Earlier in this chapter, we had a brief idea about sensors. Now we will see how to interface these sensors with the Intel Edison. Let us consider the use of the temperature sensor. As already mentioned, most of the sensors have a three or four-pin configuration:
- Vcc
- Ground
- Signal
If you have a look at the Edison board, the board will have analog pins. Ideally, if the sensor returns an analog value, then it goes in the analog pin. It is similar for digital output: we prefer the use of digital pins. Let us look at the following example, where we will interface a temperature sensor. Normally, a typical temperature sensor has three pins. The configuration is the same as previously. However, sometimes due to board compatibility issues, it may come with a four-pin configuration, but in that case one of the pins is not used.
In this example, we are going to use a Grove temperature sensor module:
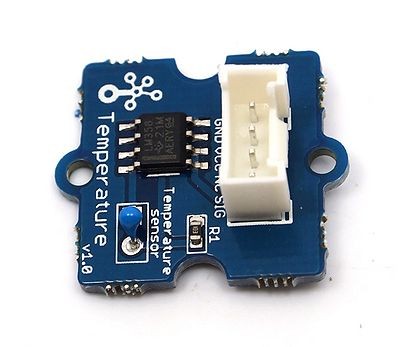
The preceding image is a temperature sensor. You may notice that it has four-pins, designated as Vcc, Gnd, Sig, and NC. In order to connect with your Edison, follow the following circuit diagram:
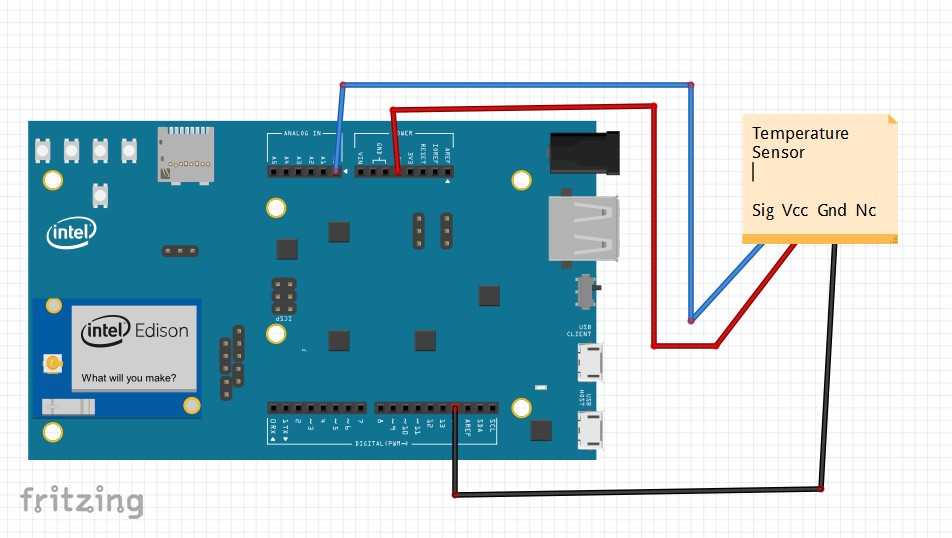
In this circuit diagram, it is noticed that the NC pin is not connected. Thus, only three-pins, Vcc, Gnd, and Sig, are connected. Now, once you are done with the connections, we need to write some algorithms for reading the data. The standard procedure is to search for the datasheet of the sensor. Normally, we also get mathematical equations for the sensor to obtain the desired parameters.
For the Grove temperature sensor module, our first target is to obtain some data from the manufacturer's website. Normally, these sensors calculate temperature based on the change in resistance:
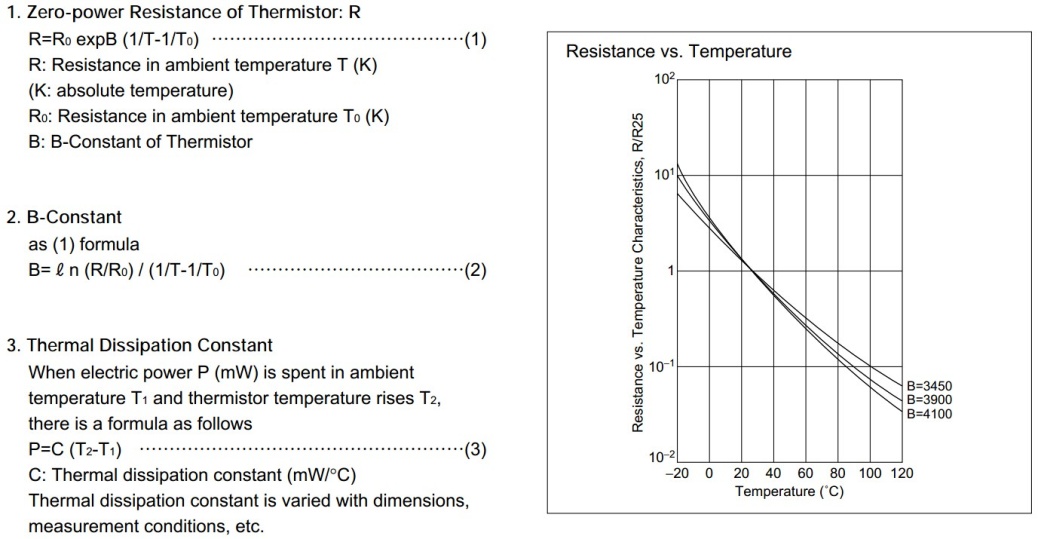
The final formulae will be as follows:
B=4275
R0=100000
R= 1023.0/a-1.0 R= 100000*R
Final Temperature = 1.0/(log(R/100000.0)/B+1/298.15)-273.15
The preceding deduction was obtained from http://wiki.seeed.cc/Grove-Temperature_Sensor_V1.2/.
When converting the preceding deduction into code for the Arduino IDE to deploy into the Edison, the result will be as follows:
#include <math.h>
constint B=4275; constint R0 = 100000; constint tempPin = A0; void setup()
{
Serial.begin(9600);
}
void loop()
{
int a = analogRead(tempPin ); float R = 1023.0/((float)a)-1.0;
R = 100000.0*R;
float temperature=1.0/(log(R/100000.0)/B+1/298.15)-273.15;
Serial.print("temperature = "); Serial.println(temperature);
delay(500);
}