Sometimes, instead of just moving the end-effector towards a goal, we may be interested in setting a goal for a specific joint. Let's see how we can do this.
First of all, we'll need to launch the MoveIt RViz environment by using the following command:
$ roslaunch fetch_moveit_config fetch_planning_execution.launch
And, execute the following Python code:
#! /usr/bin/env python import sys import copy import rospy import moveit_commander import moveit_msgs.msg import geometry_msgs.msg moveit_commander.roscpp_initialize(sys.argv) rospy.init_node('move_group_python_interface_tutorial', anonymous=True) robot = moveit_commander.RobotCommander() scene = moveit_commander.PlanningSceneInterface() group = moveit_commander.MoveGroupCommander("arm") display_trajectory_publisher = rospy.Publisher('/move_group/display_planned_path', moveit_msgs.msg.DisplayTrajectory) group_variable_values = group.get_current_joint_values() group_variable_values[5] = -1.5 group.set_joint_value_target(group_variable_values) plan2 = group.plan() rospy.sleep(5) moveit_commander.roscpp_shutdown()
When the code finishes executing, we'll see how the robot is planning the specified motion described in the preceding code:
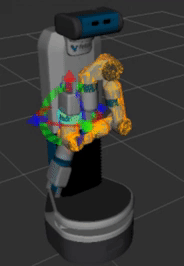
That's great, right? But, as we did previously, let's analyze the new code we've introduced in order to understand what's going on:
group.clear_pose_targets()
Here, we are just clearing the actual values of the pose_target variable:
group_variable_values = group.get_current_joint_values()
Next, we are getting the current values of the joints:
group_variable_values[3] = 1.5 group.set_joint_value_target(group_variable_values)
Now, we will modify the value of one of the joints and set this new joint value as a target:
plan2 = group.plan()
Finally, we just compute the plan for the new joint space goal.
Inside the my_motion_scripts package, we will create a new file named joint_planning.py. Similarly, as we did previously, we can perform some tests by giving different values to different joints.