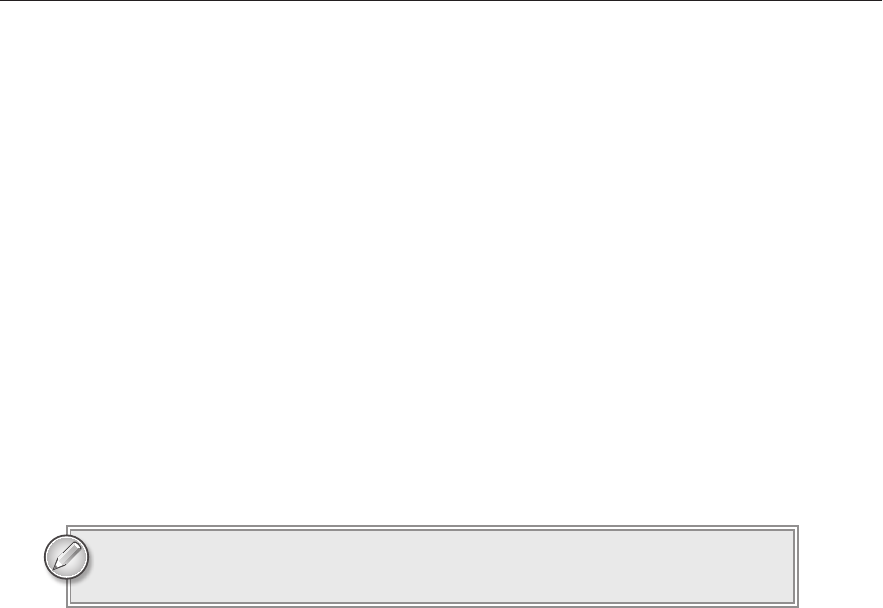
Converting
Casting only works between compatible types. The Convert utility class (which is provided by the
.NET Framework) gives you methods that you can use to try to convert values even if the data types
are incompatible. These are shared methods provided by the
Convert class itself, so you don’t need
to create an instance of the class to use them.
For example, the
bool and int data types are not compatible, so C# doesn’t let you cast from one
to the other. Occasionally, however, you might want to convert an
int into a bool or vice versa.
In that case you can use the
Convert class’s ToBoolean and ToInt32 methods. (You use ToInt32
because
ints are 32-bit integers.)
The following code declares two
int variables and assigns them values. It uses Convert to change
them into
bools and then changes one of them back into an int.
int trueInt = -1;
int falseInt = 0;
bool trueBool = Convert.ToBoolean(trueInt);
bool falseBool = Convert.ToBoolean(falseInt);
int anotherTrueInt = Convert.ToInt32(trueBool);
When you treat integer values as a Booleans, the value 0 is false and all other
values are true. If you convert
true into an integer value, you get –1.
In a particularly common scenario, a program must convert text entered by the user into some other
data type such as an
int or decimal. The following uses the Convert.ToInt32 method to convert
whatever the user entered in the
ageTextBox into an int:
int age = Convert.ToInt32(ageTextBox.Text);
This conversion works only if the user enters a value that can be reasonably converted into an int. If
the user enters 13, 914, or –1, the conversion works. If the user enters “seven,” the conversion fails.
Converting text into another data type is more properly an example of parsing than of data type
conversion, however. So while the
Convert methods work, your code will be easier to read and
understand if you use the parsing methods described in the next section.
Parsing
Trying to find structure and meaning in text is called parsing. All of the simple data types (int,
double, decimal) provide a method that converts text into that data type. For example, the int
data type’s
Parse method takes a string as a parameter and returns an int; at least it does if the
string contains an integer value.
The following code declares a
decimal variable named salary, uses the decimal’s Parse method to
convert the value in the
salaryTextBox into a decimal, and saves the result in the variable:
decimal salary = decimal.Parse(salaryTextBox.Text);
596906c11.indd 135 4/7/10 12:32:33 PM