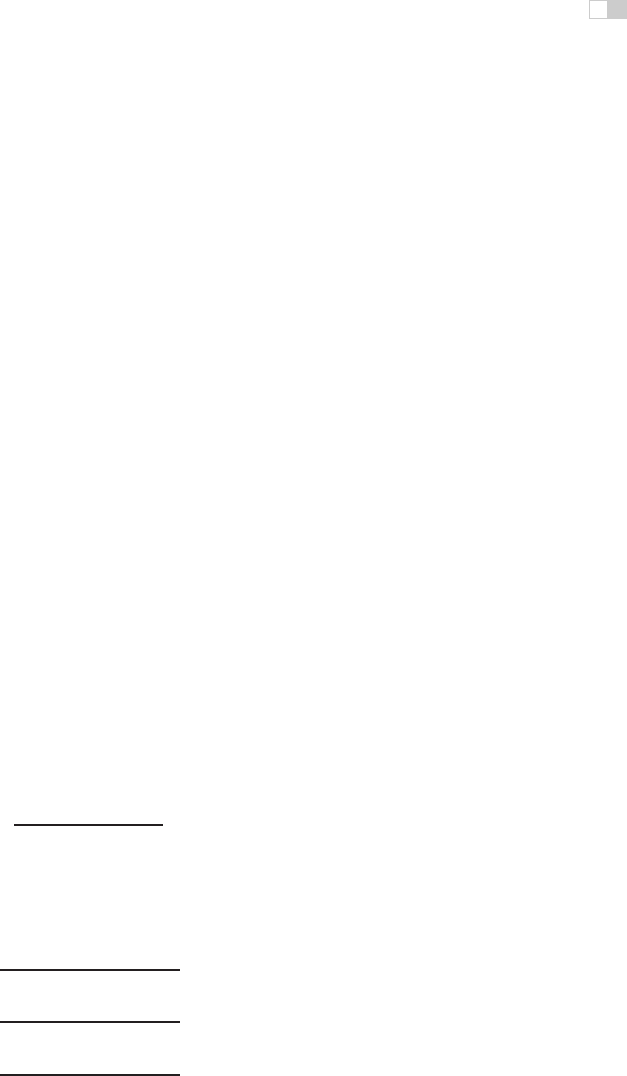
i
i
i
i
i
i
i
i
7.2. Projective Transformations 149
where y is the distance of the point along the y-axis, and y
s
is where the point
should be drawn on the screen.
We would really like to use the matrix machinery we developed for ortho-
graphic projection to draw perspective images; we could then just multiply an-
other matrix into our composite matrix and use the algorithm we already have.
However, this type of transformation, in which one of the coordinates of the input
vector appears in the denominator, can’t be achieved using affine transformations.
We can allow for division with a simple generalization of the mechanism of
homogeneous coordinates that we have been using for affine transformations.
We have agreed to represent the point (x, y, z) using the homogeneous vector
[xyz1]
T
; the extra coordinate, w,isalwaysequalto1, and this is ensured by
always using [0001]
T
as the fourth row of an affine transformation matrix.
Rather than just thinking of the 1 as an extra piece bolted on to coerce matrix
multiplication to implement translation, we now define it to be the denominator
of the x-, y-, and z-coordinates: the homogeneous vector [xyzw]
T
represents
the point (x/w, y/w, z/w). This makes no difference when w =1, but it allows a
broader range of transformations to be implemented if we allow any values in the
bottom row of a transformation matrix, causing w to take on values other than 1.
Concretely, linear transformations allow us to compute expressions like
x
= ax + by + cz
and affine transformations extend this to
x
= ax + by + cz + d.
Treating w as the denominator further expands the possibilities, allowing us to
compute functions like
x
=
ax + by + cz + d
ex + fy + gz + h
;
this could be called a “linear rational function” of x, y,andz. But there is an extra
constraint—the denominators are the same for all coordinates of the transformed
point:
x
=
a
1
x + b
1
y + c
1
z + d
1
ex + fy + gz + h
,
y
=
a
2
x + b
2
y + c
2
z + d
2
ex + fy + gz + h
,
z
=
a
3
x + b
3
y + c
3
z + d
3
ex + fy + gz + h
.