In this chapter, you will create the example project used throughout this part of the book. The project contains a data model that is displayed using simple controllers and Razor Pages.
Creating the Project
Open a new PowerShell command prompt from the Windows Start menu and run the commands shown in Listing 32-1.
You can download the example project for this chapter—and for all the other chapters in this book—from https://github.com/apress/pro-asp.net-core-3. See Chapter 1 for how to get help if you have problems running the examples.
Creating the Project
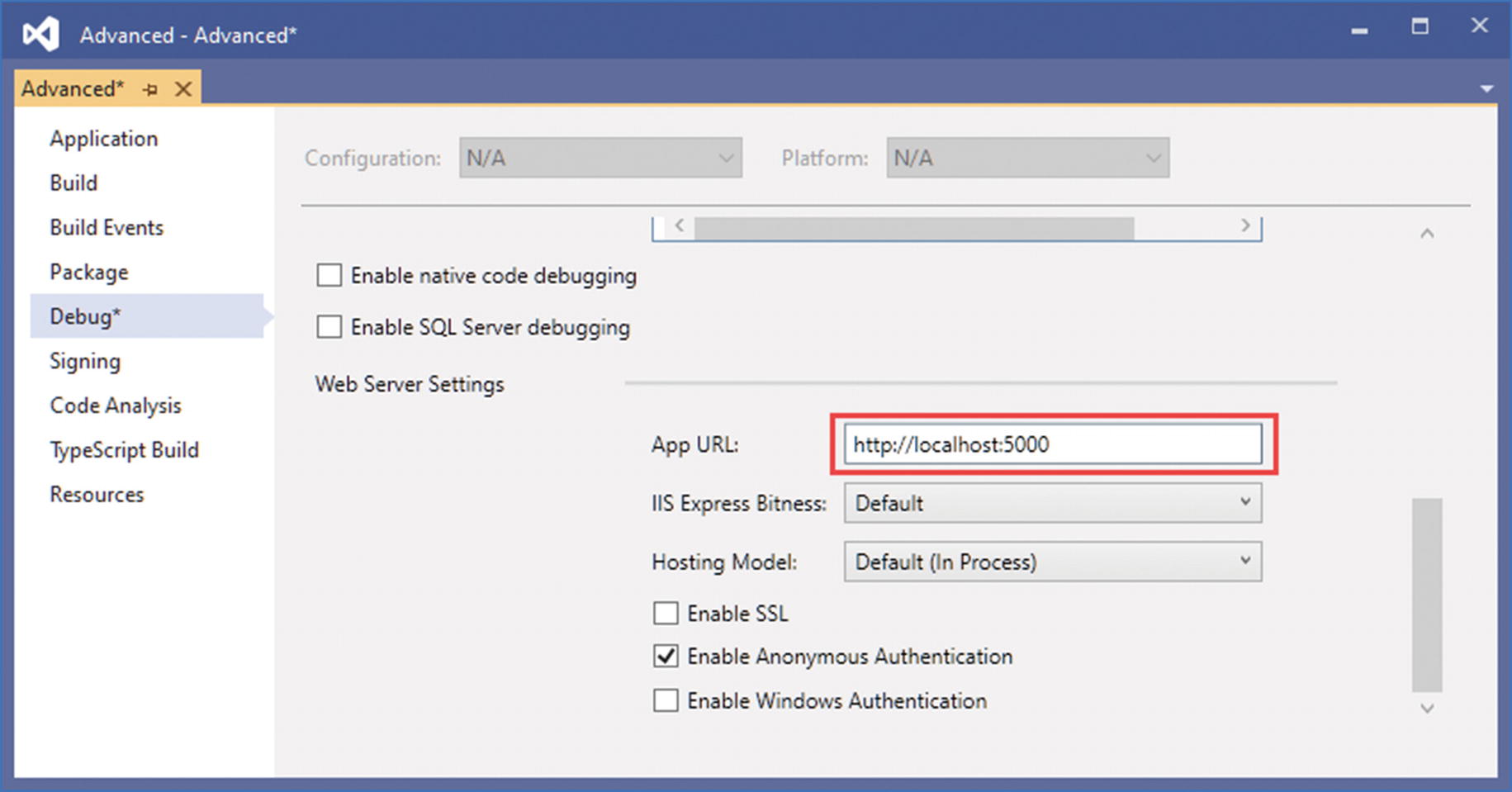
Changing the HTTP port
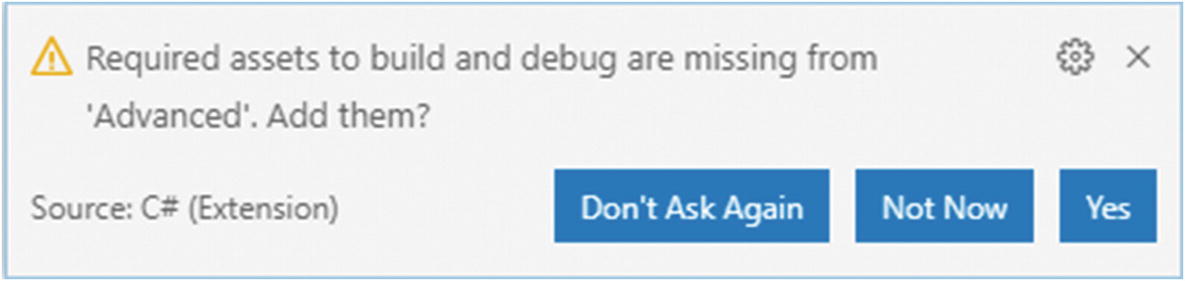
Adding project assets
Adding NuGet Packages to the Project
Adding Packages to the Project
If you are using Visual Studio, you can add the packages by selecting Project ➤ Manage NuGet Packages. Take care to choose the correct version of the packages to add to the project.
Installing a Global Tool Package
Adding a Data Model
The Contents of the Person.cs File in the Models Folder
The Contents of the Department.cs File in the Models Folder
The Contents of the Location.cs File in the Models Folder
Each of the three data model classes defines a key property whose value will be allocated by the database when new objects are stored and defines foreign key properties that define the relationships between the classes. These are supplemented by navigation properties that will be used with the Entity Framework Core Include method to incorporate related data into queries.
The Contents of the DataContext.cs File in the Models Folder
The context class defines properties that will be used to query the database for Person, Department, and Location data.
Preparing the Seed Data
The Contents of the SeedData.cs File in the Models Folder
