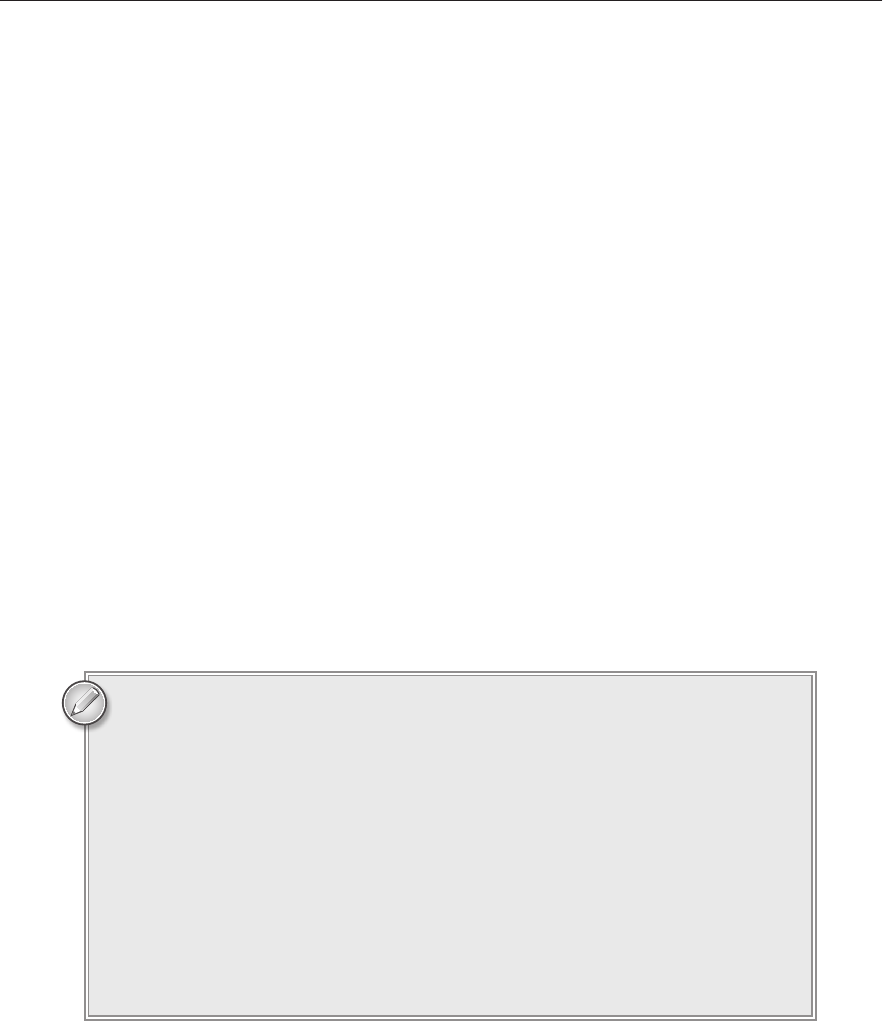
418
LESSON 37 LINQ to objects
// Select students with average below the class average ordered by average.
var belowAverageStudents =
from Student student in students
orderby student.TestScores.Average()
where student.TestScores.Average() < classAverage
select new {Name = student.FirstName + “ “ + student.LastName,
Average = student.TestScores.Average()};
foreach (var info in belowAverageStudents)
{
belowAverageListBox.Items.Add(info.Name + “ ” + info.Average);
}
This snippet starts by selecting all of the students’ test score averages. This returns a List<double>.
The program calls that list’s
Average function to get the class average.
Next the code queries the student data again, this time selecting students with averages below the
class average.
This query demonstrates a new kind of select clause that creates a list of objects. The new objects
have two properties,
Name and Average, that are given values by the select clause.
The data type of these new objects is created automatically and isn’t given an explicit name so this is
known as an anonymous type.
After creating the query, the code loops through its results, using each object’s
Name and Average
property to display the below average students in a
ListBox. Notice that the code gives the looping
variable
info the implicit data type var so it doesn’t need to figure out what data type it really has.
Objects with anonymous data types actually have a true data type, just not
one that you want to have to figure out. For example, you can add the follow-
ing statement inside the previous code’s
foreach loop to see what data type the
objects actually have:
Console.WriteLine(info.GetType().ToString());
If you look in the Output window, you’ll see that these objects have the ungainly
data type:
<>f__AnonymousType0`2[System.String,System.Double]
Though you can sort of see what’s going on here (note that the object contains
a string and a double), you probably wouldn’t want to type this mess into your
code even if you could. In this case, the
var type is a lot easier to read.
LINQ provides plenty of other features that won’t fit in this lesson. It lets you:
Group results to produce output lists that contain other lists
Take only a certain number of results or take results while a certain condition is true
596906c37.indd 418 4/7/10 12:35:01 PM