Let's add some data to our array. There are a few different convenience methods for adding data to an array.
A convenience method is, just as its name implies, a method that makes things convenient. A method is a function that lives inside a class. We will discuss classes later in this book. If this is starting to get overwhelming, that's understandable. You do not need to worry about every single detail at this time. We will cover this again, and things will slowly start to click at some point. Everyone learns differently, so there is no reason to worry if someone else understands something more quickly. Just go at your own pace.
The first convenience method we will look at is the append() method:
states.append(23)
Your code and the output window should now look like this:

Houston, we have a problem! You will see that we are getting an error. I did this for a couple of reasons, because getting errors is normal and common. Most people who start out coding are afraid to make a mistake or get scared about getting or seeing errors. Trust me, I have been coding for years, and I make mistakes all the time. The error is telling us that we tried to add an int into an array that can only hold strings.
For every developer, whether they are a beginner or a veteran, there will come a time when they encounter an error that they cannot figure out. This error might get you frustrated to the point where you want to throw the computer across the room (I have been there a few times). The best advice my boss ever gave me was to take a walk for 10-15 minutes or do something to take your mind off it. Sometimes, this helps, and you will come up with an idea after you walk away. Even if you come back and it still takes you hours to figure out what is wrong, this is still part of the process. The best errors are the ones where you overlooked the simplest thing and had to spend hours trying to figure it out. You might have lost time, but you will have learned a great lesson. Lessons like these will stay with you forever, and you will never forget the error the next time you encounter it. So, if your coding results in an error, even in this book, embrace the challenge, because there is no greater feeling than figuring out a challenging error.
So, let's correct what we just did by revising the array to show the following:
states.append("Florida")
The following is how your code should now look:
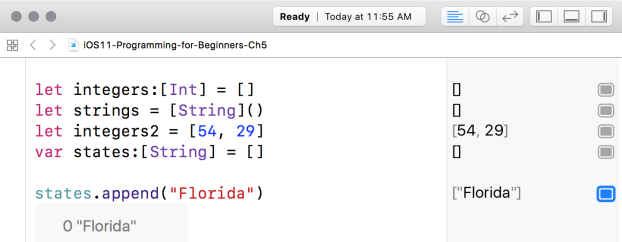
In the Results Panel, you will see the contents of our corrected array.
Since an array can hold any number of items, let's add some more. Earlier, I mentioned that we have a variety of ways to add items to an array. The append() method allows us to add only one item at a time. To add multiple items, we can use the convenience called append(contentsOf:).
Add the following to Playgrounds:
states.append(contentsOf:["California", "New York"])
Now, your code should look like this:

We added two more items to our array, but, so far, every example we have utilized has added items at the end of our array. We have two convenience methods that allow us to add items at any index position that is available in the array.
The first method we can utilize to do this is called insert(at:), which allows us to add a single item at a specific index position. We also have insert(contentsOf:at:), which enables us to add multiple items into an array at a certain index position. Let's use them both and add Ohio after Florida and then North Carolina, South Carolina, and Nevada in front of New York:
states.insert("Ohio", at:1)
states.insert(contentsOf:["North Carolina", "South Carolina", "Nevada"],at:3)
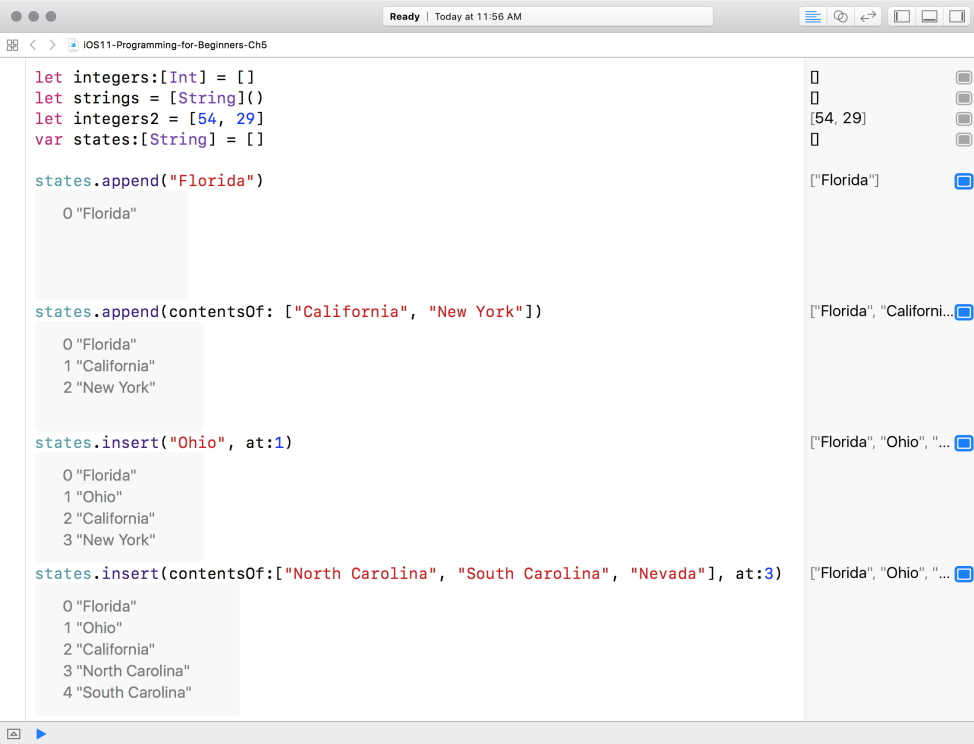
We just added items to our array using append(contentsOf:), but there also is a shorthand version of this, which is done by using the += operator. As a next step, let's add the following:
states += ["Texas", "Colorado"]
Now, your code should look like this:

This technique for adding items is much more concise and is my preferred way of inserting items into an array. Writing less code is not always better but, in this case, using the += operator is my go-to method.