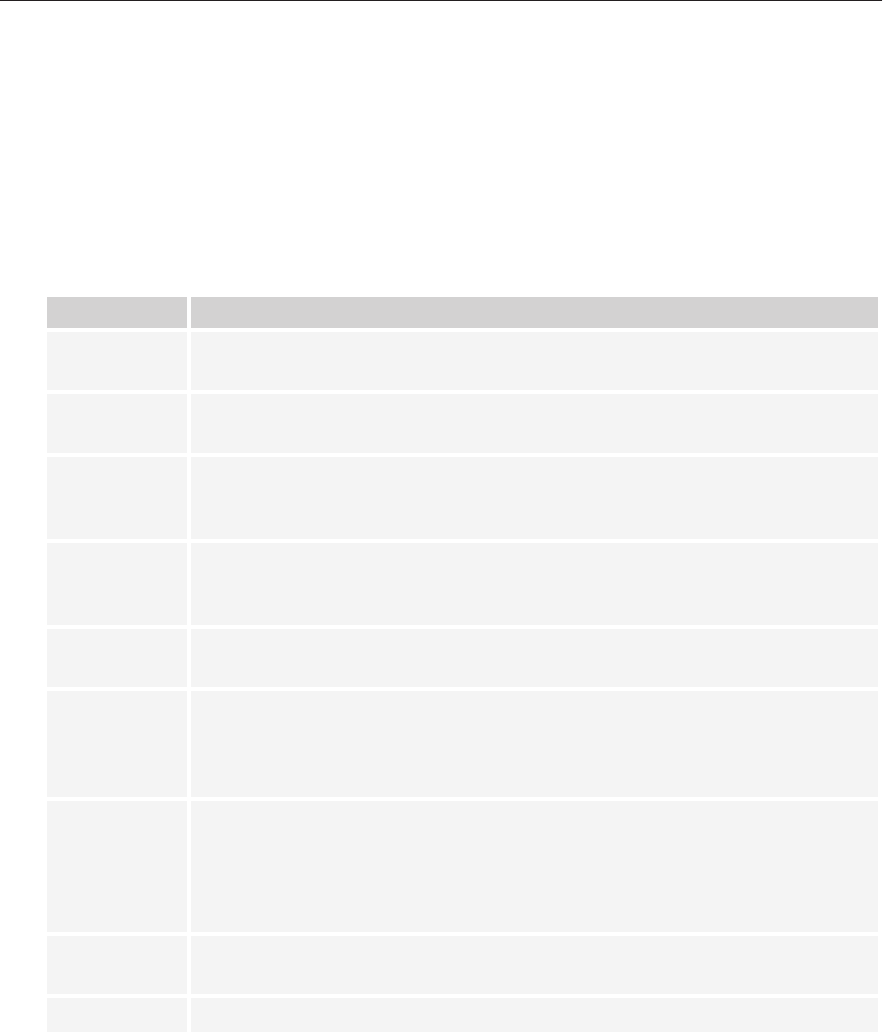
180
LESSON 14 Working With StringS
For the C, E, F, N, and P formats, the precision specifier indicates the number of digits after the
decimal point.
Custom Numeric Formats
If the standard numeric formatting characters don’t do what you want, you can use a custom
numeric format. Table 14-3 summarizes the custom numeric formatting characters.
TABLE 143
CHARACTER MEANING
0 Digit or zero. A digit is displayed here or a zero if there is no corresponding digit in
the value being formatted.
# Digit or nothing. A digit is displayed here or nothing if there is no corresponding
digit in the value being formatted.
. Decimal separator. The decimal separator goes here. Note that the actual separa-
tor character may not be a period depending on the computer’s regional settings,
although you still use the period in the format string.
, Thousands separator. The thousands separator goes here. The actual separator
character may not be a comma depending on the computer’s regional settings,
although you still use the comma in the format string.
% Percent. The number is multiplied by 100 and the percent sign is added at this point.
For example, %0 puts the percent sign before the number and 0% puts it after.
E+0 Scientific notation. The number of 0s indicates the number of digits in the exponent.
If + is included, the exponent always includes a + or – sign. If + is omitted, the expo-
nent only includes a sign if the exponent is negative. For example, the format string
#.##E+000 used with the value 1234.56 produces the result 1.23E+003.
Escape character. Whatever follows the is displayed without any conversion. For
example, the format 0.00\% would add a percent sign to a number without scaling it
by 100 as the format 0.00% does. Note that you must escape the escape character
itself in a normal (non-verbatim) string. For example, a format string might look like
{0:0.00\%} in the code.
‘ABC’ Literal string. Characters enclosed in single or double quotes are displayed without
any conversion.
; Section separator. See the following text.
You can use a section separator to divide a formatting string into two or three sections. If you use
two sections, the first applies to values greater than or equal to zero, and the second section applies
to values less than zero. If you use three sections, they apply to values that are greater than, less
than, and equal to zero, respectively.
596906c14.indd 180 4/7/10 12:32:54 PM