Now, it is time to get into an enjoyable part of programming and learn how to write functions. Functions are self-contained pieces of code that you want to run on something. In Swift 3, Apple made a change to how you should write functions. All of the functions we will write in this chapter will perform an action (think of verbs). Let's create a simple function called greet():
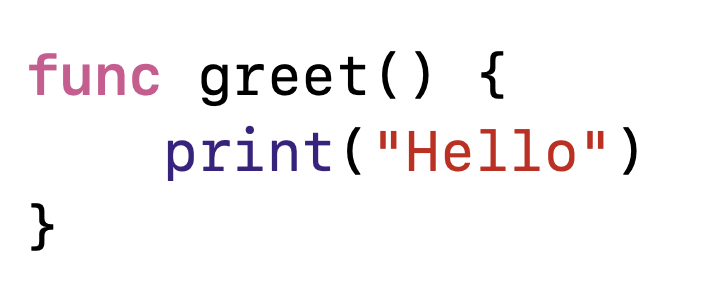
This example is a basic function with a print statement in it. In programming, functions do not run until you call them. We call a function by merely calling its name. So, let's call greet, as follows:
greet()
Once we add this to the code, this is what we'll see on screen:

That's it! We just created our first function and called it. However, functions can do so much more. We can add what is called a parameter to a function. A parameter allows us to accept data types inside our parentheses. Doing this will enable us to build more reusable chunks of code. So, let's update our greet() function to accept a parameter called name:
func greet(name:String) {
print("Hello")
}
After you update the function, you will get the following error:

We received this error because we updated our function, but we did not update the line where we called it. Let's update where we call greet() to the following:
greet(name: "Joshua")
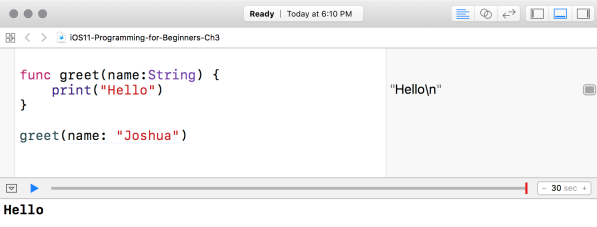
The preceding code looks good; however, the Debug Panel shows us that we are not using the name in our greeting. Earlier, you learned how to create a string interpolation. So, we need to append our variable name inside our print statement, as follows:
print("Hello (name)")
This is how your code will now look:

Functions can take multiple parameters, so let's create another greet() function that takes two parameters, a first name, and a last name:
func greet(first:String, last:String) {
print("Hello (first) (last)")
}
Now, your code and its output should look as shown in the following screenshot:

