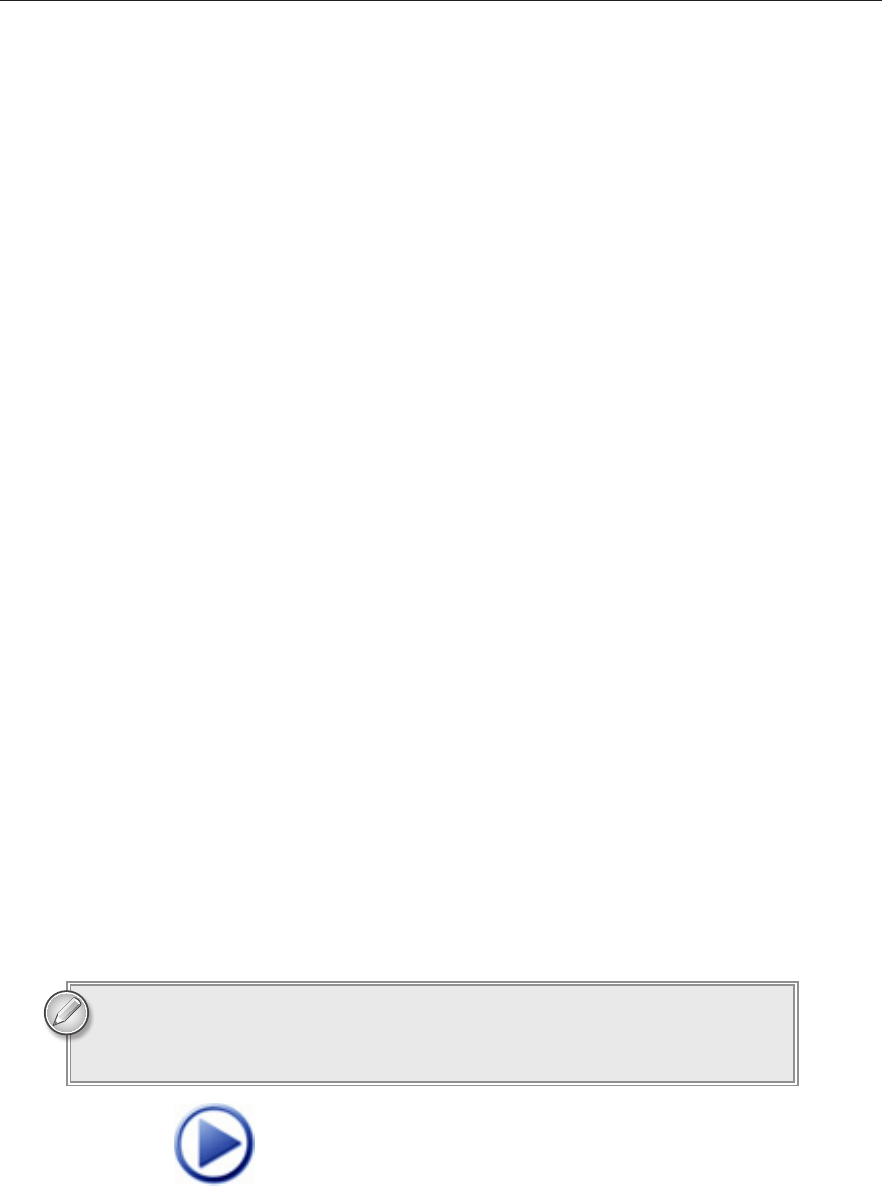
If the user made a choice for all of the ListBoxes, create a variable total to hold the total
cost. Use three
switch statements to add the appropriate amounts to total and display the
result. (Tip: Add a
default statement to each switch statement to catch unexpected selec-
tions, even though none should occur in this program. Try adding a new hotel and see what
happens if you select it.)
2. (SimpleEdit) Copy the SimpleEdit program you built in Lesson 8, Exercise 3 (or download
Lesson 8’s version from the book’s web site) and add code to protect the user from losing
unsaved changes.
The basic idea is to check whether the document has been modified before doing anything
that will lose the changes, such as starting a new document, opening another file, or exiting
the program.
a. In the File menu’s New, Open, and Exit event handlers, check the RichTextBox’s
Modified property to see if the document has unsaved changes.
b. If there are unsaved changes, ask if the user wants to save them. Display a message box
with the buttons Yes, No, and Cancel.
c. If the user clicks Yes, save the changes and continue the operation.
d. If the user clicks No, don’t save the changes (do nothing special) and let the operation
continue.
e. If the user clicks Cancel, don’t perform the operation. For example, don’t open a new
file.
f. After starting a new document or saving an old one, set the RichTextBox control’s
Modified property to false to indicate that there are no unsaved changes any more.
3. (SimpleEdit) Copy the SimpleEdit program you built for Exercise 2. That program protects
against lost changes if the user opens the File menu and selects Exit, but there are several
other ways the user can close the program such as pressing [Alt]+F4, clicking the “X” button
in the program’s title bar, and opening the system menu in the form’s upper left corner and
selecting Close. Currently the program doesn’t guard unsaved changes for any of those.
To fix this, give the form a
FormClosing event handler. When the form is about to close,
it raises this event. If you set the event’s
e.Cancel parameter to true, the form cancels the
close and remains open. Add code to this event handler to protect unsaved changes.
Now that the
FormClosing event handler is protecting against lost changes, you don’t need
to perform the same checks in the Exit menu item’s event handler. Make that event handler
simply call
this.Close and FormClosing will do the rest.
You can download the solutions to these exercises from the book’s web page at
www.wrox.com or www.CSharpHelper.com/24hour.html. You can find them in
the Lesson18 folder.
596906c18.indd 227 4/8/10 8:13:19 AM
Click here to Play