Now, it is time to start deleting items from our array. Let's delete the first item from our list. We have a convenience method for removing items from an array, called removeFirst(). This method will remove the first item from our array, which, in our case, is Florida. Let's remove Florida and add this line above our for...in loop:
let updatedStates = states.removeFirst()
for state in states {
print(state)
}
This is how our code and output should now look:

Since we removed Florida, all of our states' index positions will be updated to move one position closer to the top of the array. But what if we wanted to remove an item that was not first? To do this, we can use the remove(at:) convenience. So, let's remove North Carolina and New York, which are sitting at positions 2 and 4, respectively. We will add the following above our for...in loop:
states.remove(at:2) states.remove(at:4)
This is how our code and output should now look:
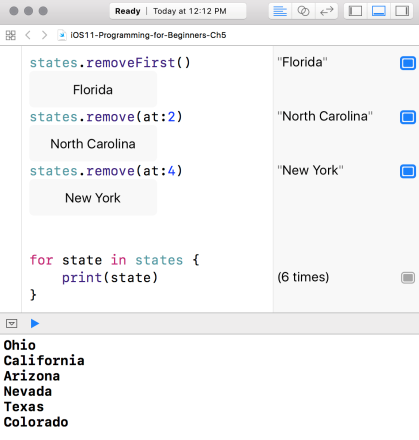
Now, both North Carolina and New York have been removed. You will see that California and Ohio did not move, but Colorado and Nevada moved up closer to the top of the list. To remove the remaining six items, we could use remove(at:) for each one, but instead we will use the simpler method of removeAll(). So, let's use removeAll() in Playgrounds:
states.removeAll()
Now, your code should look something like this:
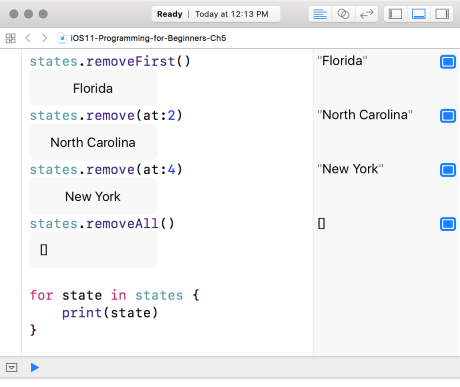
Now, we are back to where we started with an empty array. We have only scratched the surface of arrays. We will do more with arrays later in this book, but we first need to look at the next collection type: dictionaries.