Let's add our first piece of logic using an if statement. An if statement is a simple statement to determine whether or not a statement is true. Input the following into Xcode:

In the first line of the preceding code, we created a constant named isPictureVisible, and we set it to true. The next line starts our if statement and reads as follows: if isPictureVisible is true, then print Picture is visible. When we write if statements, we must use the curly braces to enclose our logic. It is a good practice to put the opening curly brace ({) on the same line as the if statement and the closing curly brace (}) on the line immediately after your logic.
When writing if statements using a bool, you are always checking for true; however, if you wanted to check for false, you would do the following:

Bools work great with if statements, but we also can use them with other data types. Let's try an if statement with an Int next. Write the following in Playgrounds:

In the preceding example, we first created another constant with our Int set to 19. The next line says: if the drinkingAgeLimit is less than 21, then print Since we cannot offer you an adult beverage - would you like water or soda to drink? When you are using Int within if statements, you will use the comparison operators (<, >, <=, >=, ==, or !=). However, our last if statement feels incomplete because we are not doing anything for someone over 21. When you need to cover the contradictory statement, this is where you will utilize an if...else statement. You enter an if...else statement precisely as you do with an if statement, but, at the end, you add the word else.
You can add else to both of the if statements we have inputted so far, but, for now, add it to the end of our last if statement:
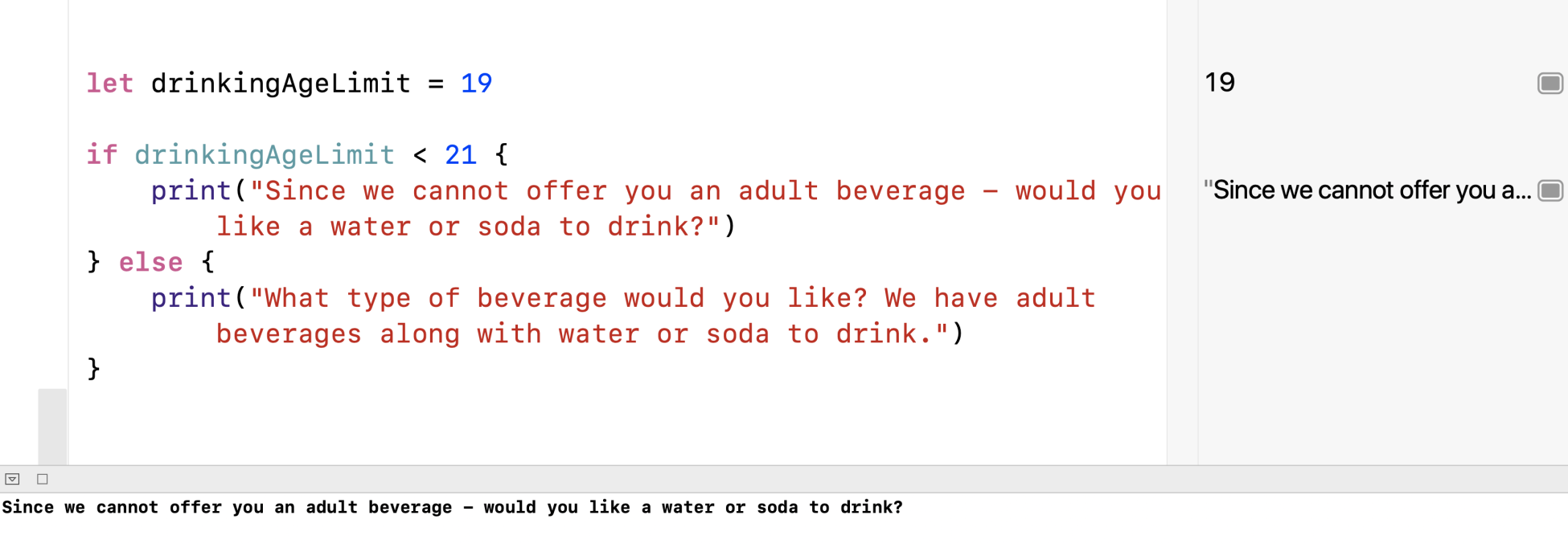
With else added to the end of our if statement, it turns into an if...else statement, which now reads: if the drinkingAgeLimit is less than 21, then print Since we cannot offer you an adult beverage - would you like water or soda to drink? Otherwise (or else), print What type of beverage would you like? We have adult beverages along with water or soda to drink.
Now, our if...else statement can handle both conditions. Based on the value 19 for our drinkingAgeLimit, we can see the following in the Debug Panel: Since we cannot offer you an adult beverage - would you like water or soda to drink? If we change drinkingAgeLimit to 30, our Debug Panel says What type of beverage would you like? We have adult beverages along with water or soda to drink. Go ahead and change 19 to 30 in Playgrounds:

Note that we got the behavior we wanted in the Debug Panel.
So far, we have covered using an if statement with a bool and an Int. Let's take a look at one more example using a string. Add the following bit of code to Playgrounds:
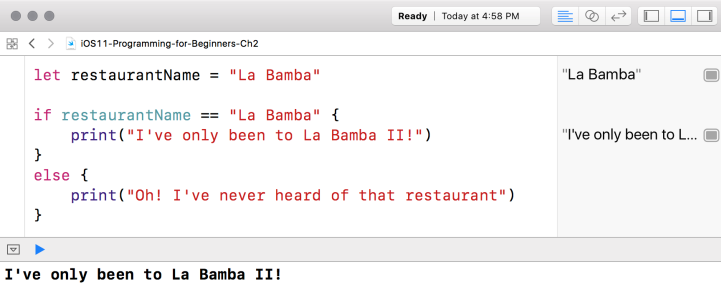
In programming, we use equals (=) when setting data to variables. However, to compare two data types, we must use the double equals (==) sign. Therefore, when we write an if statement that compares two strings, we must use double equals (==) instead of a single equal (=) to determine equality.
An if...else statement only lets us check two conditions, whether they are true or not. If we wanted to add more conditions, we would not be able to use just an if...else statement. To accomplish this, we would use what is called an if...else...if...else statement. This statement gives us the ability to add any number of else-if inside our if...else statement. We will not go overboard, so let's add one. Update your last if...else statement to the following:

In this example of an if...else...if...else statement, we are checking whether restaurantName equals La Bamba, then print I've only been to La Bamba II!, else if restaurantName equals La Bamba II, then print This restaurant is excellent!, else print Oh! I've never heard of that restaurant.
Using if, if...else, and if...else if...else statements helps you to create simple or complex logic for your app. Being able to use them with Strings, bools, Ints, and floating-point numbers give you more flexibility.