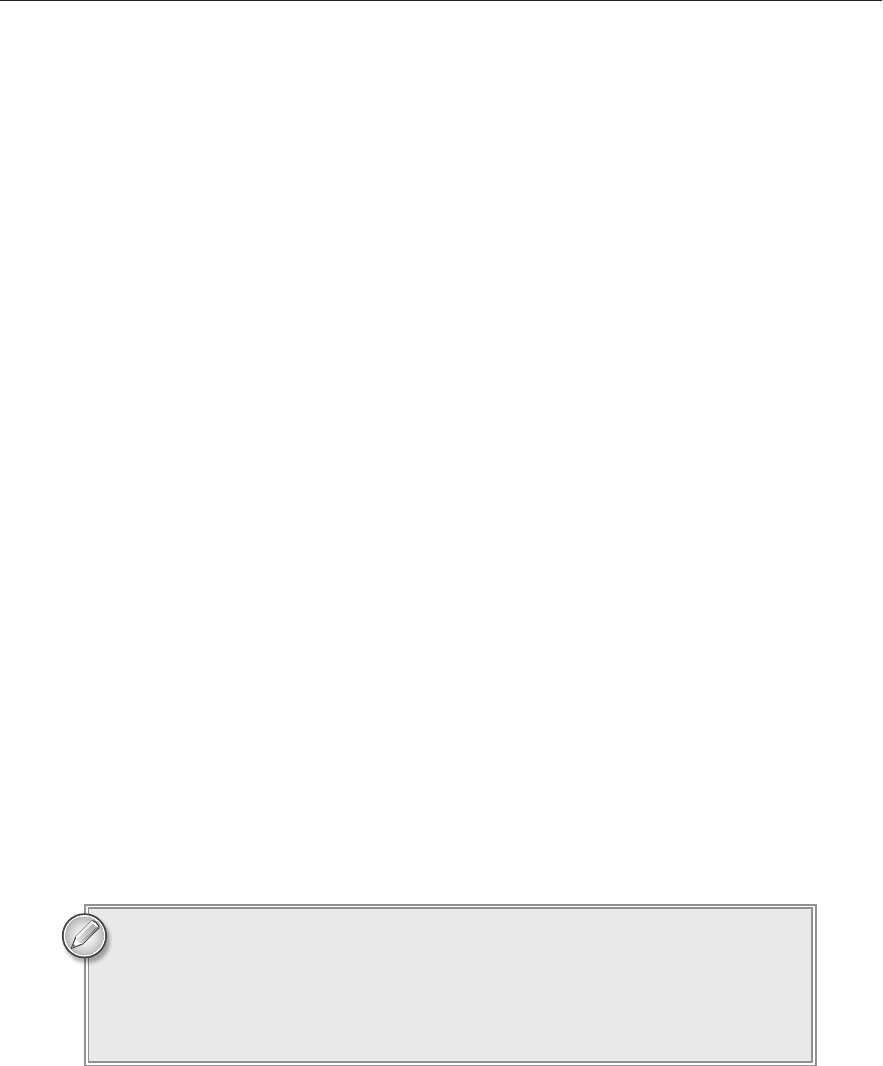
444
LESSON 39 Drawing with gDi+
The four rectangles on the left demonstrate a TextureBrush, PathGradientBrush,
LinearGradientBrush, and SolidBrush. The three overlapping circles demonstrate transpar-
ent
SolidBrushes, and the square on the right demonstrates a TextureBrush filled with a small
repeating image.
For example, the following code shows how the program draws the hatched rectangle in the upper
left corner.
// Use a HatchBrush.
using (HatchBrush br = new HatchBrush(
HatchStyle.DiagonalBrick,
Color.Green,
Color.White))
{
e.Graphics.FillRectangle(br, 10, 10, 100, 50);
e.Graphics.DrawRectangle(Pens.Black, 10, 10, 100, 50);
}
You can look at the code for additional details, but I will mention a few extra facts about the
different kinds of brushes.
The
HatchBrush provides more than 50 hatch patterns. If you want a pattern that isn’t in the
list, use a
TextureBrush. Parameters to the HatchBrush’s constructor give the pattern’s fore-
ground and background colors.
The
LinearGradientBrush shades from one color at one point to another color at another
point. More complicated versions of the brush can shade between several colors and points
and let you change the way colors drop off.
The
PathGradientBrush makes colors shade from points along a path to another color at a
“center point,” although you can move the “center point” so it isn’t actually in the center.
Stock
SolidBrushes use predefined, solid, opaque colors. You can also define SolidBrushes
by specifying the brush’s color and that color can be translucent.
By default the
TextureBrush tiles the area it is filling with copies of its image. You can change
that behavior so the brush fills the area with different kinds of reflections of the image if you
like. For example, it could flip copies that are adjacent in the X direction horizontally so they
look like mirror images.
Instead of passing x, y, width, and height parameters to rectangle or ellipse
methods, you can pass a
Rectangle or RectangleF.
If you are going to fill a shape and then outline it, use the same
Rectangle or
RectangleF for both so you’re guaranteed that the fill and draw methods line up
properly, even if you need to change the location later.
You can learn more about the Brush class’s properties by looking at the following web pages:
HatchBrush
— msdn.microsoft.com/library/system.drawing.drawing2d
.hatchbrush.aspx
596906c39.indd 444 4/7/10 12:35:14 PM