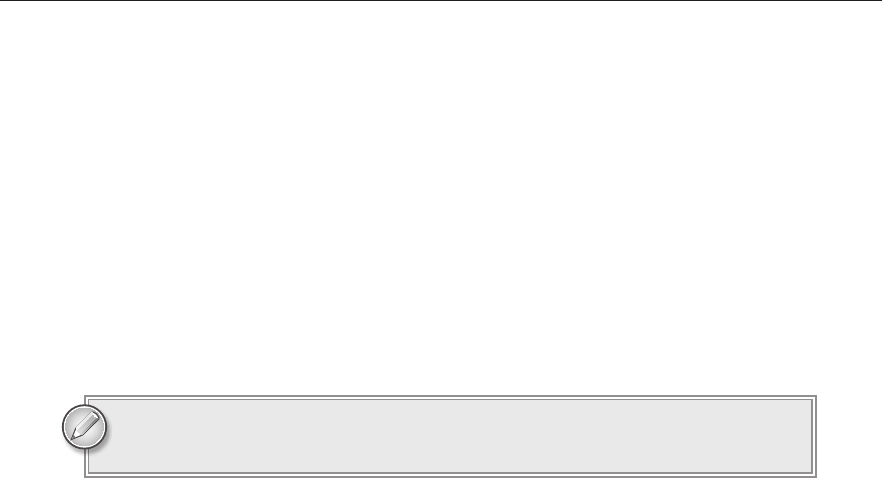
454
LESSON 40 Making WPF aPPlications
<Grid>
<Image Height=”100” HorizontalAlignment=”Left” Margin=”12,12,0,0”
Name=”butterflyImage” Source=”/Critters;component/Butterfly.jpg”
Stretch=”Uniform” VerticalAlignment=”Top” Width=”100”
MouseDown=”butterflyImage_MouseDown” />
... Other Image controls omitted ...
</Grid>
</Window>
If you study this code, you’ll see that the Window contains a Grid and the Grid contains the Image
controls. You’ll also notice that the XAML code gives the name of the code-behind event handlers
that should execute when the user presses the mouse down on an
Image. In the code shown here, the
MouseDown event handler is called butterflyImage_MouseDown.
A Window can contain only a single child control. Normally that is a container
such as a
Grid that holds all of the other controls.
Although this simple application works, it could use some improvements. For example, the images
are not centered vertically. They also do not resize to take advantage of the form’s current size.
One of the philosophical goals of WPF is to make controls arrange themselves to make the best use
of whatever spaces is available. In a Windows Forms application you might use
Anchor properties to
do this. In a WPF application, you generally use container controls that help arrange their children.
The following section shows how you can improve the way this program arranges its controls.
ARRANGING WPF CONTROLS
The first version of the Critters program placed Image controls directly inside the Window’s Grid. The
Grid control arranges its children in rows and columns, although the designer creates only one row and
column by default. A better arrangement for this program would use five columns: one for each
Image.
To make the columns, select the
Grid control. In the Properties window, click the ellipsis button to
the right of the
ColumnDefinitions property and use the dialog that appears to create five columns.
By default the columns have
Width set to *, which means they divide the Grid’s area evenly.
Because the
Image controls were originally placed in the Grid’s first column, they are still contained
in that column. To move the controls into their proper columns, set their
Grid.Column properties.
Note that the columns are numbered starting with 0 so the column indexes should be 0, 1, 2, 3, and 4.
Originally the
Image controls used their Margin properties to position themselves within the Grid’s
first row and column. Now those values move them to strange locations within their new columns.
For example, the frog
Image’s Margin value of 118,12,0,0 moves the image 118 pixels to the right so
it isn’t visible in its column.
596906c40.indd 454 4/7/10 12:35:19 PM