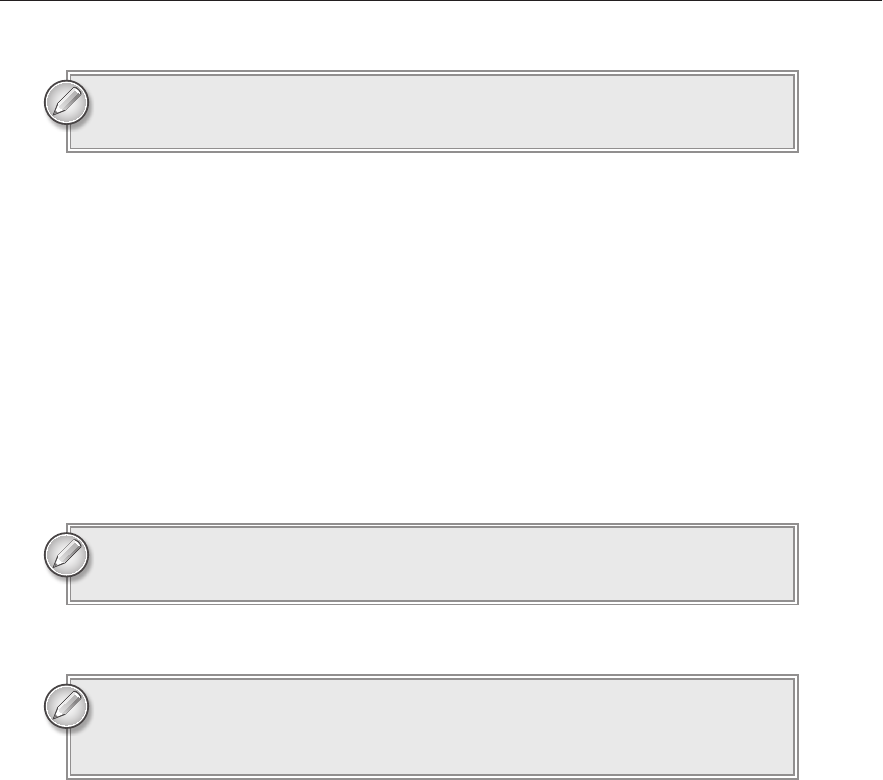
Getting Data from the Clipboard
371
The StringCollection class is in the System.Collections namespace, so the
program includes a
using directive to make using the class easier.
The audio copying button copies an audio resource to the clipboard.
To add an audio resource to the project at design time, open the Project menu and select Properties
at the bottom. Next click the Resources tab. Click the Add Resource dropdown arrow at the top of
the page and select Add Existing File. Select the audio file that you want to add to the project and
click Open.
Visual Studio adds the audio file to the project and creates a memory stream property that you can
use to refer to it. In this example, the file I used was named boing.wav so the program can refer to it
as
Properties.Resources.boing.
The audio copying button calls the
Clipboard’s SetAudio method, passing it the audio resource.
Then instead of playing the system’s beep sound, the program creates a
SoundPlayer associated
with the audio resource and calls the player’s
Play method.
The SoundPlayer class is in the System.Media namespace, so the program
includes a
using directive to make using the class easier.
Programs that accept audio pasted from the clipboard are uncommon. To
test this program’s ability to copy audio to the clipboard, you can use the
ReadFromClipboard example program described in the following section.
GETTING DATA FROM THE CLIPBOARD
Getting data from the clipboard is just as easy as putting it there because the Clipboard class provides
static methods that return audio streams, file drop lists, images, and text.
Before you try to copy data from the clipboard, however, you should make sure the data you want is
present. To let you do that, the
Clipboard class also provides a set of functions that tell you whether
data in a given format is available.
For example, the following code checks whether text is available on the clipboard and, if it is, copies
the text into the textbox named
dataTextBox:
if (Clipboard.ContainsText()) dataTextBox.Text = Clipboard.GetText();
596906c32.indd 371 4/7/10 12:34:35 PM