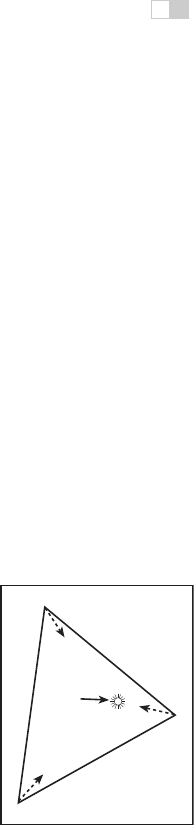
i
i
i
i
i
i
i
i
18.3. Processing Geometry into Pixels 453
room for the vertex array containing the vertices of the triangle mesh. Next, the
vertex array and index array are copied over to the graphics hardware. When it
is time to render the geometry, the vertex buffer object identifier can be used to
instruct the graphics hardware to draw your geometry. If you are already using
vertex arrays in your application, modifying your code to use VBOs should likely
require a minimal change.
18.3 Processing Geometry into Pixels
After the geometry has been placed in the graphics hardware memory, each ver-
tex must be lit as well as transformed into screen coordinates during the geometry
processing stage. In the fixed-functiongraphics pipeline illustrated in Figure 18.1,
vertices are transformed from a model coordinate system to a screen coordinate
frame of reference. This process and the matrices involved are described in Chap-
ters 7 and 8. The modelview and projection matrices needed for this transfor-
mation are defined using functions provided with the graphics API you decide to
use.
Lighting is calculated on a per-vertex basis. Depending on the global shading
parameters, the triangle face will either have a flat-shaded look or the face color
will be diffusely shaded (Gouraud shading) by linearly interpolating the color at
each triangle vertex across the face of the triangle. The latter method produces
a much smoother appearance. The color at each vertex is computed based on
the assigned material properties, the lights in the scene, and various lighting
parameters.
The lighting model in the fixed-function graphics pipeline is good for fast
lighting of vertices; we make a tradeoff for increased speed over accurate illu-
mination. As a result, Phong shaded surfaces are not supported with this fixed-
function framework.
Figure 18.4. The distance
to the light source is small
relative to the size of the tri-
angle.
In particular, the diffuse shading algorithm built into the graphics hardware
often fails to compute the appropriate illumination since the lighting is only being
calculated at each vertex. For example, when the distance to the light source is
small, as compared with the size of the face being shaded, the illumination on
the face will be incorrect. Figure 18.4 illustrates this situation. The center of
the triangle will not be illuminated brightly despite being very close to the light
source, since the lighting on the vertices, which are far from the light source, are
used to interpolate the shading across the face.
With the fixed-function pipeline, this issue can only be remedied by increasing
the tessellation of the geometry. This solution works but is of limited use in real-