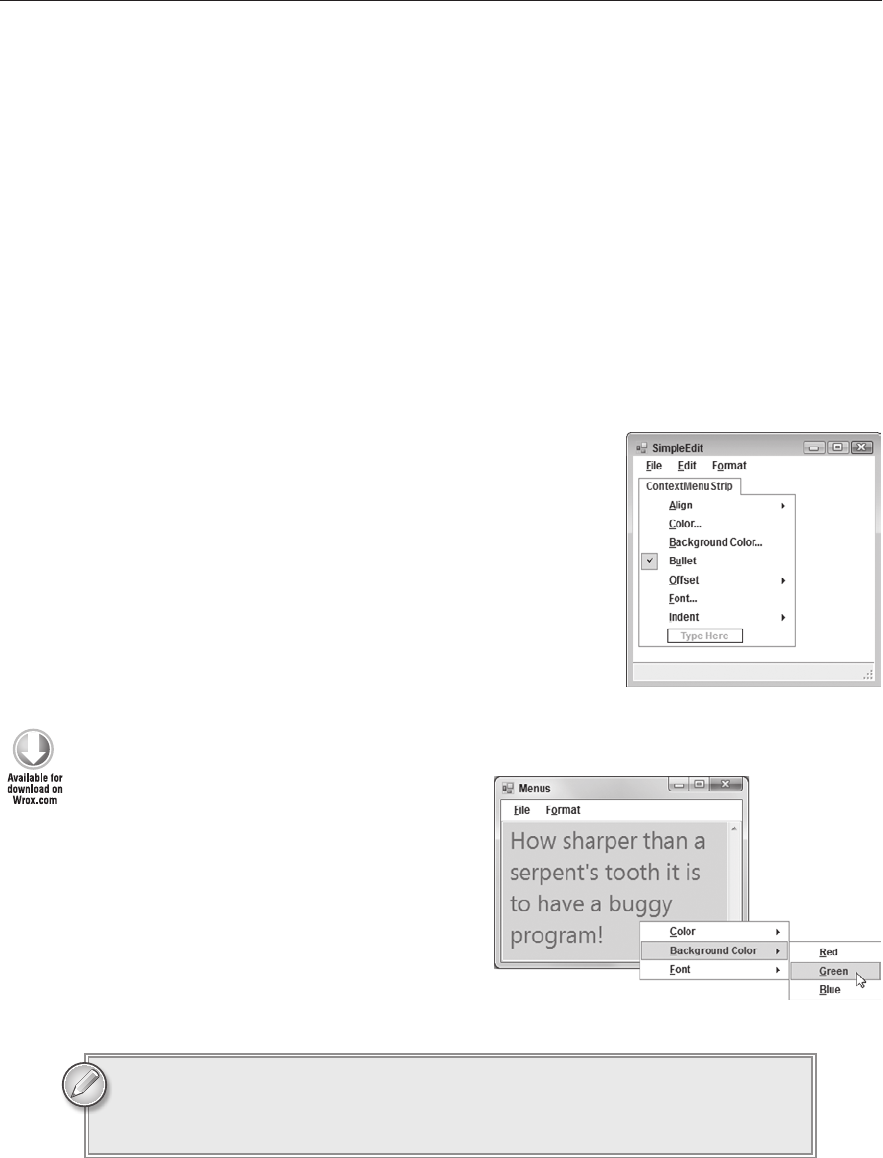
60
❘
LESSON 5 Making Menus
CREATING CONTEXT MENUS
A context menu appears when you right-click a particular control. In Visual C# building a context
menu is almost as easy as building a form’s main menu.
Start by dropping a
ContextMenuStrip on the form. Like a MenuStrip, a ContextMenuStrip
appears below the form in the Component Tray so you can just double-click the Toolbox’s
ContextMenuStrip tool and not worry about positioning the menu.
Unlike a
MenuStrip, a ContextMenuStrip does not appear at the top of the form. In the Form
Designer, you can click a
MenuStrip either on the form or in the Component Tray to select it. To
select a
ContextMenuStrip, you must click it in the Component Tray. (Immediately after you add a
ContextMenuStrip to a form, it is selected so you can see it on the form.)
After you select the
ContextMenuStrip, you can edit it much as you can a MenuStrip. The big dif-
ference is that a
ContextMenuStrip does not have top-level menus, just submenu items.
Figure 5-5 shows the Form Designer with a
ContextMenuStrip
selected. By now the menu editor should look familiar.
After you create a
ContextMenuStrip, you need to associate it
with the control that should display it. To do that, simply set the
control’s
ContextMenuStrip property to the ContextMenuStrip
from the dropdown list. To do that, select the control’s
ContextMenuStrip property in the Properties window, click the
dropdown arrow on the right, and select the
ContextMenuStrip.
The rest is automatic. When the user right-clicks the control, it
automatically displays the
ContextMenuStrip.
TRY IT
In this Try It, you create a main menu and a
context menu. The main menu includes an
Exit command that closes the form. Both
menus contain commands that let you change
the appearance of a
TextBox on the form.
Figure 5-6 shows the finished program dis-
playing its context menu.
You can download the code and resources for this Try It from the book’s web
page at
www.wrox.com or www.CSharpHelper.com/24hour.html. You can find
them in the Lesson05 folder in the download.
FIGURE 55
FIGURE 56
596906c05.indd 60 4/7/10 12:31:52 PM