The static SeedDatabase method ensures that all pending migrations have been applied to the database. If the database is empty, it is seeded with data. Entity Framework Core will take care of mapping the objects into the tables in the database, and the key properties will be assigned automatically when the data is stored.
Configuring Entity Framework Core Services and Middleware
Preparing Services and Middleware in the Startup.cs File in the Advanced Folder
Defining a Connection String in the appsettings.json File in the Advanced Folder
In addition to the connection string, Listing 32-10 increases the logging detail for Entity Framework Core so that the SQL queries sent to the database are logged.
Creating and Applying the Migration
Creating an Entity Framework Core Migration
Applying the Migration to the Database
The logging messages displayed by the application will show the SQL commands that are sent to the database.
If you need to reset the database, then run the dotnet ef database drop --force command and then the command in Listing 32-12.
Adding the Bootstrap CSS Framework
Installing the Bootstrap CSS Framework
If you are using Visual Studio, you can install client-side packages by right-clicking the Advanced project item in the Solution Explorer and selecting Add ➤ Client-Side Library from the popup menu.
Configuring the Services and Middleware
Adding a Package to the Example Project
Adding Services and Middleware in the Startup.cs File in the Advanced Folder
In addition to the default controller route, I have added a route that matches URL paths that begin with controllers, which will make it easier to follow the examples in later chapters as they switch between controllers and Razor Pages. This is the same convention I adopted in earlier chapters, and I will route URL paths beginning with /pages to Razor Pages.