We also need to update where we called greet() in order to accept multiple parameters as well:
greet(first: "Craig", last: "Clayton")
Now, your code and output screen should look something like this:
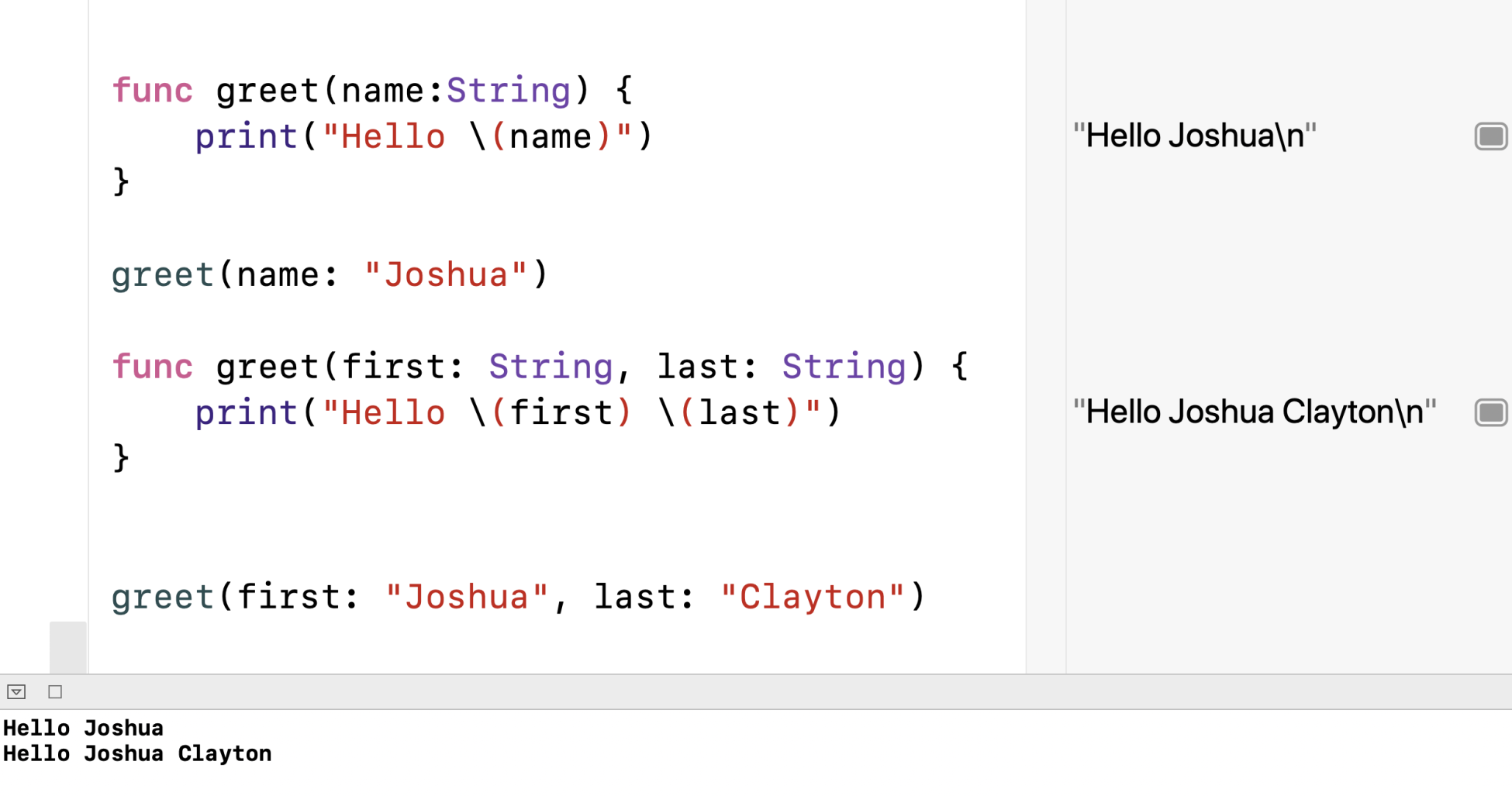
We now have a function that takes multiple parameters.
Functions can perform an action, but they can also run an action, and then, when it is done, return back some type of data. Whenever we want our function to return something, we need to use a noun as a way to describe what our function will do. We just created a function called greet() that takes a first and last name and creates a full name.
Now, let's create another function called greeting(), which will return a full name with a greeting. Let's see what this looks like:
func greeting(first:String, last:String) -> String {
return "Hello (first) (last)"
}
The following is how your code and output screen should appear:
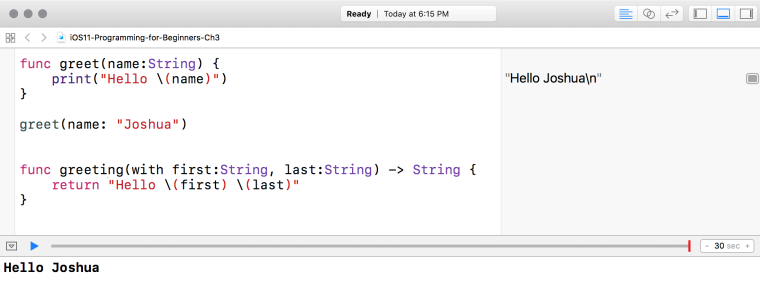
This function is almost the same as the previous one, but with a couple of new things. First, -> String tells the function that we want to return a string. Inside our function, we return "Hello (first) (last)." Since we said that we want to return something after our parentheses, we have to do just that. Now, let's look at how we can do this. Enter the following code:
print(greeting(first:"Teena", last:"Harris"))
Now, this is how your code and output screen should look:

As you may have noticed, in the Debug Panel, we now have our full name with Hello added to the beginning. As you start to build on functions, you start to see the power.
These are just the basics of functions. We will cover more advanced functions throughout our Let's Eat app. The main thing novice programmers forget is that functions should be small. Your function should do one thing and one thing only. If your function is too long, then you need to break it up into smaller chunks. Sometimes, longer functions are unavoidable, but you should always be mindful of keeping them as small as possible. Nice work!
Let's work
We covered a lot in this chapter, and now it is time to put everything we covered into practice. Here are two challenges. If you are comfortable with them, then work on them on your own. Otherwise, go back into this chapter, where you can follow along with me and see how you can do each one:
- Challenge 1: Write a function that accepts and returns a custom greeting (other than Hello, which we addressed earlier in this chapter), along with your first and last name
- Challenge 2: Write a function that will take two numbers and add, subtract, multiply, or divide those two numbers