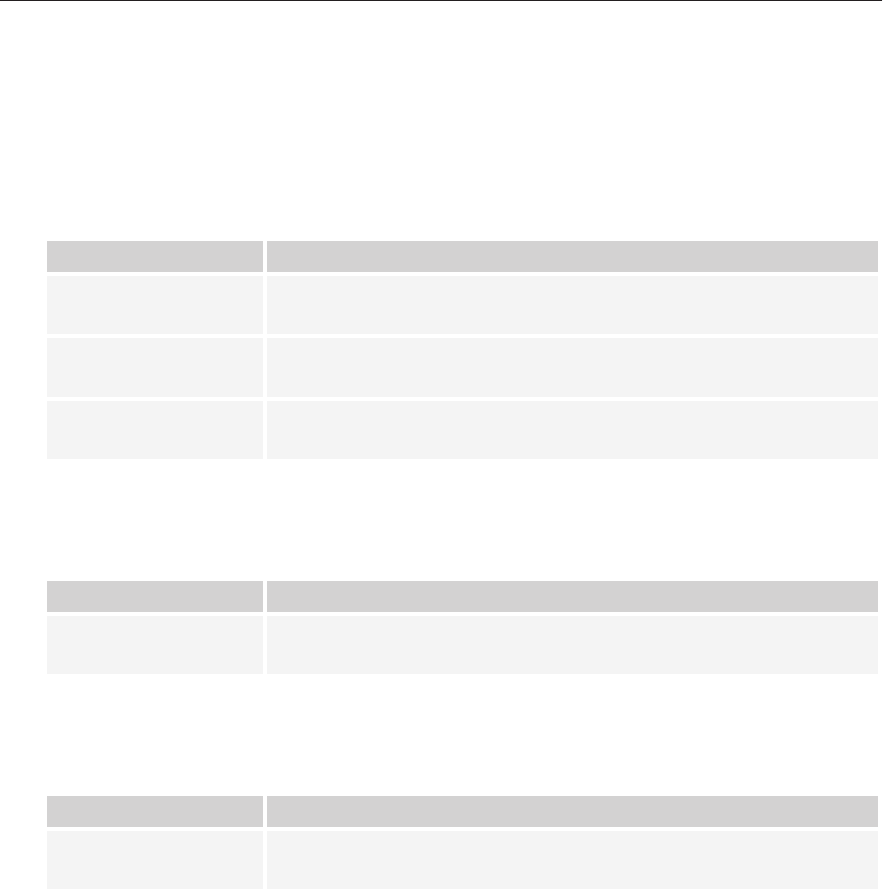
96
LESSON 8 Using standard dialogs
The SaveFileDialog has many of the same properties as the OpenFileDialog. See Table 8-5 for
descriptions of the properties
AddExtension, CheckFileExists, CheckPathExists, DefaultExt,
FileName, Filter, FilterIndex, InitialDirectory, and Title.
Table 8-6 summarizes
SaveFileDialog properties that are not shared with the OpenFileDialog.
TABLE 86
PROPERTY PURPOSE
CreatePrompt If this is True, and the user selects a file that doesn’t exist, the dialog asks
if the user wants to create the file.
OverwritePrompt If this is
True and the user selects a file that already exists, the dialog
asks if the user wants to overwrite it.
ValidateNames Determines whether the dialog verifies that the filename doesn’t contain
any invalid characters.
Table 8-7 summarizes the PrintDialog’s most useful property.
TABLE 87
PROPERTY PURPOSE
Document You set this property to tell the dialog what document object to print.
Lesson 31 has more to say about this.
Table 8-8 summarizes the PrintPreviewDialog’s most useful property.
TABLE 88
PROPERTY PURPOSE
Document You set this property to tell the dialog what document object to preview.
Lesson 31 has more to say about this.
USING FILE FILTERS
Most of the dialogs’ properties are fairly easy to understand. Two properties that are particularly
confusing and important, however, are the
Filter and FilterIndex properties provided by the
OpenFileDialog and SaveFileDialog.
The
Filter property is a list of text prompts and file matching patterns separated by the | character.
The items alternate between text prompts and the corresponding filter. The dialog provides a drop-
down list where the user can select one of the text prompts. When the user selects a prompt, the dialog
uses the corresponding filter to decide which files to display.
596906c08.indd 96 4/7/10 12:32:12 PM