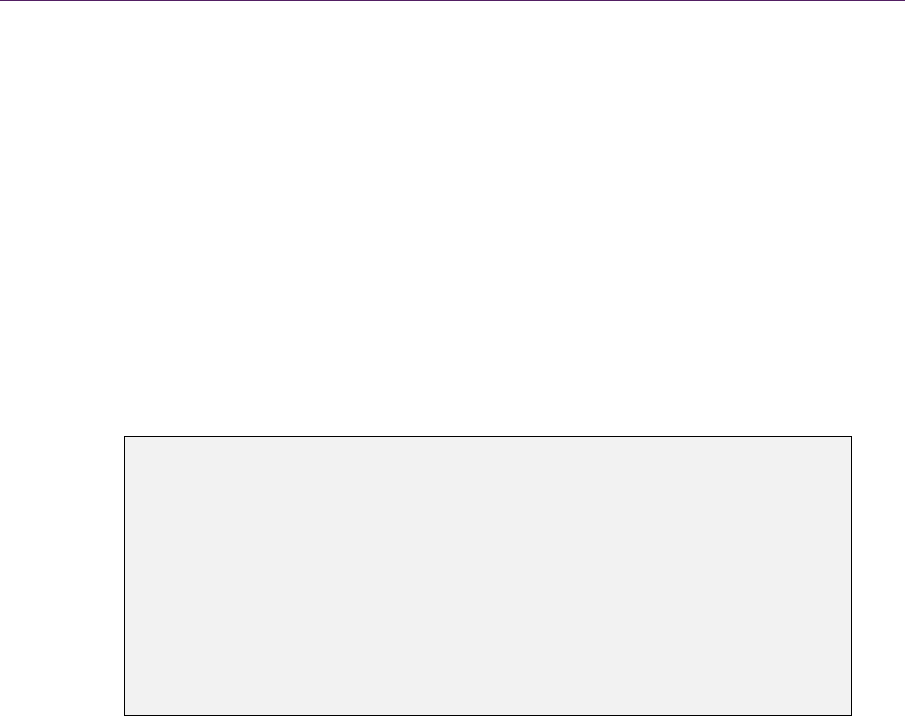
18.5PublishorPerish 317
you never publish (or receive) the actual properties used to render location and
orientation. If you do, the remote actors will get an update and render the last
known values instead of the results of dead reckoning. It’s an easy thing to over-
look and results in a massive one-frame discontinuity (a.k.a. blip). Instead, create
publishable properties for the last known values (i.e., location, velocity, accelera-
tion, orientation, and angular velocity) that are distinct from the real values.
The second consideration is partial actor updates, messages that only contain
a few actor properties. To obtain believable dead reckoning, the values in the
kinematic state need to be published frequently. However, the rest of the actor’s
properties usually don’t change that much, so the publishing code needs a way to
swap between a partial and full update. Most of the time, we just send the kine-
matic properties. Then, as needed, we send other properties that have changed
and periodically (e.g., every ten seconds) send out a heartbeat that contains eve-
rything. The heartbeat can help keep servers and clients in sync.
MythBusting—AccelerationIsNotAlwaysYourFriend
In the quest to create believable dead reckoning, acceleration can be a huge
advantage, but be warned that some physics engines give inconsistent (a.k.a.
spiky) readings for linear acceleration, especially when looked at in a single
frame as an instantaneous value. Because acceleration is difficult to predict
and is based on the square of time, it can sometimes make things worse by in-
troducing noticeable under- and overcompensations. For example, this can be
a problem with highly jointed vehicles for which the forces are competing on
a frame-by-frame basis or with actors that intentionally bounce or vibrate.
With this in mind, the third consideration is determining what the last known
values should be. The last known location and orientation come directly from the
actor’s current render values. However, if the velocity and acceleration values
from the physics engine are giving bad results, try calculating an instantaneous
velocity and acceleration instead. In extreme cases, try blending the velocity over
two or three frames to average out some of the sharp instantaneous changes.
PublishingTips
Below are some final tips for publishing:
■ Published values can be quantized or compressed to reduce bandwidth
[Sayood 2006].