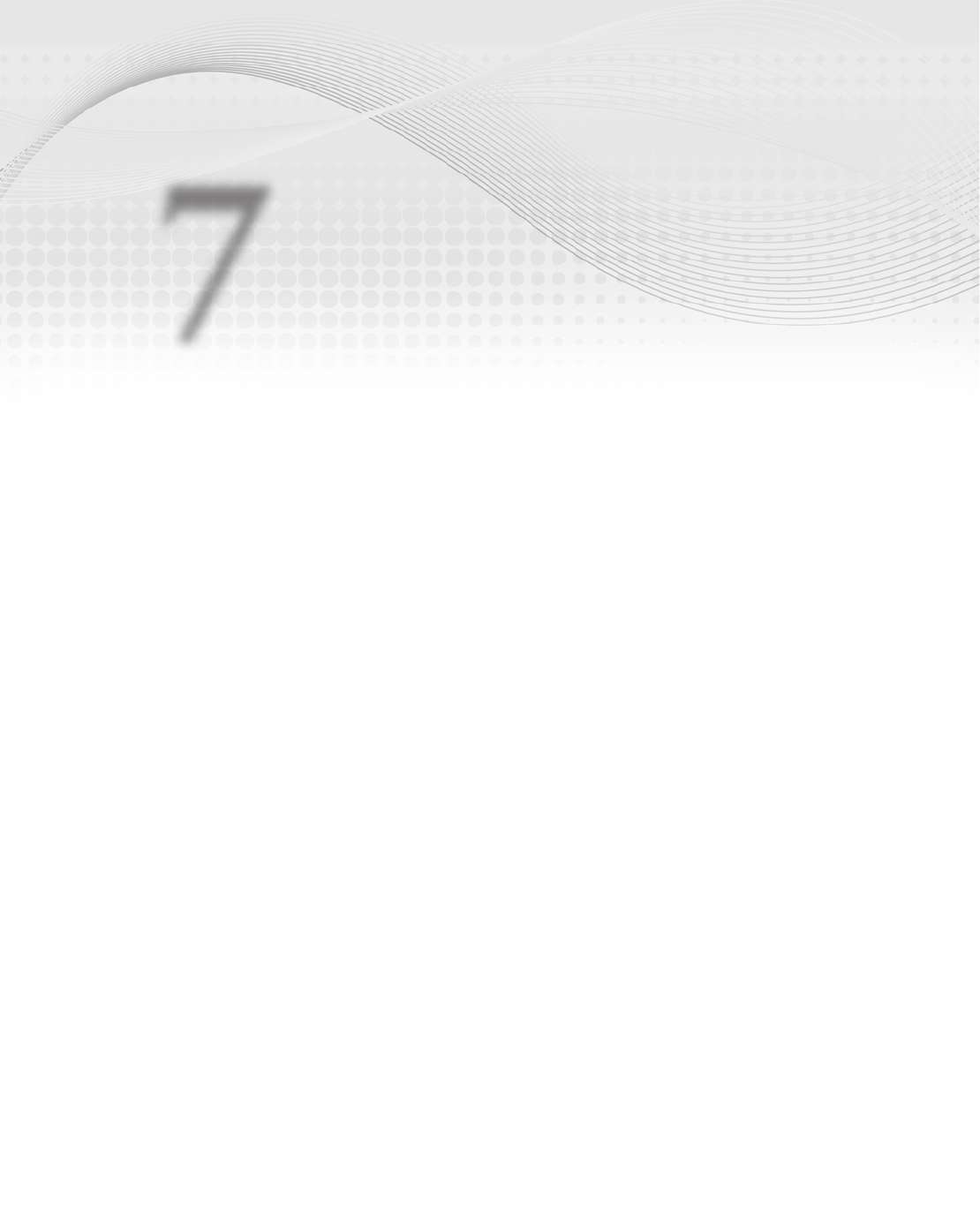
Using RichTextBoxes
The TextBox control lets the user enter text and that’s about it. It can display its text in different
colors and fonts, but it cannot give different pieces of text different properties. The
TextBox is
intended to let the user enter a simple string, like a name or street address, and very little more.
The
RichTextBox is a much more powerful control. It can display different pieces of text with
different colors, fonts, and styles. It can adjust paragraph indentation and make bulleted lists.
It can even include pictures. It’s not as powerful as a full-featured word processor, such as
Microsoft Word or OpenOffice’s Writer, but it can produce a much more sophisticated result
than the
TextBox.
In this lesson you learn more about the
RichTextBox control and how to use it. You have
a chance to experiment with the control, and you use it to add enough functionality to the
SimpleEdit program to finally make the program useful.
USING RICHTEXTBOX PROPERTIES
To change the appearance of the text inside a RichTextBox, you first select the text that you
want to change, and then you set one of the control’s properties to modify the text.
To select the text, you use the control’s
SelectionStart and SelectionLength properties
to indicate where the text begins and how many letters it includes. Note that the letters are
numbered starting with 0. (In fact, almost all numbering starts with 0 in C#.) For example,
setting
SelectionStart = 0 and SelectionLength = 1 selects the control’s first letter.
After you select the text, you set one of the
RichTextBox’s properties to the value that you
want the text to have.
For example, the following code makes the
RichTextBox named rchContent display some
text and color the word “red.”
contentRichTextBox.Text = “Some red text”;
contentRichTextBox.SelectionStart = 5;
contentRichTextBox.SelectionLength = 3;
contentRichTextBox.SelectionColor = Color.Red;
7
596906c07.indd 79 4/7/10 12:32:04 PM