Creating a Controller and View
The Contents of the HomeController.cs File in the Controllers Folder
The Contents of the Index.cshtml File in the Views/Home Folder
The Contents of the _ViewImports.cshtml File in the Views Folder
The Contents of the _ViewStart.cshtml File in the Views Folder
The Contents of the _Layout.cshtml File in the Views/Shared Folder
Creating a Razor Page
The Contents of the Index.cshtml File in the Pages Folder
The Contents of the _ViewImports.cshtml File in the Pages Folder
The Contents of the _ViewStart.cshtml File in the Pages Folder
The Contents of the _Layout.cshtml File in the Pages Folder
Running the Example Application
Running the Example Application
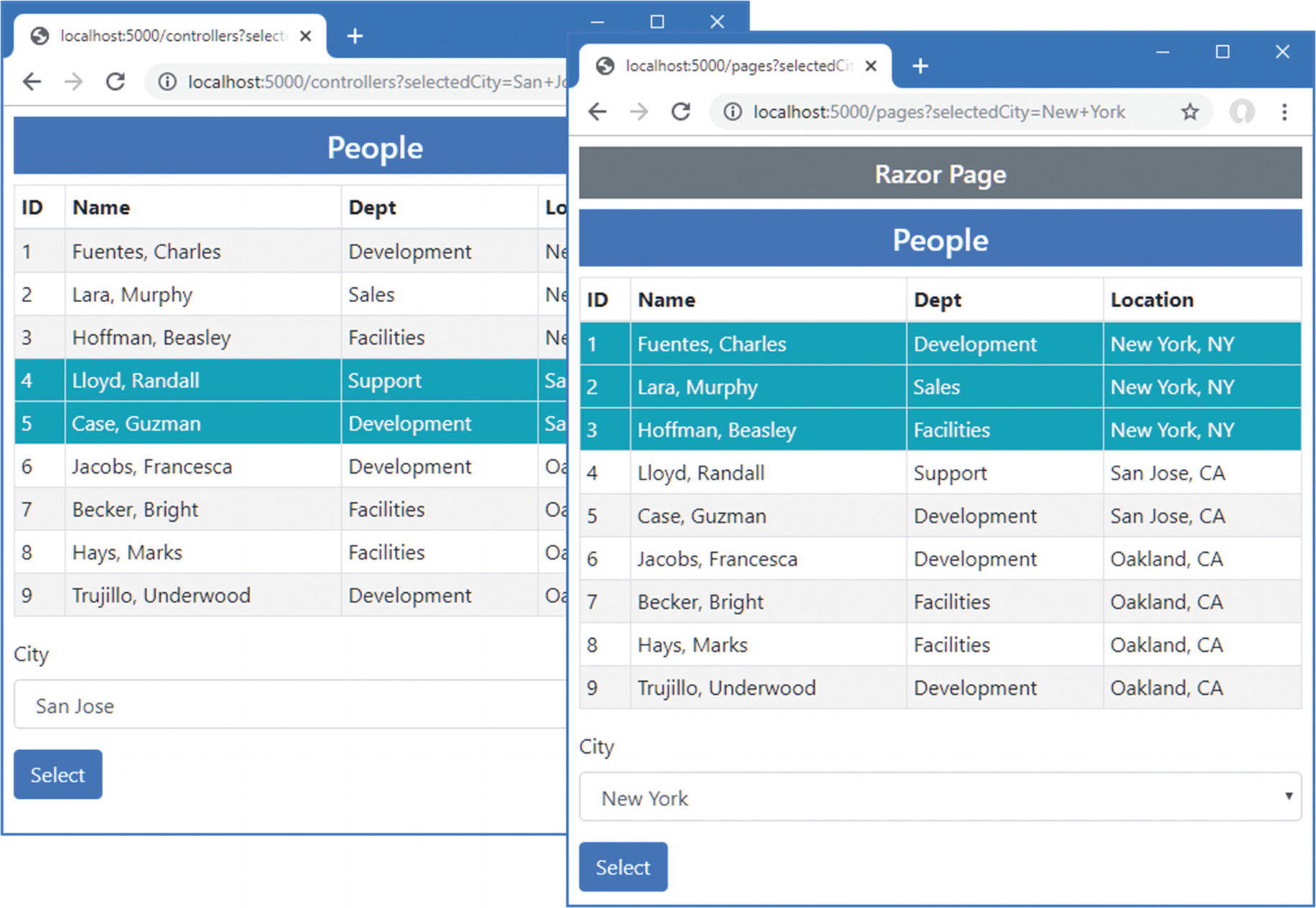
Running the example application
Summary
In this chapter, I showed how to create the example application that is used throughout this part of the book. The project was created with the Empty template, and it contains a data model that relies on Entity Framework Core and handles requests using a controller and a Razor Page. In the next chapter, I introduce Blazor, which is a new addition to ASP.NET Core.