Next, we will create a new file with the name sendGoals.cpp in the chapter7_tutorials/src folder and add the following code there:
#include <ros/ros.h>
#include <move_base_msgs/MoveBaseAction.h>
#include <actionlib/client/simple_action_client.h>
#include <tf/transform_broadcaster.h>
#include <sstream>
typedef actionlib::SimpleActionClient<move_base_msgs::MoveBaseAction> MoveBaseClient;
int main(int argc, char** argv){
ros::init(argc, argv, "navigation_goals");
MoveBaseClient ac("move_base", true);
while(!ac.waitForServer(ros::Duration(5.0))){
ROS_INFO("Waiting for the move_base action server");
}
move_base_msgs::MoveBaseGoal goal;
goal.target_pose.header.frame_id = "map";
goal.target_pose.header.stamp = ros::Time::now();
goal.target_pose.pose.position.x = 1.0;
goal.target_pose.pose.position.y = 1.0;
goal.target_pose.pose.orientation.w = 1.0;
ROS_INFO("Sending goal");
ac.sendGoal(goal);
ac.waitForResult();
if(ac.getState() == actionlib::SimpleClientGoalState::SUCCEEDED)
ROS_INFO("You have arrived to the goal position");
else{
ROS_INFO("The base failed for some reason");
}
return 0;
}
The preceding code sample is a basic example for sending a goal to the robot.
Then, we will compile the package and launch the navigation stack to test the new program. We will need to run the following commands in two separate terminals to launch all the nodes and the configurations:
$ roslaunch chapter7_tutorials chapter7_configuration_gazebo.launch
$ roslaunch chapter7_tutorials move_base.launch
After configuring the 2D pose estimate, we will need to run the sendGoal node with the following command in a new terminal:
$ rosrun chapter6_tutorials sendGoals
In the RViz screen, we can observe a new global plan over the map, as shown in the following screenshot:
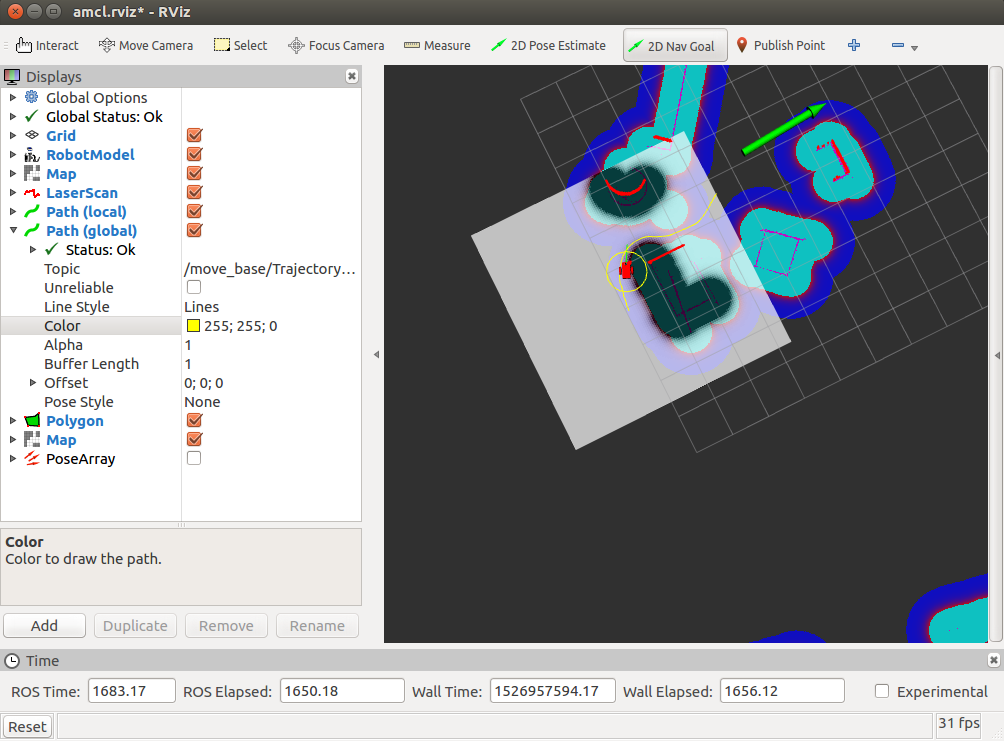
This confirms that the navigation stack has accepted the new goal command. When the robot arrives at the goal, we will see the "You have arrived to the goal position" message in the shell where sendGoals was running. In this way, we can make a list of goals or waypoints, and create a route for the robot and plan a mission.